
Axeda Ready Demo for Freescale FRDM-KL46Z as accident alert system
Dependencies: FRDM_MMA8451Q KL46Z-USBHost MAG3110 SocketModem TSI mbed FATFileSystem
Fork of AxedaGo-Freescal_FRDM-KL46Z revert by
cJSON.c
00001 /* 00002 Copyright (c) 2009 Dave Gamble 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 /* cJSON */ 00024 /* JSON parser in C. */ 00025 00026 #include <string.h> 00027 #include <stdio.h> 00028 #include <math.h> 00029 #include <stdlib.h> 00030 #include <float.h> 00031 #include <limits.h> 00032 #include <ctype.h> 00033 #include "cJSON.h" 00034 00035 static const char *ep; 00036 00037 const char *cJSON_GetErrorPtr() {return ep;} 00038 00039 static int cJSON_strcasecmp(const char *s1,const char *s2) 00040 { 00041 if (!s1) return (s1==s2)?0:1;if (!s2) return 1; 00042 for(; tolower(*s1) == tolower(*s2); ++s1, ++s2) if(*s1 == 0) return 0; 00043 return tolower(*(const unsigned char *)s1) - tolower(*(const unsigned char *)s2); 00044 } 00045 00046 static void *(*cJSON_malloc)(size_t sz) = malloc; 00047 static void (*cJSON_free)(void *ptr) = free; 00048 00049 static char* cJSON_strdup(const char* str) 00050 { 00051 size_t len; 00052 char* copy; 00053 00054 len = strlen(str) + 1; 00055 if (!(copy = (char*)cJSON_malloc(len))) return 0; 00056 memcpy(copy,str,len); 00057 return copy; 00058 } 00059 00060 void cJSON_InitHooks(cJSON_Hooks* hooks) 00061 { 00062 if (!hooks) { /* Reset hooks */ 00063 cJSON_malloc = malloc; 00064 cJSON_free = free; 00065 return; 00066 } 00067 00068 cJSON_malloc = (hooks->malloc_fn)?hooks->malloc_fn:malloc; 00069 cJSON_free = (hooks->free_fn)?hooks->free_fn:free; 00070 } 00071 00072 /* Internal constructor. */ 00073 static cJSON *cJSON_New_Item() 00074 { 00075 cJSON* node = (cJSON*)cJSON_malloc(sizeof(cJSON)); 00076 if (node) memset(node,0,sizeof(cJSON)); 00077 return node; 00078 } 00079 00080 /* Delete a cJSON structure. */ 00081 void cJSON_Delete(cJSON *c) 00082 { 00083 cJSON *next; 00084 while (c) 00085 { 00086 next=c->next; 00087 if (!(c->type&cJSON_IsReference) && c->child) cJSON_Delete(c->child); 00088 if (!(c->type&cJSON_IsReference) && c->valuestring) cJSON_free(c->valuestring); 00089 if (c->string) cJSON_free(c->string); 00090 cJSON_free(c); 00091 c=next; 00092 } 00093 } 00094 00095 /* Parse the input text to generate a number, and populate the result into item. */ 00096 static const char *parse_number(cJSON *item,const char *num) 00097 { 00098 double n=0,sign=1,scale=0;int subscale=0,signsubscale=1; 00099 00100 /* Could use sscanf for this? */ 00101 if (*num=='-') sign=-1,num++; /* Has sign? */ 00102 if (*num=='0') num++; /* is zero */ 00103 if (*num>='1' && *num<='9') do n=(n*10.0)+(*num++ -'0'); while (*num>='0' && *num<='9'); /* Number? */ 00104 if (*num=='.' && num[1]>='0' && num[1]<='9') {num++; do n=(n*10.0)+(*num++ -'0'),scale--; while (*num>='0' && *num<='9');} /* Fractional part? */ 00105 if (*num=='e' || *num=='E') /* Exponent? */ 00106 { num++;if (*num=='+') num++; else if (*num=='-') signsubscale=-1,num++; /* With sign? */ 00107 while (*num>='0' && *num<='9') subscale=(subscale*10)+(*num++ - '0'); /* Number? */ 00108 } 00109 00110 n=sign*n*pow(10.0,(scale+subscale*signsubscale)); /* number = +/- number.fraction * 10^+/- exponent */ 00111 00112 item->valuedouble=n; 00113 item->valueint=(int)n; 00114 item->type=cJSON_Number; 00115 return num; 00116 } 00117 00118 /* Render the number nicely from the given item into a string. */ 00119 static char *print_number(cJSON *item) 00120 { 00121 char *str; 00122 double d=item->valuedouble; 00123 if (fabs(((double)item->valueint)-d)<=DBL_EPSILON && d<=INT_MAX && d>=INT_MIN) 00124 { 00125 str=(char*)cJSON_malloc(21); /* 2^64+1 can be represented in 21 chars. */ 00126 if (str) sprintf(str,"%d",item->valueint); 00127 } 00128 else 00129 { 00130 str=(char*)cJSON_malloc(64); /* This is a nice tradeoff. */ 00131 if (str) 00132 { 00133 if (fabs(floor(d)-d)<=DBL_EPSILON) sprintf(str,"%.0f",d); 00134 else if (fabs(d)<1.0e-6 || fabs(d)>1.0e9) sprintf(str,"%e",d); 00135 else sprintf(str,"%f",d); 00136 } 00137 } 00138 return str; 00139 } 00140 00141 /* Parse the input text into an unescaped cstring, and populate item. */ 00142 static const unsigned char firstByteMark[7] = { 0x00, 0x00, 0xC0, 0xE0, 0xF0, 0xF8, 0xFC }; 00143 static const char *parse_string(cJSON *item,const char *str) 00144 { 00145 const char *ptr=str+1;char *ptr2;char *out;int len=0;unsigned uc,uc2; 00146 if (*str!='\"') {ep=str;return 0;} /* not a string! */ 00147 00148 while (*ptr!='\"' && *ptr && ++len) if (*ptr++ == '\\') ptr++; /* Skip escaped quotes. */ 00149 00150 out=(char*)cJSON_malloc(len+1); /* This is how long we need for the string, roughly. */ 00151 if (!out) return 0; 00152 00153 ptr=str+1;ptr2=out; 00154 while (*ptr!='\"' && *ptr) 00155 { 00156 if (*ptr!='\\') *ptr2++=*ptr++; 00157 else 00158 { 00159 ptr++; 00160 switch (*ptr) 00161 { 00162 case 'b': *ptr2++='\b'; break; 00163 case 'f': *ptr2++='\f'; break; 00164 case 'n': *ptr2++='\n'; break; 00165 case 'r': *ptr2++='\r'; break; 00166 case 't': *ptr2++='\t'; break; 00167 case 'u': /* transcode utf16 to utf8. */ 00168 sscanf(ptr+1,"%4x",&uc);ptr+=4; /* get the unicode char. */ 00169 00170 if ((uc>=0xDC00 && uc<=0xDFFF) || uc==0) break; // check for invalid. 00171 00172 if (uc>=0xD800 && uc<=0xDBFF) // UTF16 surrogate pairs. 00173 { 00174 if (ptr[1]!='\\' || ptr[2]!='u') break; // missing second-half of surrogate. 00175 sscanf(ptr+3,"%4x",&uc2);ptr+=6; 00176 if (uc2<0xDC00 || uc2>0xDFFF) break; // invalid second-half of surrogate. 00177 uc=0x10000 | ((uc&0x3FF)<<10) | (uc2&0x3FF); 00178 } 00179 00180 len=4;if (uc<0x80) len=1;else if (uc<0x800) len=2;else if (uc<0x10000) len=3; ptr2+=len; 00181 00182 switch (len) { 00183 case 4: *--ptr2 =((uc | 0x80) & 0xBF); uc >>= 6; 00184 case 3: *--ptr2 =((uc | 0x80) & 0xBF); uc >>= 6; 00185 case 2: *--ptr2 =((uc | 0x80) & 0xBF); uc >>= 6; 00186 case 1: *--ptr2 =(uc | firstByteMark[len]); 00187 } 00188 ptr2+=len; 00189 break; 00190 default: *ptr2++=*ptr; break; 00191 } 00192 ptr++; 00193 } 00194 } 00195 *ptr2=0; 00196 if (*ptr=='\"') ptr++; 00197 item->valuestring=out; 00198 item->type=cJSON_String; 00199 return ptr; 00200 } 00201 00202 /* Render the cstring provided to an escaped version that can be printed. */ 00203 static char *print_string_ptr(const char *str) 00204 { 00205 const char *ptr;char *ptr2,*out;int len=0;unsigned char token; 00206 00207 if (!str) return cJSON_strdup(""); 00208 ptr=str;while ((token=*ptr) && ++len) {if (strchr("\"\\\b\f\n\r\t",token)) len++; else if (token<32) len+=5;ptr++;} 00209 00210 out=(char*)cJSON_malloc(len+3); 00211 if (!out) return 0; 00212 00213 ptr2=out;ptr=str; 00214 *ptr2++='\"'; 00215 while (*ptr) 00216 { 00217 if ((unsigned char)*ptr>31 && *ptr!='\"' && *ptr!