
Troubleshooting
Embed:
(wiki syntax)
Show/hide line numbers
LCD.cpp
00001 #include "LCD.hpp" 00002 #include "DataTypes.hpp" 00003 00004 //LCD data sheet: https://www.rapidonline.com/pdf/57-2224.pdf 00005 00006 void LCD::INIT() 00007 { 00008 //All lines default low 00009 _LCD_RS = 0; 00010 _LCD_E = 0; 00011 00012 //LCD Initialise 00013 wait_ms(45); //Wait for LCD startup 00014 /*Step 1*/ 00015 wait_us(40); //Wait whilst LCD busy 00016 _LCD_RS = control; 00017 LCD_DDRAM = 0; //Clear data line 00018 LCD_DDRAM = (FUNC|bit8)>>4; //Put data on line 00019 LCD_strobe(); 00020 00021 /*Step 2*/ cmdLCD(FUNC|lines2); //Function Set 0x20|0x08 = 0x28 00022 /*Step 3*/ cmdLCD(FUNC|lines2); //Function Set 0x20|0x08 = 0x28 00023 /*Step 4*/ cmdLCD(DISPLAY|on); //Display Control 0x08|0x0x04 = 0x0c 00024 /*Step 5*/ cmdLCD(CLEAR); //Clear Display 0x01 00025 /*Step 6*/ cmdLCD(ENTRYMODE|I); //Set entry mode 0x04|0x02 = 0x06 00026 00027 cmdLCD(RETURN); //return home location 00028 } 00029 00030 void LCD::clear() 00031 { 00032 cmdLCD(CLEAR); 00033 } 00034 00035 void LCD::display(BYTE* str, UINT_16 location) 00036 { 00037 if(location != NULL) 00038 { 00039 pos(location); 00040 } 00041 U_BYTE p = 0; 00042 while((str[p]!= NULL)&&(p<16)) 00043 { 00044 putt(str[p]); 00045 p++; 00046 } 00047 } 00048 00049 void LCD::pos(UINT_16 location) 00050 { 00051 cmdLCD(0x80|location); 00052 } 00053 00054 void LCD::putt(U_BYTE c) 00055 { 00056 wait_us(3000); 00057 _LCD_RS = text; 00058 set_LCD_data(c); 00059 } 00060 00061 void LCD::cmdLCD(U_BYTE cmd) 00062 { 00063 wait_us(3000); //Wait whilst LCD busy 00064 _LCD_RS = control; 00065 set_LCD_data(cmd); //set data on bus 00066 } 00067 00068 void LCD::LCD_strobe(void) 00069 { 00070 wait_us(10); 00071 _LCD_E = 1; 00072 wait_us(10); 00073 _LCD_E = 0; 00074 } 00075 00076 void LCD::set_LCD_data(U_BYTE d) 00077 { 00078 // Send upper 4 bits then lower for bits 00079 // e.g. 11110000 => 1111 -> 0000 00080 00081 LCD_DDRAM = 0; //Clear data line 00082 LCD_DDRAM = d>>4; //Put data on line 00083 LCD_strobe(); 00084 wait_us(1000); 00085 LCD_DDRAM = 0; //Clear 00086 LCD_DDRAM = d; //Put remaining data on line 00087 LCD_strobe(); 00088 } 00089 00090 void LCD::enableCursor() 00091 { 00092 cmdLCD(DISPLAY|on|cursor); 00093 } 00094 00095 void LCD::disableCursor() 00096 { 00097 cmdLCD(DISPLAY|on); 00098 }
Generated on Fri Jul 15 2022 05:30:30 by
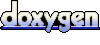