
Axeda Ready Demo for Freescale FRDM-KL46Z as accident alert system
Dependencies: FRDM_MMA8451Q KL46Z-USBHost MAG3110 SocketModem TSI mbed FATFileSystem
Fork of AxedaGo-Freescal_FRDM-KL46Z revert by
axSerializer.c
00001 /************************************************************************************/ 00002 /* axSerializer.c */ 00003 /* �2013 Axeda Corporation */ 00004 /* */ 00005 /* Defines methods for transposing the data in domain objects into a serialized */ 00006 /* encoding for transmission to the Platform Endpoint. This is a platform independent*/ 00007 /* implementation that can be applied to any device that supports ANSI C. */ 00008 /* */ 00009 /************************************************************************************/ 00010 #include "axSerializer.h" 00011 00012 #define REG_ID 0 00013 #define REG_MODEL_NUMBER 1 00014 #define REG_SERIAL_NUMBER 2 00015 #define REG_TENANT 3 00016 #define REG_PING 4 00017 #define REG_KEY 5 00018 00019 #define DATA_ALERTS 0 00020 #define DATA_EVENTS 1 00021 #define DATA_DATA 2 00022 #define DATA_LOCATIONS 3 00023 #define DATA_ITEMS 4 00024 00025 #define PKG_STATUS 0 00026 #define PKG_ERROR 1 00027 00028 #define ALERT_SEV 0 00029 #define ALERT_CAUSE 1 00030 #define ALERT_REASON 2 00031 00032 #define COM_TIME 0 00033 #define COM_PRIORITY 1 00034 #define COM_NAME 2 00035 #define COM_DESCRIPTION 3 00036 00037 //#define EVENT_NAME 1 00038 //#define EVENT_DESC 0 00039 00040 #define LOC_LAT 0 00041 #define LOC_LON 1 00042 #define LOC_ALT 2 00043 00044 int terse_enable=AX_TRUE; 00045 00046 const char *commonKW[]= {"time", "priority", "name", "description" }; 00047 const char *dataKW[]={ "alerts", "events", "data", "locations", "dataItems"}; 00048 const char *registrationKW[]= {"id", "mn", "sn", "tn", "pingRate", "key"}; 00049 const char *alertKW[]= {"severity","cause", "reason" }; 00050 const char *locationKW[]= {"latitude", "longitude", "altitude"}; 00051 const char *pkgStatusKW[]={"status", "error"}; 00052 00053 const char *terse_commonKW[]= {"t", "dp", "n", "d"}; 00054 const char *terse_dataKW[]={ "alerts", "events", "data", "locations", "di"}; 00055 const char *terse_registrationKW[]= {"id", "mn", "sn", "tn", "pr", "k"}; 00056 const char *terse_alertKW[]= {"sv", "cn", "cr" }; 00057 const char *terse_locationKW[]= {"lat", "lon", "alt"}; 00058 const char *terse_pkgStatusKW[]={"st", "err"}; 00059 /*************************************************************************************************/ 00060 /*dataSet2JSON() */ 00061 /* */ 00062 /*Takes an array of ax_dataItem structs and creates JSON to represent them in the AMMP-json */ 00063 /*protocol. The return type will be a CJSON pointer which can then be rendered into an actual */ 00064 /*JSON string. The JSON pointer will need to be free'd when you're done. If there are zero data */ 00065 /*items in the array then this method will return an empty JSON array pointer. */ 00066 /* */ 00067 /*NOTE: If any array spots are left empty they will only be ignored if theyre are NULL. Junk */ 00068 /* values from left over data will cause seg faults. NULL your empty pointers!!! */ 00069 /*************************************************************************************************/ 00070 //takes data item set, not longer just data item structs. 00071 cJSON *dataSet2JSON(ax_dataSet *di[], int len, int terse_on) 00072 { 00073 cJSON *root, *child_obj, *dis=NULL; 00074 int ctr=0; 00075 00076 ax_dataSet *curr=NULL; 00077 ax_dataNode *currNode; 00078 root=cJSON_CreateArray(); 00079 00080 for(ctr=0; ctr<len; ctr++) 00081 { 00082 curr=di[ctr]; 00083 if((curr!