='\\') *ptr2++=*ptr++; 00218 else 00219 { 00220 *ptr2++='\\'; 00221 switch (token=*ptr++) 00222 { 00223 case '\\': *ptr2++='\\'; break; 00224 case '\"': *ptr2++='\"'; break; 00225 case '\b': *ptr2++='b'; break; 00226 case '\f': *ptr2++='f'; break; 00227 case '\n': *ptr2++='n'; break; 00228 case '\r': *ptr2++='r'; break; 00229 case '\t': *ptr2++='t'; break; 00230 default: sprintf(ptr2,"u%04x",token);ptr2+=5; break; /* escape and print */ 00231 } 00232 } 00233 } 00234 *ptr2++='\"';*ptr2++=0; 00235 return out; 00236 } 00237 /* Invote print_string_ptr (which is useful) on an item. */ 00238 static char *print_string(cJSON *item) {return print_string_ptr(item->valuestring);} 00239 00240 /* Predeclare these prototypes. */ 00241 static const char *parse_value(cJSON *item,const char *value); 00242 static char *print_value(cJSON *item,int depth,int fmt); 00243 static const char *parse_array(cJSON *item,const char *value); 00244 static char *print_array(cJSON *item,int depth,int fmt); 00245 static const char *parse_object(cJSON *item,const char *value); 00246 static char *print_object(cJSON *item,int depth,int fmt); 00247 00248 /* Utility to jump whitespace and cr/lf */ 00249 static const char *skip(const char *in) {while (in && *in && (unsigned char)*in<=32) in++; return in;} 00250 00251 /* Parse an object - create a new root, and populate. */ 00252 cJSON *cJSON_Parse(const char *value) 00253 { 00254 cJSON *c=cJSON_New_Item(); 00255 ep=0; 00256 if (!c) return 0; /* memory fail */ 00257 00258 if (!parse_value(c,skip(value))) {cJSON_Delete(c);return 0;} 00259 return c; 00260 } 00261 00262 /* Render a cJSON item/entity/structure to text. */ 00263 char *cJSON_Print(cJSON *item) {return print_value(item,0,1);} 00264 char *cJSON_PrintUnformatted(cJSON *item) {return print_value(item,0,0);} 00265 00266 /* Parser core - when encountering text, process appropriately. */ 00267 static const char *parse_value(cJSON *item,const char *value) 00268 { 00269 if (!value) return 0; /* Fail on null. */ 00270 if (!strncmp(value,"null",4)) { item->type=cJSON_NULL; return value+4; } 00271 if (!strncmp(value,"false",5)) { item->type=cJSON_False; return value+5; } 00272 if (!strncmp(value,"true",4)) { item->type=cJSON_True; item->valueint=1; return value+4; } 00273 if (*value=='\"') { return parse_string(item,value); } 00274 if (*value=='-' || (*value>='0' && *value<='9')) { return parse_number(item,value); } 00275 if (*value=='[') { return parse_array(item,value); } 00276 if (*value=='{') { return parse_object(item,value); } 00277 00278 ep=value;return 0; /* failure. */ 00279 } 00280 00281 /* Render a value to text. */ 00282 static char *print_value(cJSON *item,int depth,int fmt) 00283 { 00284 char *out=0; 00285 if (!item) return 0; 00286 switch ((item->type)&255) 00287 { 00288 case cJSON_NULL: out=cJSON_strdup("null"); break; 00289 case cJSON_False: out=cJSON_strdup("false");break; 00290 case cJSON_True: out=cJSON_strdup("true"); break; 00291 case cJSON_Number: out=print_number(item);break; 00292 case cJSON_String: out=print_string(item);break; 00293 case cJSON_Array: out=print_array(item,depth,fmt);break; 00294 case cJSON_Object: out=print_object(item,depth,fmt);break; 00295 } 00296 return out; 00297 } 00298 00299 /* Build an array from input text. */ 00300 static const char *parse_array(cJSON *item,const char *value) 00301 { 00302 cJSON *child; 00303 if (*value!='[') {ep=value;return 0;} /* not an array! */ 00304 00305 item->type=cJSON_Array; 00306 value=skip(value+1); 00307 if (*value==']') return value+1; /* empty array. */ 00308 00309 item->child=child=cJSON_New_Item(); 00310 if (!item->child) return 0; /* memory fail */ 00311 value=skip(parse_value(child,skip(value))); /* skip any spacing, get the value. */ 00312 if (!value) return 0; 00313 00314 while (*value==',') 00315 { 00316 cJSON *new_item; 00317 if (!