Library designed as an interface to the mbed Pin Connect Block
Embed:
(wiki syntax)
Show/hide line numbers
PinConnectBlock.h
00001 //mbed Microcontroller Library 00002 //Library designed as an interface to the mbed Pin Connect Block 00003 //Copyright 2011 00004 //Thomas Hamilton 00005 00006 #ifndef LPC1768PinConnectBlockLibrary 00007 #define LPC1768PinConnectBlockLibrary 00008 00009 #include "cmsis.h" 00010 00011 #ifdef __cplusplus 00012 extern "C" 00013 { 00014 #endif 00015 00016 namespace PinConnectBlock 00017 { 00018 unsigned char ValidateInput( 00019 unsigned char Port, unsigned char Pin, unsigned char Mode); 00020 //This function accepts a Port number, Pin number, and Mode code for 00021 //a pin that is to be connected to microcontroller hardware. It 00022 //validates the input for future use as input to connection setting 00023 //functions. It will check for the validity of the Port and Pin and 00024 //the validity of the Function, Resistor, and Open Drain mode 00025 //selections for a valid Port and Pin. The Mode codes are specified 00026 //as follows: 00027 // 00028 //Bits 7:5 - Reserved ------------------------------------------ 00029 //Bit 4 - OpenDrain Mode | See documentation for the mapping of | 00030 //Bits 3:2 - Resistor Mode | mode code values to connections. The | 00031 //Bits 1:0 - Function Mode | reserved bits must be 0. | 00032 // ------------------------------------------ 00033 //The return value is a code with flags to alert the user of errors in 00034 //the input. The errors are specified as follows: 00035 // 00036 //Bits 7:6 - Reserved 00037 //Bit 5 - OpenDrain Mode 00038 //Bit 4 - Resistor Mode ------------------------------------------ 00039 //Bit 3 - Function Mode | A value of 0 indicates there are no | 00040 //Bit 2 - Mode | errors. A value of 1 indicates an | 00041 //Bit 1 - Pin | error in the input corresponding with | 00042 //Bit 0 - Port | that bit. Reserved bits will be 0. | 00043 // ------------------------------------------ 00044 void SetPinConnection( 00045 unsigned char Port, unsigned char Pin, unsigned char Mode); 00046 //This function accepts a Port number, Pin number, and Mode code and 00047 //connects the selected Pin on the selected Port to the hardware 00048 //specified in the Mode code. This function will not check for the 00049 //validity of the input and will write to a reserved or unrelated 00050 //sector if an invalid Port and Pin are specified. It will reject any 00051 //portion of the Mode code that connects 1.0:1.17 to functions 10 or 00052 //11, 0.27:0.30 to resistor hardware, or 0.27:0.28 to open drain mode 00053 //hardware, and will complete any valid connections in the Mode code. 00054 //The Mode codes are specified as follows: 00055 // 00056 //Bits 7:5 - Reserved ------------------------------------------ 00057 //Bit 4 - OpenDrain Mode | See documentation for the mapping of | 00058 //Bits 3:2 - Resistor Mode | mode code values to connections. The | 00059 //Bits 1:0 - Function Mode | reserved bits must be 0. | 00060 // ------------------------------------------ 00061 unsigned char GetPinConnection( 00062 unsigned char Port, unsigned char Pin); 00063 //This function accepts a Port number and Pin number and returns a 00064 //mode code that specifies the hardware connected to the selected Pin 00065 //on the selected Port. This function will not check for the validity 00066 //of the input and will return junk bits from a reserved or unrelated 00067 //sector if an invalid Port and Pin are specified. The Mode codes are 00068 //specified as follows: 00069 // 00070 //Bits 7:5 - Reserved ------------------------------------------ 00071 //Bit 4 - OpenDrain Mode | See documentation for the mapping of | 00072 //Bits 3:2 - Resistor Mode | mode code values to connections. The | 00073 //Bits 1:0 - Function Mode | reserved bits must be 0. | 00074 // ------------------------------------------ 00075 void SetFunctionMode( 00076 unsigned char Port, unsigned char Pin, unsigned char Mode); 00077 //This function accepts a Port number, Pin number, and Mode code and 00078 //connects the selected Pin on the selected Port to the function 00079 //hardware specified in the Mode code. This function will not check 00080 //for the validity of the input and will write to a reserved or 00081 //non-function mode sector if an invalid Port and Pin are specified. 00082 //Mode codes are specified as follows: 00083 // ------------------------------------------ 00084 // | See documentation for the mapping of | 00085 //Bits 7:2 - Reserved | mode code values to connections. The | 00086 //Bits 1:0 - Function Mode | reserved bits and modes must be 0. | 00087 // ------------------------------------------ 00088 unsigned char GetFunctionMode(unsigned char Port, unsigned char Pin); 00089 //This function accepts a Port number and Pin number and returns a 00090 //mode code that specifies the function hardware connected to the 00091 //selected Pin on the selected Port. This function will not check for 00092 //the validity of the input and will return junk bits from a reserved 00093 //or non-function mode sector if an invalid Port and Pin are 00094 //specified. The Mode codes are specified as follows: 00095 // ------------------------------------------ 00096 // | See documentation for the mapping of | 00097 //Bits 2:7 - Reserved | mode code values to connections. The | 00098 //Bits 1:0 - Function Mode | reserved bits will be 0. | 00099 // ------------------------------------------ 00100 void SetResistorMode( 00101 unsigned char Port, unsigned char Pin, unsigned char Mode); 00102 //This function accepts a Port number, Pin number, and Mode code and 00103 //connects the selected Pin on the selected Port to the resistor 00104 //hardware specified in the Mode code. This function will not check 00105 //for the validity of the input and will write to a reserved or 00106 //non-resistor mode sector if an invalid Port and Pin are specified. 