
Based on Peter Barrett\'s work on BlueUSB, I added support for the PS3 Sixaxis controller (both USB and Bluetooth). When connecting a Sixaxis via USB, it will be paired with the (hardcoded) MAC address of my Bluetooth dongle.
Dependents: PS3_BlueUSB_downstate
AutoEvents.cpp
00001 /* 00002 Copyright (c) 2010 Peter Barrett 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 /* 00024 Tue Apr 26 2011 Bart Janssens: added PS3 USB support 00025 */ 00026 00027 #include <stdio.h> 00028 #include <stdlib.h> 00029 #include <stdio.h> 00030 #include <string.h> 00031 00032 #include "USBHost.h" 00033 #include "Utils.h" 00034 #include "ps3.h" 00035 00036 #define AUTOEVT(_class,_subclass,_protocol) (((_class) << 16) | ((_subclass) << 8) | _protocol) 00037 #define AUTO_KEYBOARD AUTOEVT(CLASS_HID,1,1) 00038 #define AUTO_MOUSE AUTOEVT(CLASS_HID,1,2) 00039 //#define AUTO_PS3 AUTOEVT(CLASS_HID,0,0) 00040 00041 u8 auto_mouse[4]; // buttons,dx,dy,scroll 00042 u8 auto_keyboard[8]; // modifiers,reserved,keycode1..keycode6 00043 u8 auto_joystick[4]; // x,y,buttons,throttle 00044 //u8 auto_ps3[48]; 00045 00046 00047 00048 00049 void AutoEventCallback(int device, int endpoint, int status, u8* data, int len, void* userData) 00050 { 00051 int evt = (int)userData; 00052 switch (evt) 00053 { 00054 case AUTO_KEYBOARD: 00055 printf("AUTO_KEYBOARD "); 00056 break; 00057 case AUTO_MOUSE: 00058 printf("AUTO_MOUSE "); 00059 break; 00060 // case AUTO_PS3: 00061 // printf("AUTO_PS3 "); 00062 // ParsePs3Report(data,len); 00063 // break; 00064 default: 00065 printf("HUH "); 00066 } 00067 //printfBytes("data",data,len); 00068 USBInterruptTransfer(device,endpoint,data,len,AutoEventCallback,userData); 00069 } 00070 00071 // Establish transfers for interrupt events 00072 void AddAutoEvent(int device, InterfaceDescriptor* id, EndpointDescriptor* ed) 00073 { 00074 printf("message from endpoint %02X\r\n",ed->bEndpointAddress); 00075 printf("Class Sub Proto: %02X %02X %02X\r\n",id->bInterfaceClass,id->bInterfaceSubClass,id->bInterfaceProtocol); 00076 //if ((ed->bmAttributes & 3) != ENDPOINT_INTERRUPT || !(ed->bEndpointAddress & 0x80)) 00077 // return; 00078 00079 // Make automatic interrupt enpoints for known devices 00080 u32 evt = AUTOEVT(id->bInterfaceClass,id->bInterfaceSubClass,id->bInterfaceProtocol); 00081 printf("Evt: %08X \r\n",evt); 00082 u8* dst = 0; 00083 int len; 00084 switch (evt) 00085 { 00086 case AUTO_MOUSE: 00087 dst = auto_mouse; 00088 len = sizeof(auto_mouse); 00089 break; 00090 case AUTO_KEYBOARD: 00091 dst = auto_keyboard; 00092 len = sizeof(auto_keyboard); 00093 break; 00094 // case AUTO_PS3: 00095 // printf("PS3 event ? \r\n"); 00096 // dst = auto_ps3; 00097 // len = sizeof(auto_ps3); 00098 default: 00099 printf("Interrupt endpoint %02X %08X\r\n",ed->bEndpointAddress,evt); 00100 break; 00101 } 00102 if (dst) 00103 { 00104 printf("Auto Event for %02X %08X\r\n",ed->bEndpointAddress,evt); 00105 USBInterruptTransfer(device,ed->bEndpointAddress,dst,len,AutoEventCallback,(void*)evt); 00106 } 00107 } 00108 00109 void PrintString(int device, int i) 00110 { 00111 u8 buffer[256]; 00112 int le = GetDescriptor(device,DESCRIPTOR_TYPE_STRING,i,buffer,255); 