Rewrite from scratch a TCP/IP stack for mbed. So far the following parts are usable: Drivers: - EMAC driver (from CMSIS 2.0) Protocols: - Ethernet protocol - ARP over ethernet for IPv4 - IPv4 over Ethernet - ICMPv4 over IPv4 - UDPv4 over IPv4 APIs: - Sockets for UDPv4 The structure of this stack is designed to be very modular. Each protocol can register one or more protocol to handle its payload, and in each protocol, an API can be hooked (like Sockets for example). This is an early release.
mbedNet.h
00001 /* 00002 * $Id: mbedNet.h 29 2011-06-11 14:53:08Z benoit $ 00003 * $Author: benoit $ 00004 * $Date: 2011-06-11 16:53:08 +0200 (sam., 11 juin 2011) $ 00005 * $Rev: 29 $ 00006 * 00007 * 00008 * 00009 * 00010 * 00011 */ 00012 00013 #ifndef __MBEDNET_H__ 00014 #define __MBEDNET_H__ 00015 00016 00017 #include <stdint.h> 00018 00019 00020 enum Bool 00021 { 00022 False = 0, 00023 True = !False, 00024 }; 00025 typedef enum Bool Bool_t; 00026 00027 00028 #define DEBUG_ON 1 00029 00030 #define NET_DEFAULT_TTL 128 /* Default TTL */ 00031 #define NET_ENCAPSULATION_MAX_DEPTH 5 /* Maximum protocol encapsulation depth */ 00032 #define NET_PERIODIC_FUNCTION_MAX_COUNT 5 /* Maximum number of timers */ 00033 #define NETIF_MAX_COUNT 2 /* Maximum number of registrable interfaces */ 00034 #define ETHERNET_PROTOCOL_MAX_COUNT 3 /* Maximum number of registrable protocols over ethernet */ 00035 #define IPV4_PROTOCOL_MAX_COUNT 4 /* Maximum number of registrable protocols over IPv4 */ 00036 #define ARP_CACHE_MAX_ENTRIES 4 /* Maximum number of entries in the ARP cache */ 00037 #define ARP_FUNCTION_PERIOD 10 /* Period in seconds of the ARP periodic function */ 00038 #define ARP_MAX_ENTRY_AGE 60 /* Max age of a dynamic entry in seconds */ 00039 #define NET_API_PER_PROTOCOL_MAX_COUNT 3 /* Maximum number of API per protocol */ 00040 #define QUEUE_MAX_ENTRY_COUNT 16 /* Maximum entries per queue */ 00041 #define SOCKET_MAX_COUNT 10 /* Maximum number of sockets in the system */ 00042 #define SOCKET_DATAQUEUE_ENTRY_COUNT 4 /* Maximum data block entries per socket */ 00043 #define MBEDNET_HAVE_RTOS 0 /* 1 = have RTOS, 0 = no RTOS */ 00044 00045 00046 #define ntohs(a) (((((uint16_t)a) >> 8) & 0x00FF) | ((((uint16_t)a) << 8) & 0xFF00)) 00047 #define htons(a) ntohs(a) 00048 00049 00050 enum mbedNetResult 00051 { 00052 mbedNetResult_Success = 0, 00053 mbedNetResult_NotEnoughMemory, 00054 mbedNetResult_TooManyInterfaces, 00055 mbedNetResult_InvalidInterface, 00056 mbedNetResult_InterfaceAlreadyUp, 00057 mbedNetResult_InterfaceAlreadyDown, 00058 mbedNetResult_TooManyPeriodicFunctions, 00059 mbedNetResult_TooManyDrivers, 00060 mbedNetResult_InvalidDriver, 00061 mbedNetResult_InvalidParameter, 00062 mbedNetResult_TooManyRegisteredProtocols, 00063 mbedNetResult_NoProtocolRegistrationFunction, 00064 mbedNetResult_NoAPIRegistrationFunction, 00065 mbedNetResult_TooManyOpenSockets, 00066 mbedNetResult_InvalidSocketHandle, 00067 mbedNetResult_SocketAlreadyClosed, 00068 mbedNetResult_WouldBlock, 00069 mbedNetResult_QueueEmpty, 00070 mbedNetResult_QueueFull, 00071 mbedNetResult_NotIplemented, 00072 mbedNetResult_BufferTooSmall, 00073 mbedNetResult_NoRouteToHost, 00074 mbedNetResult_WaitARPResolution, 00075 mbedNetResult_DestinationAddressRequired, 00076 mbedNetResult_TooMuchData, 00077 mbedNetResult_Count, 00078 }; 00079 typedef enum mbedNetResult mbedNetResult_t; 00080 00081 00082 #define MA0 address[0] 00083 #define MA1 address[1] 00084 #define MA2 address[2] 00085 #define MA3 address[3] 00086 #define MA4 address[4] 00087 #define MA5 address[5] 00088 struct Ethernet_Addr 00089 { 00090 uint8_t address[6]; 00091 }; 00092 typedef struct Ethernet_Addr Ethernet_Addr_t; 00093 00094 extern const Ethernet_Addr_t ethernet_Addr_Broadcast; 00095 extern const Ethernet_Addr_t ethernet_Addr_Null; 00096 00097 00098 #define IP0 bytes.ip0 00099 #define IP1 bytes.ip1 00100 #define IP2 bytes.ip2 00101 #define IP3 bytes.ip3 00102 00103 #pragma push 00104 #pragma pack(1) 00105 union IPv4_Addr 00106 { 00107 struct { 00108 uint32_t ip0:8; 00109 uint32_t ip1:8; 00110 uint32_t ip2:8; 00111 uint32_t ip3:8; 00112 } bytes; 00113 uint32_t addr; 00114 }; 00115 #pragma pop 00116 00117 typedef union IPv4_Addr IPv4_Addr_t; 00118 00119 extern const IPv4_Addr_t ipv4_Addr_Broadcast; 00120 extern const IPv4_Addr_t ipv4_Addr_Any; 00121 #define IPADDR_ANY ipv4_Addr_Any.addr 00122 #define IPADDR_BROADCAST ipv4_Addr_Broadcast.addr 00123 00124 00125 #if MBEDNET_HAVE_RTOS 00126 00127 #error "Please define mutex and semaphore macros" 00128 00129 #else /* MBEDNET_HAVE_RTOS */ 00130 00131 typedef int32_t RTOS_Mutex_t; 00132 00133 #define RTOS_MUTEX_CREATE() 0 00134 #define RTOS_MUTEX_LOCK(id) id++ 00135 #define RTOS_MUTEX_UNLOCK(id) id-- 00136 00137 typedef int32_t RTOS_Sem_t; 00138 00139 #define RTOS_SEM_CREATE(i, m) 0 00140 #define RTOS_SEM_P(id) id++ 00141 #define RTOS_SEM_V(id) id-- 00142 00143 #endif /* MBEDNET_HAVE_RTOS */ 00144 00145 extern mbedNetResult_t mbedNet_LastError; 00146 00147 00148 #endif /* __MBEDNET_H__ */
Generated on Wed Jul 13 2022 06:09:33 by
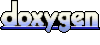