
AMMP demonstration using Freescale FRDM-KL25Z and Adafruit CC3000 Arduino shield
Dependencies: MMA8451Q NVIC_set_all_priorities TSI cc3000_hostdriver_mbedsocket mbed
Fork of AxedaGo-Freescale by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "mbed.h" 00017 #include "cc3000.h" 00018 #include "main.h" 00019 #include "TSISensor.h" 00020 #include "MMA8451Q.h" //accelerometer 00021 #include "TCPSocketConnection.h" 00022 00023 00024 using namespace mbed_cc3000; 00025 00026 /* cc3000 module declaration specific for user's board */ 00027 #if (MY_BOARD == WIFI_SHEILD_ADAFRUIT) 00028 cc3000 wifi(PTA12, PTA5, PTD0, SPI(PTD2, PTD3, PTD1), PORTA_IRQn); 00029 Serial pc(USBTX, USBRX); // tx, rx 00030 #else 00031 00032 #endif 00033 00034 tUserFS user_info; 00035 00036 char str[512]; 00037 00038 MMA8451Q acc(PTE25, PTE24, MMA8451_I2C_ADDRESS); 00039 00040 DigitalOut rled(LED_RED); 00041 DigitalOut gled(LED_GREEN); 00042 // DO NOT Use LED_BLUE - pin PTD1 already in use by WiFi chip 00043 00044 00045 00046 /** 00047 * \brief Print cc3000 information 00048 * \param none 00049 * \return none 00050 */ 00051 void print_cc3000_info() { 00052 uint8_t myMAC[8]; 00053 00054 printf("MAC address + cc3000 info \r\n"); 00055 wifi.get_user_file_info((uint8_t *)&user_info, sizeof(user_info)); 00056 wifi.get_mac_address(myMAC); 00057 printf(" MAC address %02x:%02x:%02x:%02x:%02x:%02x \r\n \r\n", myMAC[0], myMAC[1], myMAC[2], myMAC[3], myMAC[4], myMAC[5]); 00058 00059 printf(" FTC %i \r\n",user_info.FTC); 00060 printf(" PP_version %i.%i \r\n",user_info.PP_version[0], user_info.PP_version[1]); 00061 printf(" SERV_PACK %i.%i \r\n",user_info.SERV_PACK[0], user_info.SERV_PACK[1]); 00062 printf(" DRV_VER %i.%i.%i \r\n",user_info.DRV_VER[0], user_info.DRV_VER[1], user_info.DRV_VER[2]); 00063 printf(" FW_VER %i.%i.%i \r\n",user_info.FW_VER[0], user_info.FW_VER[1], user_info.FW_VER[2]); 00064 } 00065 00066 /** 00067 * \brief Connect to SSID with a timeout 00068 * \param ssid Name of SSID 00069 * \param key Password 00070 * \param sec_mode Security mode 00071 * \return none 00072 */ 00073 void connect_to_ssid(char *ssid, char *key, unsigned char sec_mode) { 00074 printf("Connecting to SSID: %s. Timeout is 10s. \r\n",ssid); 00075 if (wifi.connect_to_AP((uint8_t *)ssid, (uint8_t *)key, sec_mode) == true) { 00076 printf(" Connected. \r\n"); 00077 } else { 00078 printf(" Connection timed-out (error). Please restart. \r\n"); 00079 while(1); 00080 } 00081 } 00082 00083 00084 /** 00085 * \brief HTTP client demo 00086 * \param none 00087 * \return int 00088 */ 00089 int main() { 00090 00091 rled = 1; 00092 gled = 1; 00093 TSISensor touchSensor; 00094 const char* SERVER_ADDRESS = "216.34.120.52"; //IP address for toolbox-stage-connect.axeda.com is 216.34.120.53 00095 //IP address for toolbox-connect.axeda.com is 216.34.120.52 00096 const int SERVER_PORT = 80; 00097 char *MODEL = "Freescale"; 00098 char *SERIAL_NUM = "nlr__jrogers_axeda_com___6243311"; 00099 int http_cmd_sz=800; 00100 char http_cmd[http_cmd_sz]; 00101 int buffer_sz=300; 00102 char buffer[buffer_sz]; 00103 int returnCode = 0; 00104 int weight=0; 00105 TCPSocketConnection socket; 00106 00107 00108 float accels[3]; 00109 float resting,reading; 00110 float THRESHOLD = 0.15; 00111 float position; 00112 00113 00114 pc.printf("Starting up...