00113 if (le < 0) 00114 return; 00115 char* dst = (char*)buffer; 00116 for (int j = 2; j < le; j += 2) 00117 *dst++ = buffer[j]; 00118 *dst = 0; 00119 printf("%d:%s\r\n",i,(const char*)buffer); 00120 } 00121 00122 // Walk descriptors and create endpoints for a given device 00123 int StartAutoEvent(int device, int configuration, int interfaceNumber) 00124 { 00125 00126 printf("StartAutoEvent \r\n"); 00127 00128 u8 buffer[255]; 00129 int err = GetDescriptor(device,DESCRIPTOR_TYPE_CONFIGURATION,0,buffer,255); 00130 if (err < 0) 00131 return err; 00132 00133 int len = buffer[2] | (buffer[3] << 8); 00134 u8* d = buffer; 00135 u8* end = d + len; 00136 while (d < end) 00137 { 00138 if (d[1] == DESCRIPTOR_TYPE_INTERFACE) 00139 { 00140 InterfaceDescriptor* id = (InterfaceDescriptor*)d; 00141 if (id->bInterfaceNumber == interfaceNumber) 00142 { 00143 d += d[0]; 00144 while (d < end && d[1] != DESCRIPTOR_TYPE_INTERFACE) 00145 { 00146 if (d[1] == DESCRIPTOR_TYPE_ENDPOINT) 00147 AddAutoEvent(device,id,(EndpointDescriptor*)d); 00148 d += d[0]; 00149 } 00150 } 00151 } 00152 d += d[0]; 00153 } 00154 return 0; 00155 } 00156 00157 /* 00158 int StartPS3Event(int device, int configuration, int interfaceNumber) 00159 { 00160 00161 printf("StartPS3Event \r\n"); 00162 00163 EndpointDescriptor* ep; 00164 00165 u8 buf[4]; 00166 buf[0] = 0x42; 00167 buf[1] = 0x0c; 00168 buf[2] = 0x00; 00169 buf[3] = 0x00; 00170 00171 u8 buf2[8]; 00172 u8 buf3[8]; 00173 00174 buf2[0] = 0x01; 00175 buf2[1] = 0x00; 00176 buf2[2] = 0x00; 00177 buf2[3] = 0x02; 00178 buf2[4] = 0x72; 00179 buf2[5] = 0xAD; 00180 buf2[6] = 0xF3; 00181 buf2[7] = 0x5B; 00182 00183 00184 00185 00186 int result; 00187 int err; 00188 00189 u8 buffer[255]; 00190 err = GetDescriptor(device,DESCRIPTOR_TYPE_CONFIGURATION,0,buffer,255); 00191 if (err < 0) 00192 return err; 00193 00194 00195 00196 //configure the device 00197 //err = USBControlTransfer(device, HOST_TO_DEVICE|REQUEST_TYPE_STANDARD|RECIPIENT_DEVICE, SET_CONFIGURATION, 1, 0, 0, 0, 0, 0 ); 00198 err = SetConfiguration(device,1); 00199 printf("set config result = %d\r\n", err); 00200 00201 // get Mac address 00202 //err = USBControlTransfer(device, HOST_TO_DEVICE|REQUEST_TYPE_CLASS|RECIPIENT_DEVICE, HID_REQUEST_GET_REPORT, 0x03f5, 0, buf3, sizeof(buf3), 0, 0 ); 00203 //printf("get Mac to %02X:%02X:%02X:%02X:%02X:%02X , result = %d\r\n", buf3[2], buf3[3], buf3[4], buf3[5], buf3[6], buf3[7], err); 00204 00205 // set Mac address 00206 err = USBControlTransfer(device, HOST_TO_DEVICE|REQUEST_TYPE_CLASS|RECIPIENT_INTERFACE, HID_REQUEST_SET_REPORT, 0x03f5, 0, buf2, sizeof(buf2), 0, 0 ); 00207 printf("set Mac to %02X:%02X:%02X:%02X:%02X:%02X , result = %d\r\n", buf2[2], buf2[3], buf2[4], buf2[5], buf2[6], buf2[7], err); 00208 00209 // get Mac address 00210 //err = USBControlTransfer(device, HOST_TO_DEVICE|REQUEST_TYPE_CLASS|RECIPIENT_DEVICE, HID_REQUEST_GET_REPORT, 0x03f5, 0, buf3, sizeof(buf3), 0, 0 ); 00211 //printf("get Mac to %02X:%02X:%02X:%02X:%02X:%02X , result = %d\r\n", buf3[2], buf3[3], buf3[4], buf3[5], buf3[6], buf3[7], err); 00212 00213 err = USBControlTransfer(device, HOST_TO_DEVICE|REQUEST_TYPE_CLASS|RECIPIENT_INTERFACE, HID_REQUEST_SET_REPORT, 