=NULL)&&(curr->dataNode_ct>0)) //If this set doesn't have any data in it, don't include it. 00084 { 00085 cJSON_AddItemToArray(root, child_obj=cJSON_CreateObject()); 00086 if(terse_on==AX_TRUE) { 00087 cJSON_AddItemToObject(child_obj, terse_dataKW[DATA_ITEMS], dis=cJSON_CreateObject()); } 00088 else { 00089 cJSON_AddItemToObject(child_obj, dataKW[DATA_ITEMS], dis=cJSON_CreateObject()); 00090 } 00091 if((curr->acquisitionTime>=0)&&(curr->acquisitionTime)){ 00092 if(terse_on==AX_TRUE){ 00093 cJSON_AddNumberToObject(child_obj, terse_commonKW[COM_TIME], curr->acquisitionTime); 00094 } 00095 else { 00096 cJSON_AddNumberToObject(child_obj, commonKW[COM_TIME], curr->acquisitionTime); 00097 } 00098 } 00099 if((curr->priority>=1)&&(curr->priority<=100)) { 00100 if(terse_on==AX_TRUE){ 00101 cJSON_AddNumberToObject(child_obj, terse_commonKW[COM_PRIORITY], curr->priority); 00102 } 00103 else { 00104 cJSON_AddNumberToObject(child_obj, commonKW[COM_PRIORITY], curr->priority); 00105 } 00106 } 00107 currNode=curr->data_first; 00108 while(currNode!=NULL) 00109 { 00110 if(currNode->type==AX_DIGITAL) { 00111 if(currNode->dValue==1) { 00112 cJSON_AddTrueToObject(dis, currNode->name); 00113 } 00114 else { 00115 cJSON_AddFalseToObject(dis, currNode->name); 00116 } 00117 } 00118 else if(currNode->type==AX_ANALOG) { 00119 cJSON_AddNumberToObject(dis, currNode->name, currNode->dValue); 00120 } 00121 else { 00122 cJSON_AddStringToObject(dis, currNode->name, currNode->sValue); 00123 } 00124 //advance to the next data item 00125 currNode=currNode->next; 00126 } 00127 } 00128 } 00129 return root; 00130 } 00131 /*************************************************************************************************/ 00132 /* eventsToJSON() */ 00133 /* */ 00134 /*Takes an array of ax_event struct pointers and creates JSON to represent them in the AMMP-json */ 00135 /*protocol. The return type will be a CJSON pointer which can then be rendered into an actual */ 00136 /*JSON string. The JSON pointer will need to be free'd when you're done. If there are zero data */ 00137 /*items in the array then this method will return an empty JSON array pointer. */ 00138 /* */ 00139 /*NOTE: If any array spots are left empty they will only be ignored if theyre are NULL. Junk */ 00140 /* values from left over data will cause seg faults. NULL your empty pointers!!! */ 00141 /*************************************************************************************************/ 00142 cJSON *eventsToJSON(ax_event *events[], int len, int terse_on) { 00143 cJSON *root, *dis; 00144 ax_event *curr; 00145 int ctr=0; 00146 root=cJSON_CreateArray(); 00147 curr=events[0]; 00148 for(ctr=0; ctr<len; ctr++) 00149 { 00150 curr=events[ctr]; 00151 if(curr!=NULL) 00152 { 00153 cJSON_AddItemToArray(root, dis=cJSON_CreateObject()); 00154 if(terse_on==AX_TRUE){ 00155 cJSON_AddStringToObject(dis, terse_commonKW[COM_NAME], curr->name); 00156 cJSON_AddStringToObject(dis, terse_commonKW[COM_DESCRIPTION], curr->description); 00157 } 00158 else { 00159 cJSON_AddStringToObject(dis, commonKW[COM_NAME], curr->name); 00160 cJSON_AddStringToObject(dis, commonKW[COM_DESCRIPTION], curr->description); 00161 } 00162 00163 if((curr->dateAcquired>=0)&&(curr->dateAcquired)){ 00164 if(terse_on==AX_TRUE){ 00165 cJSON_AddNumberToObject(dis, terse_commonKW[COM_TIME], curr->dateAcquired); 00166 } 00167 else { 00168 cJSON_AddNumberToObject(dis, commonKW[COM_TIME], curr->dateAcquired); 00169 } 00170 } 00171 if((curr->priority>=1)&&(curr->priority<=100)&&(curr->priority)) { 00172 if(terse_on==AX_TRUE){ 00173 cJSON_AddNumberToObject(dis, terse_commonKW[COM_PRIORITY], curr->priority); 00174 } 00175 else { 00176 cJSON_AddNumberToObject(dis, commonKW[COM_PRIORITY], curr->priority); 00177 } 00178 } 00179 } 00180 } 00181 00182 return root; 00183 } 00184 /*************************************************************************************************/ 00185 /* locationsToJSON() */ 00186 /* */ 00187 /*Takes an