00107 //The Mode codes are ------------------------------------------- 00108 //specified as follows: | Value - Connection | 00109 // |-----------------------------------------| 00110 // | 00 - Pull Up Resistor | 00111 // | 01 - Repeater Mode | 00112 //Bits 7:2 - Reserved | 10 - No Resistor | 00113 //Bits 1:0 - Resistor Mode | 11 - Pull Down Resistor | 00114 // ------------------------------------------- 00115 unsigned char GetResistorMode(unsigned char Port, unsigned char Pin); 00116 //This function accepts a Port number and Pin number and returns a 00117 //mode code that specifies the resistor hardware connected to the 00118 //selected Pin on the selected Port. This function will not check for 00119 //the validity of the input and will return junk bits from a reserved 00120 //or non-resistor mode sector if an invalid Port and Pin are 00121 //specified. The Mode ------------------------------------------- 00122 //codes are specified as | Value - Connection | 00123 //follows: |-----------------------------------------| 00124 // | 00 - Pull Up Resistor | 00125 // | 01 - Repeater Mode | 00126 //Bits 7:2 - Reserved | 10 - No Resistor | 00127 //Bits 1:0 - Resistor Mode | 11 - Pull Down Resistor | 00128 // ------------------------------------------- 00129 void SetOpenDrainMode( 00130 unsigned char Port, unsigned char Pin, bool Mode); 00131 //This function accepts a Port number, Pin number, and Mode bit and 00132 //connects or disconnects the open drain hardware for the selected Pin 00133 //on the selected Port. A 0 will disconnect the open drain hardware, 00134 //and a 1 will connect the open drain hardware. This function will 00135 //not check for the validity of the input before making a connection, 00136 //and will write to a reserved or non-open drain mode sector if an 00137 //invalid Port and Pin are specified. 00138 bool GetOpenDrainMode(unsigned char Port, unsigned char Pin); 00139 //This function accepts a Port number and Pin number and returns a 00140 //mode bit that specifies the resistor hardware that is connected to 00141 //the selected Pin on the selected Port. A value of 0 indicates that 00142 //the open drain hardware is disconnected, and a value of 1 indicates 00143 //that the open drain hardware is connected. This function will not 00144 //check for the validity of the input and will return a junk bit from 00145 //a reserved or non-open drain sector if an invalid Port and Pin are 00146 //specified. 00147 void SetTraceMode(bool Mode); 00148 //This function accepts a Mode bit and connects or disconnects the 00149 //trace port hardware. A value of 0 will disconnect the trace port 00150 //hardware, and a value of 1 will connect the trace port hardware. 00151 //The trace port uses pins 2.2:2.6, and will override any function, 00152 //resistor, or open drain hardware connected to these pins. 00153 bool GetTraceMode(); 00154 //This function returns a mode bit that specifies the current 00155 //connection status of the trace port. A value of 0 indicates that 00156 //the trace port is disconnected, and a value of 1 indicates that the 00157 //trace port is connected. 00158 void SetI2C0Mode(unsigned char Mode); 00159 //This function accepts a Mode code and connects or disconnects 00160 //specialized I2C hardware to the I2C0 bus on pins 0.27:0.28. The 00161 //Mode codes are specified as follows: 00162 // ----------------------------------------------- 00163 //Bit 1: I2C Fast Mode | A value of 0 will disconnect the hard- | 00164 //Bit 0: I2C Filter | ware, and a value of 1 will connect it. | 00165 // ----------------------------------------------- 00166 //For non-I2C of these pins, the Filter and Fast Mode hardware should 00167 //be disconnected. For normal I2C use of these pins, the Filter 00168 //hardware should be connected and the Fast Mode hardware should be 00169 //disconnected. For I2C Fast Mode, the Filter hardware and Fast Mode 00170 //hardware should both be connected. 00171 unsigned char GetI2C0Mode(); 00172 //This function returns a mode code that specifies the current 00173 //connection status of the I2C0 bus with its specialized I2C hardware. 00174 //The first two bits indicate the current hardware connection, and 00175 //the next four bits indicate errors in the connection. The 00176 //connection status of SCL pin 0.28 must mach that of SDA pin 0.27. 00177 //If this is not the case, the bus will not function properly and the 00178 //function will return an error code. The I2C Mode codes are 00179 //specified as follows: ---------------------------- 00180 // | A value of o indicates | 00181 //Bit 5: Error - Only SCL Fast Mode is On | the hardware is discon- | 00182 //Bit 4: Error - Only SDA Fast Mode is On | nected or the error is | 00183 //Bit 3: Error - Only SCL Filter is On | not present. A value of | 00184 //Bit 2: Error - Only SDA Filter is On | 1 indicates the hardware | 00185 //Bit 1: I2C Fast Mode | is connected or the | 00186 //Bit 0: I2C Filter | error is present. | 00187 // ---------------------------- 00188 } 00189 00190 #ifdef __cplusplus 00191 } 00192 #endif 00193 00194 #endif
Generated on Thu Aug 18 2022 12:58:48 by
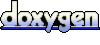