
Calculates azimuth and elevation of a satellite dish based on its longitude, latitude and selected satellite.
Dependencies: PinDetect TextLCD mbed MODGPS
SatelliteList.cpp
00001 #include "SatelliteList.h" 00002 #include <iostream> 00003 00004 SatelliteList::SatelliteList(char *f, char delimiter){ 00005 00006 string line; 00007 vector<string> v; 00008 00009 sfile.open(f); 00010 if (sfile.is_open()) { 00011 while ( getline(sfile,line) ){ 00012 if( line.empty() ); // ignore empty lines 00013 else if (line.at(0) != ';'){ // ignore comments 00014 split( v, line, ';' ); 00015 //for ( int i = 0; i < v.size(); i++) printf("%s ",v[i].c_str()); 00016 //printf("\r\n"); 00017 if (v.size() == 4) { 00018 Satellite *s = new Satellite(v[0],v[1], ::atof(v[2].c_str()), ::atof(v[3].c_str())); 00019 clist.addNode(*s); 00020 delete s; 00021 } 00022 v.clear(); 00023 } 00024 } 00025 //printf("Size Circular List = %d \r\n", clist.getSize()); 00026 sfile.close(); 00027 } else printf("Can't open filename %s\r\n", f); 00028 } 00029 00030 void SatelliteList::split(vector<string> & theStringVector, /* Altered/returned value */ 00031 const string & theString, 00032 const char theDelimiter) 00033 { 00034 00035 00036 size_t start = 0, end = 0; 00037 00038 while ( end != string::npos) 00039 { 00040 end = theString.find( theDelimiter, start); 00041 00042 00043 theStringVector.push_back( theString.substr( start, 00044 (end == string::npos) ? string::npos : end - start)); 00045 00046 00047 start = ( ( end > (string::npos - 1) ) 00048 ? string::npos : end + 1); 00049 } 00050 } 00051 00052 void SatelliteList::display() 00053 { 00054 string n, c ; 00055 float o,i ; 00056 00057 clist.toHead(); 00058 for ( int j = 0; j < clist.getSize(); j++) { 00059 Satellite *s = clist.getNode(); 00060 n = s->getName(); 00061 c = s->getCname(); 00062 o = s->getOrbit(); 00063 i = s->getInclination(); 00064 printf("Size = %d i = %d Name = %s Cname = %s \r\n",clist.getSize(), j, n.c_str(), c.c_str()); 00065 clist.moveForward(); 00066 } 00067 } 00068 00069 Satellite * SatelliteList::getNext() 00070 { 00071 clist.moveForward(); 00072 Satellite *s = clist.getNode(); 00073 return(s); 00074 } 00075 00076 Satellite * SatelliteList::getPrev() 00077 { 00078 clist.moveBackward(); 00079 Satellite *s = clist.getNode(); 00080 return(s); 00081 } 00082 00083 Satellite * SatelliteList::getCurrent() 00084 { if (!clist.isEmpty()) { 00085 Satellite *s = clist.getNode(); 00086 return(s); 00087 } 00088 }
Generated on Tue Jul 12 2022 21:03:40 by
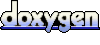