array of ax_location struct pointers and creates JSON to represent them in the AMMP */ 00188 /*protocol. The return type will be a CJSON pointer which can then be rendered into an actual */ 00189 /*JSON string. The JSON pointer will need to be free'd when you're done. If there are zero data */ 00190 /*items in the array then this method will return an empty JSON array pointer. */ 00191 /* */ 00192 /*NOTE: If any array spots are left empty they will only be ignored if theyre are NULL. Junk */ 00193 /* values from left over data will cause seg faults. NULL your empty pointers!!! */ 00194 /*************************************************************************************************/ 00195 cJSON *locationsToJSON(ax_location *locations[], int len, int terse_on) { 00196 cJSON *root, *dis; 00197 ax_location *curr; 00198 int ctr=0; 00199 root=cJSON_CreateArray(); 00200 for(ctr=0; ctr<len; ctr++) 00201 { 00202 curr=locations[ctr]; 00203 if(curr) 00204 { 00205 cJSON_AddItemToArray(root, dis=cJSON_CreateObject()); 00206 if(terse_on==AX_TRUE){ 00207 cJSON_AddNumberToObject(dis, terse_locationKW[LOC_LAT], curr->latitude); 00208 cJSON_AddNumberToObject(dis, terse_locationKW[LOC_LON], curr->longitude); 00209 cJSON_AddNumberToObject(dis, terse_locationKW[LOC_ALT], curr->altitude); 00210 } 00211 else { 00212 cJSON_AddNumberToObject(dis, locationKW[LOC_LAT], curr->latitude); 00213 cJSON_AddNumberToObject(dis, locationKW[LOC_LON], curr->longitude); 00214 cJSON_AddNumberToObject(dis, locationKW[LOC_ALT], curr->altitude); 00215 } 00216 if((curr->dateAcquired)&&(curr->dateAcquired>=0)){ 00217 if(terse_on==AX_TRUE){ 00218 cJSON_AddNumberToObject(dis, terse_commonKW[COM_TIME], curr->dateAcquired); 00219 } 00220 else { 00221 cJSON_AddNumberToObject(dis, commonKW[COM_TIME], curr->dateAcquired); 00222 } 00223 } 00224 if((curr->priority>=1)&&(curr->priority<=100)&&(curr->priority)) { 00225 if(terse_on==AX_TRUE){ 00226 cJSON_AddNumberToObject(dis, terse_commonKW[COM_PRIORITY], curr->priority); 00227 } 00228 else { 00229 cJSON_AddNumberToObject(dis, commonKW[COM_PRIORITY], curr->priority); 00230 } 00231 } 00232 } 00233 } 00234 00235 return root; 00236 } 00237 00238 /*************************************************************************************************/ 00239 /* alarmsToJSON() */ 00240 /* */ 00241 /*Takes an array of ax_alarm struct pointers and creates JSON to represent them in the AMMP-json */ 00242 /*protocol. The return type will be a CJSON pointer which can then be rendered into an actual */ 00243 /*JSON string. The JSON pointer will need to be free'd when you're done. If there are zero data */ 00244 /*items in the array then this method will return an empty JSON array pointer. */ 00245 /* */ 00246 /*NOTE: If any array spots are left empty they will only be ignored if theyre are NULL. Junk */ 00247 /* values from left over data will cause seg faults. NULL your empty pointers!!! */ 00248 /*************************************************************************************************/ 00249 cJSON *AlarmsToJSON(ax_alarm *alarms[], int len, int terse_on) 00250 { 00251 cJSON *root, *dis; 00252 int ctr=0; 00253 ax_alarm *curr; 00254 root=cJSON_CreateArray(); 00255 curr=alarms[0]; 00256 for(ctr=0; ctr<len; ctr++) { 00257 curr=alarms[ctr]; 00258 if(curr!