Rewrite from scratch a TCP/IP stack for mbed. So far the following parts are usable: Drivers: - EMAC driver (from CMSIS 2.0) Protocols: - Ethernet protocol - ARP over ethernet for IPv4 - IPv4 over Ethernet - ICMPv4 over IPv4 - UDPv4 over IPv4 APIs: - Sockets for UDPv4 The structure of this stack is designed to be very modular. Each protocol can register one or more protocol to handle its payload, and in each protocol, an API can be hooked (like Sockets for example). This is an early release.
Ethernet.cpp
00001 /* 00002 * $Id: Ethernet.c 29 2011-06-11 14:53:08Z benoit $ 00003 * $Author: benoit $ 00004 * $Date: 2011-06-11 16:53:08 +0200 (sam., 11 juin 2011) $ 00005 * $Rev: 29 $ 00006 * 00007 * 00008 * 00009 * 00010 * 00011 */ 00012 00013 #include "Ethernet.h" 00014 #include "Debug.h" 00015 #include <string.h> 00016 00017 00018 #define DEBUG_CURRENT_MODULE_NAME "Ethernet" 00019 #define DEBUG_CURRENT_MODULE_ID DEBUG_MODULE_ETHERNET 00020 00021 00022 static void Init(void); 00023 static int32_t RegisterProtocol(Protocol_Handler_t *protocolHandler); 00024 static int32_t RegisterAPI(Net_API_t *api); 00025 static void Handler(NetIF_t *netIF, NetPacket_t *packet); 00026 00027 00028 static Protocol_Handler_t *protocolHandlerTable[ETHERNET_PROTOCOL_MAX_COUNT]; 00029 static int32_t protocolHandlerCount = 0; 00030 00031 const Ethernet_Addr_t ethernet_Addr_Broadcast = {0xff, 0xff, 0xff, 0xff, 0xff, 0xff}; 00032 const Ethernet_Addr_t ethernet_Addr_Null = {0, 0, 0, 0, 0, 0}; 00033 00034 00035 Protocol_Handler_t ethernet = 00036 { 00037 PROTOCOL_INDEX_NOT_INITIALIZED, /* Always PROTOCOL_INDEX_NOT_INITIALIZED at initialization */ 00038 Protocol_ID_Ethernet, /* Protocol ID */ 00039 PROTOCOL_NUMBER_NONE, /* Protocol number */ 00040 Init, /* Protocol initialisation function */ 00041 Handler, /* Protocol handler */ 00042 RegisterProtocol, /* Protocol registration function */ 00043 RegisterAPI, /* API registration function */ 00044 }; 00045 00046 00047 static Net_API_t *netAPITable[NET_API_PER_PROTOCOL_MAX_COUNT]; 00048 static int32_t netAPICount = 0; 00049 00050 00051 static void Init(void) 00052 { 00053 DEBUG_MODULE(DEBUG_LEVEL_INFO, ("Initializing ethernet layer")); 00054 memset(protocolHandlerTable, 0, sizeof(protocolHandlerTable)); 00055 protocolHandlerCount = 0; 00056 memset(netAPITable, 0, sizeof(netAPITable)); 00057 netAPICount = 0; 00058 } 00059 00060 00061 static int32_t RegisterProtocol(Protocol_Handler_t *protocolHandler) 00062 { 00063 int32_t result = 0; 00064 00065 if (protocolHandlerCount >= ETHERNET_PROTOCOL_MAX_COUNT) 00066 { 00067 DEBUG_SOURCE(DEBUG_LEVEL_ERROR, ("Too many protocols")); 00068 result = -1; 00069 mbedNet_LastError = mbedNetResult_TooManyRegisteredProtocols; 00070 goto Exit; 00071 } 00072 00073 protocolHandlerTable[protocolHandlerCount] = protocolHandler; 00074 protocolHandler->index = protocolHandlerCount; 00075 protocolHandler->Init(); 00076 00077 DEBUG_MODULE(DEBUG_LEVEL_INFO, ("Registered protocol %04X ethernet/%s", 00078 ntohs(protocolHandler->protocolNumber), 00079 protocol_IDNames[protocolHandler->protocolID] 00080 )); 00081 00082 protocolHandlerCount++; 00083 00084 Exit: 00085 return result; 00086 } 00087 00088 00089 static int32_t RegisterAPI(Net_API_t *netAPI) 00090 { 00091 DEBUG_MODULE(DEBUG_LEVEL_INFO, ("Registering %s API", api_IDNames[netAPI->apiID])); 00092 netAPI->InitAPI(); 00093 netAPITable[netAPICount] = netAPI; 00094 netAPICount++; 00095 return -1; 00096 } 00097 00098 00099 void Handler(NetIF_t *netIF, NetPacket_t *packet) 00100 { 00101 int32_t protocolIndex, index; 00102 Ethernet_Proto_t protocolNumber; 00103 Protocol_Handler_t *protocolHandler; 00104 Ethernet_Header_t *ethernetHeader; 00105 00106 ethernetHeader = (Ethernet_Header_t *)packet->data; 00107 protocolNumber = ethernetHeader->protocol; 00108 DEBUG_MODULE(DEBUG_LEVEL_INFO, ("frame of %d bytes for protocol %04X (payload + %02d)", packet->length, ntohs(protocolNumber), sizeof(Ethernet_Header_t))); 00109 //Debug_DumpBufferHex(packet, length); 00110 00111 /* Process API if any */ 00112 for (index = 0; index < netAPICount; index++) 00113 { 00114 netAPITable[index]->Hook(netIF, Protocol_ID_Ethernet, packet); 00115 } 00116 00117 for (protocolIndex = 0; protocolIndex < protocolHandlerCount; protocolIndex++) 00118 { 00119 protocolHandler = protocolHandlerTable[protocolIndex]; 00120 if (protocolHandler->protocolNumber == protocolNumber) 00121 { 00122 DEBUG_SOURCE(DEBUG_LEVEL_VERBOSE0, ("'%s' frame of %d bytes", protocol_IDNames[protocolHandler->protocolID], packet->length)); 00123 NetIF_ProtoPush(packet, sizeof(Ethernet_Header_t), Protocol_ID_Ethernet); 00124 protocolHandler->HandlePacket(netIF, packet); 00125 break; 00126 } 00127 } 00128 return; 00129 } 00130 00131
Generated on Wed Jul 13 2022 06:09:33 by
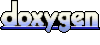