\n"); 00115 00116 00117 pc.printf("Before wifi.start...\n"); 00118 wifi.start(0); 00119 printf("cc3000 HTTP client demo. \r\n"); 00120 print_cc3000_info(); 00121 00122 printf("Attempting SSID Connection. \r\n"); 00123 00124 wifi._wlan.ioctl_set_connection_policy(0, 0, 0); 00125 00126 connect_to_ssid(SSID, AP_KEY, AP_SECURITY); 00127 00128 printf("send DHCP request \r\n"); 00129 gled =0; 00130 00131 00132 while (wifi.is_dhcp_configured() == false) { 00133 00134 wait_ms(500); 00135 printf(" Waiting for dhcp to be set. \r\n"); 00136 00137 } 00138 00139 gled = 1; rled = 0; 00140 00141 tNetappIpconfigRetArgs ipinfo2; 00142 wifi.get_ip_config(&ipinfo2); // data is returned in the ipinfo2 structure 00143 printf("DHCP assigned IP Address = %d.%d.%d.%d \r\n", ipinfo2.aucIP[3], ipinfo2.aucIP[2], ipinfo2.aucIP[1], ipinfo2.aucIP[0]); 00144 printf(" subnet mask = %d.%d.%d.%d \r\n", ipinfo2.aucSubnetMask[3], ipinfo2.aucSubnetMask[2], ipinfo2.aucSubnetMask[1], ipinfo2.aucSubnetMask[0]); 00145 printf(" gateway = %d.%d.%d.%d \r\n", ipinfo2.aucDefaultGateway[3], ipinfo2.aucDefaultGateway[2], ipinfo2.aucDefaultGateway[1], ipinfo2.aucDefaultGateway[0]); 00146 printf(" DNS IP Address = %d.%d.%d.%d \r\n", ipinfo2.aucDNSServer[3], ipinfo2.aucDNSServer[2], ipinfo2.aucDNSServer[1], ipinfo2.aucDNSServer[0]); 00147 00148 00149 00150 00151 wait(0.7); 00152 acc.getAccAllAxis( accels ); 00153 resting = abs(accels[0]) + abs(accels[1]) + abs(accels[2]); 00154 printf("Set up resting accelerometer - %.2f\r\n", resting); 00155 wait(0.1); 00156 00157 00158 00159 while(1) 00160 { 00161 00162 acc.getAccAllAxis( accels ); 00163 reading = abs(accels[0]) + abs(accels[1]) + abs(accels[2]); 00164 if ( abs( resting - reading ) > THRESHOLD ){ 00165 wait(0.1); 00166 position = touchSensor.readPercentage(); 00167 printf( "BUMPED resting %.2f read %.2f position %.2f \r\n", resting, reading, position ); 00168 rled = 1; 00169 gled = 1; 00170 00171 printf("Before connect\r\n"); 00172 //socket.connect(SERVER_ADDRESS, SERVER_PORT); 00173 while ( socket.connect(SERVER_ADDRESS, SERVER_PORT) < 0) { 00174 printf("Unable to connect to (%s) on port (%d) \r\n", SERVER_ADDRESS, SERVER_PORT); 00175 wait(1); 00176 printf(" - retring to connect"); 00177 } 00178 printf( "connected"); 00179 weight = position * 300; 00180 printf("\n%d\n", weight); 00181 snprintf(http_cmd, http_cmd_sz, "POST /ammp/data/1/%s!%s HTTP/1.1\r\nContent-type: application/json\r\nContent-Length: 52\r\n\r\n{\"data\":[{\"dataItems\":{\"weight\":%d}}]}\r\n\r\n", MODEL, SERIAL_NUM, weight); 00182 socket.send_all(http_cmd, http_cmd_sz-1); 00183 00184 returnCode = socket.receive(buffer, buffer_sz-1); 00185 00186 buffer[returnCode] = '\0'; 00187 printf("Sent->%s<-\r\n",http_cmd); 00188 printf("Received %d chars from server:\n\r%s\n", returnCode, buffer); 00189 00190 socket.close(); 00191 printf("Socket closed\r\n"); 00192 rled = 0; 00193 gled = 0; 00194 00195 //memset null 00196 memset(buffer, '\0', buffer_sz); 00197 memset(http_cmd, '\0', http_cmd_sz); 00198 } 00199 00200 } 00201 00202 }
Generated on Tue Jul 12 2022 21:32:58 by
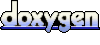