=NULL) 00259 { 00260 cJSON_AddItemToArray(root, dis=cJSON_CreateObject()); 00261 if(terse_on==AX_TRUE){ 00262 cJSON_AddStringToObject(dis, terse_commonKW[COM_NAME], curr->alarmName); 00263 cJSON_AddStringToObject(dis, terse_commonKW[COM_DESCRIPTION], curr->alarmDescription); 00264 cJSON_AddNumberToObject(dis, terse_alertKW[ALERT_SEV], curr->alarmSeverity); 00265 cJSON_AddStringToObject(dis, terse_alertKW[ALERT_CAUSE], curr->alarmCause); 00266 cJSON_AddStringToObject(dis, terse_alertKW[ALERT_REASON], curr->alarmReason); 00267 } 00268 else { 00269 cJSON_AddStringToObject(dis, commonKW[COM_NAME], curr->alarmName); 00270 cJSON_AddStringToObject(dis, commonKW[COM_DESCRIPTION], curr->alarmDescription); 00271 cJSON_AddNumberToObject(dis, alertKW[ALERT_SEV], curr->alarmSeverity); 00272 cJSON_AddStringToObject(dis, alertKW[ALERT_CAUSE], curr->alarmCause); 00273 cJSON_AddStringToObject(dis, alertKW[ALERT_REASON], curr->alarmReason); 00274 } 00275 00276 if((curr->dateAcquired)&&(curr->dateAcquired>=0)){ 00277 if(terse_on==AX_TRUE){ 00278 cJSON_AddNumberToObject(dis, terse_commonKW[COM_TIME], curr->dateAcquired); 00279 } 00280 else { 00281 cJSON_AddNumberToObject(dis, commonKW[COM_TIME], curr->dateAcquired); 00282 } 00283 } 00284 if((curr->priority>=1)&&(curr->priority<=100)&&(curr->priority)) { 00285 if(terse_on==AX_TRUE){ 00286 cJSON_AddNumberToObject(dis, terse_commonKW[COM_PRIORITY], curr->priority); 00287 } 00288 else { 00289 cJSON_AddNumberToObject(dis, commonKW[COM_PRIORITY], curr->priority); 00290 } 00291 } 00292 } 00293 } 00294 00295 return root; 00296 } 00297 /*************************************************************************************************/ 00298 /* getRegistrationJSON() */ 00299 /* */ 00300 /*Takes a device and ping rate and will return a cJSON structure that represents a registration */ 00301 /*message in the AMMP protocol. */ 00302 /* */ 00303 /*************************************************************************************************/ 00304 cJSON *getRegistrationJSON(ax_deviceID *device, int pingRate) 00305 { 00306 cJSON *root, *child; 00307 00308 root=cJSON_CreateObject(); 00309 00310 cJSON_AddItemToObject(root, registrationKW[REG_ID], child=cJSON_CreateObject()); 00311 if(device->model) { 00312 cJSON_AddStringToObject(child, registrationKW[REG_MODEL_NUMBER], device->model); 00313 } 00314 if(device->serial) { 00315 cJSON_AddStringToObject(child, registrationKW[REG_SERIAL_NUMBER], device->serial); 00316 } 00317 if(strlen(device->tenant)>=1) 00318 { 00319 cJSON_AddStringToObject(child, registrationKW[REG_TENANT], device->tenant); 00320 } 00321 cJSON_AddNumberToObject(root, registrationKW[REG_PING], pingRate); 00322 00323 00324 return root; 00325 } 00326 00327 //TODO: Fill out this description 00328 /*************************************************************************************************/ 00329 /*getPKGStatusJSON() */ 00330 /* */ 00331 /* */ 00332 /* */ 00333 /*************************************************************************************************/ 00334 cJSON *getPKGStatusJSON(int status, char *error, int priority, int time, int terse_on){ 00335 cJSON *root; 00336 root=cJSON_CreateObject(); 00337 //STATUS 00338 if(terse_on==AX_TRUE) { 00339 cJSON_AddNumberToObject(root, pkgStatusKW[PKG_STATUS], status); 00340 } 00341 else { 00342 cJSON_AddNumberToObject(root, pkgStatusKW[PKG_STATUS], status); 00343 } 00344 //TIME 00345 if(time>=0) { 00346 if(terse_on==AX_TRUE) { 00347 cJSON_AddNumberToObject(root, terse_commonKW[COM_TIME], time); 00348 } 00349 else { 00350 cJSON_AddNumberToObject(root, commonKW[COM_TIME], time); 00351 } 00352 } 00353 //PRIORITY 00354 if((priority>0)&&(priority<=100)) { 00355 if(terse_on==AX_TRUE) { 00356 cJSON_AddNumberToObject(root, terse_commonKW[COM_PRIORITY], priority); 00357 } 00358 else { 00359 cJSON_AddNumberToObject(root, commonKW[COM_PRIORITY], priority); 00360 } 00361 } 00362 //ERROR 00363 if(error!=NULL) { 00364 if(terse_on==AX_TRUE) { 00365 cJSON_AddStringToObject(root, terse_pkgStatusKW[PKG_ERROR], error); 00366 } 00367 else { 00368 cJSON_AddStringToObject(root, pkgStatusKW[PKG_ERROR], error); 00369 } 00370 } 00371 return root; 00372 } 00373 00374
Generated on Wed Jul 13 2022 02:45:01 by
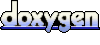