
Axeda Ready Demo for Freescale FRDM-KL46Z as accident alert system
Dependencies: FRDM_MMA8451Q KL46Z-USBHost MAG3110 SocketModem TSI mbed FATFileSystem
Fork of AxedaGo-Freescal_FRDM-KL46Z revert by
cJSON.h
00001 /* 00002 Copyright (c) 2009 Dave Gamble 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef cJSON__h 00024 #define cJSON__h 00025 00026 #ifdef __cplusplus 00027 extern "C" 00028 { 00029 #endif 00030 00031 #include <string.h> 00032 #include <stdio.h> 00033 #include <math.h> 00034 #include <stdlib.h> 00035 #include <float.h> 00036 #include <limits.h> 00037 #include <ctype.h> 00038 00039 /* cJSON Types: */ 00040 #define cJSON_False 0 00041 #define cJSON_True 1 00042 #define cJSON_NULL 2 00043 #define cJSON_Number 3 00044 #define cJSON_String 4 00045 #define cJSON_Array 5 00046 #define cJSON_Object 6 00047 00048 #define cJSON_IsReference 256 00049 00050 /* The cJSON structure: */ 00051 typedef struct cJSON { 00052 struct cJSON *next,*prev; /* next/prev allow you to walk array/object chains. Alternatively, use GetArraySize/GetArrayItem/GetObjectItem */ 00053 struct cJSON *child; /* An array or object item will have a child pointer pointing to a chain of the items in the array/object. */ 00054 00055 int type; /* The type of the item, as above. */ 00056 00057 char *valuestring; /* The item's string, if type==cJSON_String */ 00058 int valueint; /* The item's number, if type==cJSON_Number */ 00059 double valuedouble; /* The item's number, if type==cJSON_Number */ 00060 00061 char *string; /* The item's name string, if this item is the child of, or is in the list of subitems of an object. */ 00062 } cJSON; 00063 00064 typedef struct cJSON_Hooks { 00065 void *(*malloc_fn)(size_t sz); 00066 void (*free_fn)(void *ptr); 00067 } cJSON_Hooks; 00068 00069 /* Supply malloc, realloc and free functions to cJSON */ 00070 extern void cJSON_InitHooks(cJSON_Hooks* hooks); 00071 00072 00073 /* Supply a block of JSON, and this returns a cJSON object you can interrogate. Call cJSON_Delete when finished. */ 00074 extern cJSON *cJSON_Parse(const char *value); 00075 /* Render a cJSON entity to text for transfer/storage. Free the char* when finished. */ 00076 extern char *cJSON_Print(cJSON *item); 00077 /* Render a cJSON entity to text for transfer/storage without any formatting. Free the char* when finished. */ 00078 extern char *cJSON_PrintUnformatted(cJSON *item); 00079 /* Delete a cJSON entity and all subentities. */ 00080 extern void cJSON_Delete(cJSON *c); 00081 00082 /* Returns the number of items in an array (or object). */ 00083 extern int cJSON_GetArraySize(cJSON *array); 00084 /* Retrieve item number "item" from array "array". Returns NULL if unsuccessful. */ 00085 extern cJSON *cJSON_GetArrayItem(cJSON *array,int item); 00086 /* Get item "string" from object. Case insensitive. */ 00087 extern cJSON *cJSON_GetObjectItem(cJSON *object,const char *string); 00088 00089 /* For analysing failed parses. This returns a pointer to the parse error. You'll probably need to look a few chars back to make sense of it. Defined when cJSON_Parse() returns 0. 0 when cJSON_Parse() succeeds. */ 00090 extern const char *cJSON_GetErrorPtr(); 00091 00092 /* These calls create a cJSON item of the appropriate type. */ 00093 extern cJSON *cJSON_CreateNull(); 00094 extern cJSON *cJSON_CreateTrue(); 00095 extern cJSON *cJSON_CreateFalse(); 00096 extern cJSON *cJSON_CreateBool(int b); 00097 extern cJSON *cJSON_CreateNumber(double num); 00098 extern cJSON *cJSON_CreateString(const char *string); 00099 extern cJSON *cJSON_CreateArray(); 00100 extern cJSON *cJSON_CreateObject(); 00101 00102 /* These utilities create an Array of count items. */ 00103 extern cJSON *cJSON_CreateIntArray(int *numbers,int count); 00104 extern cJSON *cJSON_CreateFloatArray(float *numbers,int count); 00105 extern cJSON *cJSON_CreateDoubleArray(double *numbers,int count); 00106 extern cJSON *cJSON_CreateStringArray(const char **strings,int count); 00107 00108 /* Append item to the specified array/object. */ 00109 extern void cJSON_AddItemToArray(cJSON *array, cJSON *item); 00110 extern void cJSON_AddItemToObject(cJSON *object,const char *string,cJSON *item); 00111 /* Append reference to item to the specified array/object. Use this when you want to add an existing cJSON to a new cJSON, but don't want to corrupt your existing cJSON. */ 00112 extern void cJSON_AddItemReferenceToArray(cJSON *array, cJSON *item); 00113 extern void cJSON_AddItemReferenceToObject(cJSON *object,const char *string,cJSON *item); 00114 00115 /* Remove/Detatch items from Arrays/Objects. */ 00116 extern cJSON *cJSON_DetachItemFromArray(cJSON *array,int which); 00117 extern void cJSON_DeleteItemFromArray(cJSON *array,int which); 00118 extern cJSON *cJSON_DetachItemFromObject(cJSON *object,const char *string); 00119 extern void cJSON_DeleteItemFromObject(cJSON *object,const char *string); 00120 00121 /* Update array items. */ 00122 extern void cJSON_ReplaceItemInArray(cJSON *array,int which,cJSON *newitem); 00123 extern void cJSON_ReplaceItemInObject(cJSON *object,const char *string,cJSON *newitem); 00124 00125 #define cJSON_AddNullToObject(object,name) cJSON_AddItemToObject(object, name, cJSON_CreateNull()) 00126 #define cJSON_AddTrueToObject(object,name) cJSON_AddItemToObject(object, name, cJSON_CreateTrue()) 00127 #define cJSON_AddFalseToObject(object,name) cJSON_AddItemToObject(object, name, cJSON_CreateFalse()) 00128 #define cJSON_AddNumberToObject(object,name,n) cJSON_AddItemToObject(object, name, cJSON_CreateNumber(n)) 00129 #define cJSON_AddStringToObject(object,name,s) cJSON_AddItemToObject(object, name, cJSON_CreateString(s)) 00130 00131 #ifdef __cplusplus 00132 } 00133 #endif 00134 00135 #endif 00136
Generated on Wed Jul 13 2022 02:45:01 by
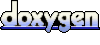