0x03f4,0, buf, sizeof(buf), 0, 0 ); 00214 printf("set report result = %d\r\n", err); 00215 //USBTransfer(device,0,DEVICE_TO_HOST,buf,sizeof(buf),0,0); 00216 00217 int len = buffer[2] | (buffer[3] << 8); 00218 u8* d = buffer; 00219 u8* end = d + len; 00220 while (d < end) 00221 { 00222 if (d[1] == DESCRIPTOR_TYPE_INTERFACE) 00223 { 00224 InterfaceDescriptor* id = (InterfaceDescriptor*)d; 00225 if (id->bInterfaceNumber == interfaceNumber) 00226 { 00227 d += d[0]; 00228 while (d < end && d[1] != DESCRIPTOR_TYPE_INTERFACE) 00229 { 00230 if (d[1] == DESCRIPTOR_TYPE_ENDPOINT) 00231 ep = (EndpointDescriptor*)d; 00232 00233 if (ep->bEndpointAddress == 0x02) { 00234 printf("PS3 input endpoint (0x02) found\r\n"); 00235 00236 } 00237 if (ep->bEndpointAddress == 0x81) { 00238 printf("PS3 output endpoint (0x81) found\r\n"); 00239 AddAutoEvent(device,id,(EndpointDescriptor*)d); 00240 } 00241 d += d[0]; 00242 } 00243 } 00244 } 00245 d += d[0]; 00246 } 00247 return 0; 00248 } 00249 */ 00250 00251 // Implemented in main.cpp 00252 int OnDiskInsert(int device); 00253 00254 // Implemented in TestShell.cpp 00255 int OnBluetoothInsert(int device); 00256 00257 void OnLoadDevice(int device, DeviceDescriptor* deviceDesc, InterfaceDescriptor* interfaceDesc) 00258 { 00259 printf("LoadDevice %d %02X:%02X:%02X\r\n",device,interfaceDesc->bInterfaceClass,interfaceDesc->bInterfaceSubClass,interfaceDesc->bInterfaceProtocol); 00260 char s[128]; 00261 u8 my_mac[6] = {0x00, 0x02, 0x72, 0xAD, 0xF3, 0x5B}; // mac address of my Bluetooth device 00262 00263 u8 buf2[6]; 00264 00265 buf2[0] = 0x00; 00266 buf2[1] = 0x02; 00267 buf2[2] = 0x72; 00268 buf2[3] = 0xAD; 00269 buf2[4] = 0xF3; 00270 buf2[5] = 0x5B; 00271 00272 00273 for (int i = 1; i < 3; i++) 00274 { 00275 if (GetString(device,i,s,sizeof(s)) < 0) 00276 break; 00277 printf("%d: %s\r\n",i,s); 00278 } 00279 00280 switch (interfaceDesc->bInterfaceClass) 00281 { 00282 case CLASS_MASS_STORAGE: 00283 if (interfaceDesc->bInterfaceSubClass == 0x06 && interfaceDesc->bInterfaceProtocol == 0x50) 00284 OnDiskInsert(device); // it's SCSI! 00285 break; 00286 case CLASS_WIRELESS_CONTROLLER: 00287 if (interfaceDesc->bInterfaceSubClass == 0x01 && interfaceDesc->bInterfaceProtocol == 0x01) 00288 OnBluetoothInsert(device); // it's bluetooth! 00289 break; 00290 case CLASS_HID: 00291 printf("idVendor = %04X idProduct = %04X \r\n",deviceDesc->idVendor,deviceDesc->idProduct); 00292 //printf("device = %d configuration = %d interfaceNumber = %d\r\n", device, configuration, interfaceNumber); 00293 //if (deviceDesc->idVendor == 0x054C && deviceDesc->idProduct == 0x0268) StartPS3Event(device,1,0); 00294 if (deviceDesc->idVendor == 0x054C && deviceDesc->idProduct == 0x0268) { 00295 Ps3USB _Ps3USB(device,1,0); 00296 00297 _Ps3USB.SetPair(my_mac); 00298 _Ps3USB.Enable(); 00299 _Ps3USB.Led(1); 00300 _Ps3USB.Rumble(0x20,0xff,0x20,0xff); 00301 _Ps3USB.ShowPair(); 00302 00303 } 00304 else StartAutoEvent(device,1,0); 00305 break; 00306 default: 00307 printf("Not yet supported \r\n"); 00308 //StartAutoEvent(device,1,0); 00309 break; 00310 } 00311 }
Generated on Fri Jul 22 2022 14:50:38 by
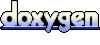