(new_item=cJSON_New_Item())) return 0; /* memory fail */ 00318 child->next=new_item;new_item->prev=child;child=new_item; 00319 value=skip(parse_value(child,skip(value+1))); 00320 if (!value) return 0; /* memory fail */ 00321 } 00322 00323 if (*value==']') return value+1; /* end of array */ 00324 ep=value;return 0; /* malformed. */ 00325 } 00326 00327 /* Render an array to text */ 00328 static char *print_array(cJSON *item,int depth,int fmt) 00329 { 00330 char **entries; 00331 char *out=0,*ptr,*ret;int len=5; 00332 cJSON *child=item->child; 00333 int numentries=0,i=0,fail=0; 00334 00335 /* How many entries in the array? */ 00336 while (child) numentries++,child=child->next; 00337 /* Allocate an array to hold the values for each */ 00338 entries=(char**)cJSON_malloc(numentries*sizeof(char*)); 00339 if (!entries) return 0; 00340 memset(entries,0,numentries*sizeof(char*)); 00341 /* Retrieve all the results: */ 00342 child=item->child; 00343 while (child && !fail) 00344 { 00345 ret=print_value(child,depth+1,fmt); 00346 entries[i++]=ret; 00347 if (ret) len+=strlen(ret)+2+(fmt?1:0); else fail=1; 00348 child=child->next; 00349 } 00350 00351 /* If we didn't fail, try to malloc the output string */ 00352 if (!fail) out=(char*)cJSON_malloc(len); 00353 /* If that fails, we fail. */ 00354 if (!out) fail=1; 00355 00356 /* Handle failure. */ 00357 if (fail) 00358 { 00359 for (i=0;i<numentries;i++) if (entries[i]) cJSON_free(entries[i]); 00360 cJSON_free(entries); 00361 return 0; 00362 } 00363 00364 /* Compose the output array. */ 00365 *out='['; 00366 ptr=out+1;*ptr=0; 00367 for (i=0;i<numentries;i++) 00368 { 00369 strcpy(ptr,entries[i]);ptr+=strlen(entries[i]); 00370 if (i!=numentries-1) {*ptr++=',';if(fmt)*ptr++=' ';*ptr=0;} 00371 cJSON_free(entries[i]); 00372 } 00373 cJSON_free(entries); 00374 *ptr++=']';*ptr++=0; 00375 return out; 00376 } 00377 00378 /* Build an object from the text. */ 00379 static const char *parse_object(cJSON *item,const char *value) 00380 { 00381 cJSON *child; 00382 if (*value!='{') {ep=value;return 0;} /* not an object! */ 00383 00384 item->type=cJSON_Object; 00385 value=skip(value+1); 00386 if (*value=='}') return value+1; /* empty array. */ 00387 00388 item->child=child=cJSON_New_Item(); 00389 if (!item->child) return 0; 00390 value=skip(parse_string(child,skip(value))); 00391 if (!value) return 0; 00392 child->string=child->valuestring;child->valuestring=0; 00393 if (*value!=':') {ep=value;return 0;} /* fail! */ 00394 value=skip(parse_value(child,skip(value+1))); /* skip any spacing, get the value. */ 00395 if (!value) return 0; 00396 00397 while (*value==',') 00398 { 00399 cJSON *new_item; 00400 if (!(new_item=cJSON_New_Item())) return 0; /* memory fail */ 00401 child->next=new_item;new_item->prev=child;child=new_item; 00402 value=skip(parse_string(child,skip(value+1))); 00403 if (!value) return 0; 00404 child->string=child->valuestring;child->valuestring=0; 00405 if (*value!