Rewrite from scratch a TCP/IP stack for mbed. So far the following parts are usable: Drivers: - EMAC driver (from CMSIS 2.0) Protocols: - Ethernet protocol - ARP over ethernet for IPv4 - IPv4 over Ethernet - ICMPv4 over IPv4 - UDPv4 over IPv4 APIs: - Sockets for UDPv4 The structure of this stack is designed to be very modular. Each protocol can register one or more protocol to handle its payload, and in each protocol, an API can be hooked (like Sockets for example). This is an early release.
IPv4.h
00001 /* 00002 * $Id: IPv4.h 27 2011-06-09 12:55:57Z benoit $ 00003 * $Author: benoit $ 00004 * $Date: 2011-06-09 14:55:57 +0200 (jeu., 09 juin 2011) $ 00005 * $Rev: 27 $ 00006 * 00007 * 00008 * 00009 * 00010 * 00011 */ 00012 00013 #ifndef __IPV4_H__ 00014 #define __IPV4_H__ 00015 00016 #include "NetIF.h" 00017 00018 00019 #define IPV4_PROTO_ICMPV4 1 00020 #define IPV4_PROTO_TCPV4 6 00021 #define IPV4_PROTO_UDPV4 17 00022 00023 00024 #define IPV4_VERSION 4 00025 #define IPV4_FLAG_DF 0x02 00026 #define IPV4_FLAG_MF 0x04 00027 00028 typedef uint16_t IPv4_Proto_t; 00029 00030 00031 #pragma push 00032 #pragma pack(1) 00033 struct IPv4_Header 00034 { 00035 uint8_t ihl :4; 00036 uint8_t version :4; 00037 uint8_t tos; 00038 uint16_t totalLength; 00039 uint16_t id; 00040 uint16_t fragmentFlags; 00041 uint8_t ttl; 00042 uint8_t protocol; 00043 uint16_t crc; 00044 IPv4_Addr_t source, 00045 dest; 00046 }; 00047 #pragma pop 00048 typedef struct IPv4_Header IPv4_Header_t; 00049 00050 00051 extern Protocol_Handler_t ipv4; 00052 00053 00054 void IPv4_DumpIPv4Header(const char *prefix, IPv4_Header_t *ipv4Packet); 00055 uint16_t IPv4_ComputeCRC(IPv4_Header_t *ipv4Header); 00056 00057 00058 #endif /* __IPV4_H__ */ 00059
Generated on Wed Jul 13 2022 06:09:33 by
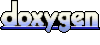