Rewrite from scratch a TCP/IP stack for mbed. So far the following parts are usable: Drivers: - EMAC driver (from CMSIS 2.0) Protocols: - Ethernet protocol - ARP over ethernet for IPv4 - IPv4 over Ethernet - ICMPv4 over IPv4 - UDPv4 over IPv4 APIs: - Sockets for UDPv4 The structure of this stack is designed to be very modular. Each protocol can register one or more protocol to handle its payload, and in each protocol, an API can be hooked (like Sockets for example). This is an early release.
Sockets.h
00001 /* 00002 * $Id: Sockets.h 27 2011-06-09 12:55:57Z benoit $ 00003 * $Author: benoit $ 00004 * $Date: 2011-06-09 14:55:57 +0200 (jeu., 09 juin 2011) $ 00005 * $Rev: 27 $ 00006 * 00007 * 00008 * 00009 * 00010 * 00011 */ 00012 00013 #ifndef __SOCKETS_H__ 00014 #define __SOCKETS_H__ 00015 00016 00017 #include <stdint.h> 00018 #include "NetIF.h" 00019 00020 00021 typedef int32_t Socket_t; 00022 00023 enum Socket_Family 00024 { 00025 AF_INET = 0, 00026 AF_INET6, 00027 Socket_Family_Count, 00028 }; 00029 typedef enum Socket_Family Socket_Family_t; 00030 00031 00032 enum Socket_Protocol 00033 { 00034 SOCK_DGRAM = 0, 00035 SOCK_STREAM, 00036 SOCK_RAW, 00037 Socket_Protocol_Count, 00038 }; 00039 typedef enum Socket_Protocol Socket_Protocol_t; 00040 00041 00042 #pragma push 00043 #pragma pack(1) 00044 struct Socket_Addr 00045 { 00046 Socket_Family_t family; 00047 uint16_t len; 00048 }; 00049 #pragma pop 00050 typedef struct Socket_Addr Socket_Addr_t; 00051 00052 00053 #pragma push 00054 #pragma pack(1) 00055 struct Socket_AddrIn 00056 { 00057 Socket_Family_t family; 00058 uint16_t len; 00059 IPv4_Addr_t address; 00060 uint16_t port; 00061 }; 00062 #pragma pop 00063 typedef struct Socket_AddrIn Socket_AddrIn_t; 00064 00065 00066 extern Net_API_t sockets; 00067 00068 00069 Socket_t Sockets_Open(Socket_Family_t family, Socket_Protocol_t protocol, int32_t options); 00070 int32_t Sockets_Bind(Socket_t socket, Socket_Addr_t *addr, int32_t addrLen); 00071 int32_t Sockets_Send(Socket_t socket, uint8_t *data, int32_t length, int32_t flags); 00072 int32_t Sockets_SendTo(Socket_t socket, uint8_t *data, int32_t length, int32_t flags, const Socket_Addr_t *remoteAddr, int32_t addrLen); 00073 int32_t Sockets_Recv(Socket_t socket, uint8_t *data, int32_t length, int32_t flags); 00074 int32_t Sockets_RecvFrom(Socket_t socket, uint8_t *data, int32_t length, int32_t flags, Socket_Addr_t *remoteAddr, int32_t *addrLen); 00075 int32_t Sockets_Close(Socket_t socket); 00076 00077 00078 #endif /* __SOCKET_H__ */
Generated on Wed Jul 13 2022 06:09:33 by
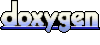