=':') {ep=value;return 0;} /* fail! */ 00406 value=skip(parse_value(child,skip(value+1))); /* skip any spacing, get the value. */ 00407 if (!value) return 0; 00408 } 00409 00410 if (*value=='}') return value+1; /* end of array */ 00411 ep=value;return 0; /* malformed. */ 00412 } 00413 00414 /* Render an object to text. */ 00415 static char *print_object(cJSON *item,int depth,int fmt) 00416 { 00417 char **entries=0,**names=0; 00418 char *out=0,*ptr,*ret,*str;int len=7,i=0,j; 00419 cJSON *child=item->child; 00420 int numentries=0,fail=0; 00421 /* Count the number of entries. */ 00422 while (child) numentries++,child=child->next; 00423 /* Allocate space for the names and the objects */ 00424 entries=(char**)cJSON_malloc(numentries*sizeof(char*)); 00425 if (!entries) return 0; 00426 names=(char**)cJSON_malloc(numentries*sizeof(char*)); 00427 if (!names) {cJSON_free(entries);return 0;} 00428 memset(entries,0,sizeof(char*)*numentries); 00429 memset(names,0,sizeof(char*)*numentries); 00430 00431 /* Collect all the results into our arrays: */ 00432 child=item->child;depth++;if (fmt) len+=depth; 00433 while (child) 00434 { 00435 names[i]=str=print_string_ptr(child->string); 00436 entries[i++]=ret=print_value(child,depth,fmt); 00437 if (str && ret) len+=strlen(ret)+strlen(str)+2+(fmt?2+depth:0); else fail=1; 00438 child=child->next; 00439 } 00440 00441 /* Try to allocate the output string */ 00442 if (!fail) out=(char*)cJSON_malloc(len); 00443 if (!out) fail=1; 00444 00445 /* Handle failure */ 00446 if (fail) 00447 { 00448 for (i=0;i<numentries;i++) {if (names[i]) cJSON_free(names[i]);if (entries[i]) cJSON_free(entries[i]);} 00449 cJSON_free(names);cJSON_free(entries); 00450 return 0; 00451 } 00452 00453 /* Compose the output: */ 00454 *out='{';ptr=out+1;if (fmt)*ptr++='\n';*ptr=0; 00455 for (i=0;i<numentries;i++) 00456 { 00457 if (fmt) for (j=0;j<depth;j++) *ptr++='\t'; 00458 strcpy(ptr,names[i]);ptr+=strlen(names[i]); 00459 *ptr++=':';if (fmt) *ptr++='\t'; 00460 strcpy(ptr,entries[i]);ptr+=strlen(entries[i]); 00461 if (i!=numentries-1) *ptr++=','; 00462 if (fmt) *ptr++='\n';*ptr=0; 00463 cJSON_free(names[i]);cJSON_free(entries[i]); 00464 } 00465 00466 cJSON_free(names);cJSON_free(entries); 00467 if (fmt) for (i=0;i<depth-1;i++) *ptr++='\t'; 00468 *ptr++='}';*ptr++=0; 00469 return out; 00470 } 00471 00472 /* Get Array size/item / object item. */ 00473 int cJSON_GetArraySize(cJSON *array) {cJSON *c=array->child;int i=0;while(c)i++,c=c->next;return i;} 00474 cJSON *cJSON_GetArrayItem(cJSON *array,int item) {cJSON *c=array->child; while (c && item>0) item--,c=c->next; return c;} 00475 cJSON *cJSON_GetObjectItem(cJSON *object,const char *string) {cJSON *c=object->child; while (c && cJSON_strcasecmp(c->string,string)) c=c->next; return c;} 00476 00477 /* Utility for array list handling. */ 00478 static void suffix_object(cJSON *prev,cJSON *item) {prev->next=item;item->prev=prev;} 00479 /* Utility for handling references. */ 00480 static cJSON *create_reference(cJSON *item) {cJSON *ref=cJSON_New_Item();if (!ref) return 0;memcpy(ref,item,sizeof(cJSON));ref->string=0;ref->type|=cJSON_IsReference;ref->next=ref->prev=0;return ref;} 00481 00482 /* Add item to array/object. */ 00483 void cJSON_AddItemToArray(cJSON *array, cJSON *item) {cJSON *c=array->child;if (!item) return; if (!c) {array->child=item;} else {while (c && c->next) c=c->next; suffix_object(c,item);}} 00484 void cJSON_AddItemToObject(cJSON *object,const char *string,cJSON *item) {if (!item) return; if (item->string) cJSON_free(item->string);item->string=cJSON_strdup(string);cJSON_AddItemToArray(object,item);} 00485 void cJSON_AddItemReferenceToArray(cJSON *array, cJSON *item) {cJSON_AddItemToArray(array,create_reference(item));} 00486 void cJSON_AddItemReferenceToObject(cJSON *object,const char *string,cJSON *item) {cJSON_AddItemToObject(object,string,create_reference(item));} 00487 00488 cJSON *cJSON_DetachItemFromArray(cJSON *array,int which) {cJSON *c=array->child;while (c && which>0) c=c->next,which--;if (!c) return 0; 00489 if (c->prev) c->prev->next=c->next;if (c->next) c->next->prev=c->prev;if (c==array->child) array->child=c->next;c->prev=c->next=0;return c;} 00490 void cJSON_DeleteItemFromArray(cJSON *array,int which) {cJSON_Delete(cJSON_DetachItemFromArray(array,which));} 00491 cJSON *cJSON_DetachItemFromObject(cJSON *object,const char *string) {int i=0;cJSON *c=object->child;while (c && cJSON_strcasecmp(c->string,string)) i++,c=c->next;if (c) return cJSON_DetachItemFromArray(object,i);return 0;} 00492 void cJSON_DeleteItemFromObject(cJSON *object,const char *string) {cJSON_Delete(cJSON_DetachItemFromObject(object,string));} 00493 00494 /* Replace array/object items with new ones. */ 00495 void cJSON_ReplaceItemInArray(cJSON *array,int which,cJSON *newitem) {cJSON *c=array->child;while (c && which>0) c=c->next,which--;if (!c) return; 00496 newitem->next=c->next;newitem->prev=c->prev;if (newitem->next) newitem->next->prev=newitem; 00497 if (c==array->child) array->child=newitem; else newitem->prev->next=newitem;c->next=c->prev=0;cJSON_Delete(c);} 00498 void cJSON_ReplaceItemInObject(cJSON *object,const char *string,cJSON *newitem){int i=0;cJSON *c=object->child;while(c && cJSON_strcasecmp(c->string,string))i++,c=c->next;if(c){newitem->string=cJSON_strdup(string);cJSON_ReplaceItemInArray(object,i,newitem);}} 00499 00500 /* Create basic types: */ 00501 cJSON *cJSON_CreateNull() {cJSON *item=cJSON_New_Item();if(item)item->type=cJSON_NULL;return item;} 00502 cJSON *cJSON_CreateTrue() {cJSON *item=cJSON_New_Item();if(item)item->type=cJSON_True;return item;} 00503 cJSON *cJSON_CreateFalse() {cJSON *item=cJSON_New_Item();if(item)item->type=cJSON_False;return item;} 00504 cJSON *cJSON_CreateBool(int b) {cJSON *item=cJSON_New_Item();if(item)item->type=b?cJSON_True:cJSON_False;return item;} 00505 cJSON *cJSON_CreateNumber(double num) {cJSON *item=cJSON_New_Item();if(item){item->type=cJSON_Number;item->valuedouble=num;item->valueint=(int)num;}return item;} 00506 cJSON *cJSON_CreateString(const char *string) {cJSON *item=cJSON_New_Item();if(item){item->type=cJSON_String;item->valuestring=cJSON_strdup(string);}return item;} 00507 cJSON *cJSON_CreateArray() {cJSON *item=cJSON_New_Item();if(item)item->type=cJSON_Array;return item;} 00508 cJSON *cJSON_CreateObject() {cJSON *item=cJSON_New_Item();if(item)item->type=cJSON_Object;return item;} 00509 00510 /* Create Arrays: */ 00511 cJSON *cJSON_CreateIntArray(int *numbers,int count) {int i;cJSON *n=0,*p=0,*a=cJSON_CreateArray();for(i=0;a && i<count;i++){n=cJSON_CreateNumber(numbers[i]);if(!i)a->child=n;else suffix_object(p,n);p=n;}return a;} 00512 cJSON *cJSON_CreateFloatArray(float *numbers,int count) {int i;cJSON *n=0,*p=0,*a=cJSON_CreateArray();for(i=0;a && i<count;i++){n=cJSON_CreateNumber(numbers[i]);if(!i)a->child=n;else suffix_object(p,n);p=n;}return a;} 00513 cJSON *cJSON_CreateDoubleArray(double *numbers,int count) {int i;cJSON *n=0,*p=0,*a=cJSON_CreateArray();for(i=0;a && i<count;i++){n=cJSON_CreateNumber(numbers[i]);if(!i)a->child=n;else suffix_object(p,n);p=n;}return a;} 00514 cJSON *cJSON_CreateStringArray(const char **strings,int count) {int i;cJSON *n=0,*p=0,*a=cJSON_CreateArray();for(i=0;a && i<count;i++){n=cJSON_CreateString(strings[i]);if(!i)a->child=n;else suffix_object(p,n);p=n;}return a;} 00515
Generated on Wed Jul 13 2022 02:45:01 by
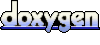