
Axeda Ready Demo for Freescale FRDM-KL46Z as accident alert system
Dependencies: FRDM_MMA8451Q KL46Z-USBHost MAG3110 SocketModem TSI mbed FATFileSystem
Fork of AxedaGo-Freescal_FRDM-KL46Z revert by
USBHost.h
00001 // Simple USBHost for FRDM-KL46Z 00002 #pragma once 00003 #include "mbed.h" 00004 #include "USBHALHost.h" 00005 #include "USBDeviceConnected.h" 00006 #include "IUSBEnumerator.h" 00007 #include "USBHostConf.h" 00008 #include "USBEndpoint.h" 00009 00010 class USBHost : public USBHALHost { 00011 public: 00012 /** 00013 * Static method to create or retrieve the single USBHost instance 00014 */ 00015 static USBHost* getHostInst(); 00016 00017 /** 00018 * Control read: setup stage, data stage and status stage 00019 * 00020 * @param dev the control read will be done for this device 00021 * @param requestType request type 00022 * @param request request 00023 * @param value value 00024 * @param index index 00025 * @param buf pointer on a buffer where will be store the data received 00026 * @param len length of the transfer 00027 * 00028 * @returns status of the control read 00029 */ 00030 USB_TYPE controlRead(USBDeviceConnected * dev, uint8_t requestType, uint8_t request, uint32_t value, uint32_t index, uint8_t * buf, uint32_t len); 00031 00032 /** 00033 * Control write: setup stage, data stage and status stage 00034 * 00035 * @param dev the control write will be done for this device 00036 * @param requestType request type 00037 * @param request request 00038 * @param value value 00039 * @param index index 00040 * @param buf pointer on a buffer which will be written 00041 * @param len length of the transfer 00042 * 00043 * @returns status of the control write 00044 */ 00045 USB_TYPE controlWrite(USBDeviceConnected * dev, uint8_t requestType, uint8_t request, uint32_t value, uint32_t index, uint8_t * buf, uint32_t len); 00046 /** 00047 * Bulk read 00048 * 00049 * @param dev the bulk transfer will be done for this device 00050 * @param ep USBEndpoint which will be used to read a packet 00051 * @param buf pointer on a buffer where will be store the data received 00052 * @param len length of the transfer 00053 * @param blocking if true, the read is blocking (wait for completion) 00054 * 00055 * @returns status of the bulk read 00056 */ 00057 USB_TYPE bulkRead(USBDeviceConnected * dev, USBEndpoint * ep, uint8_t * buf, uint32_t len, bool blocking = true); 00058 00059 /** 00060 * Bulk write 00061 * 00062 * @param dev the bulk transfer will be done for this device 00063 * @param ep USBEndpoint which will be used to write a packet 00064 * @param buf pointer on a buffer which will be written 00065 * @param len length of the transfer 00066 * @param blocking if true, the write is blocking (wait for completion) 00067 * 00068 * @returns status of the bulk write 00069 */ 00070 USB_TYPE bulkWrite(USBDeviceConnected * dev, USBEndpoint * ep, uint8_t * buf, uint32_t len, bool blocking = true); 00071 00072 /** 00073 * Interrupt read 00074 * 00075 * @param dev the interrupt transfer will be done for this device 00076 * @param ep USBEndpoint which will be used to write a packet 00077 * @param buf pointer on a buffer which will be written 00078 * @param len length of the transfer 00079 * @param blocking if true, the read is blocking (wait for completion) 00080 * 00081 * @returns status of the interrupt read 00082 */ 00083 USB_TYPE interruptRead(USBDeviceConnected * dev, USBEndpoint * ep, uint8_t * buf, uint32_t len, bool blocking = true); 00084 00085 /** 00086 * Interrupt write 00087 * 00088 * @param dev the interrupt transfer will be done for this device 00089 * @param ep USBEndpoint which will be used to write a packet 00090 * @param buf pointer on a buffer which will be written 00091 * @param len length of the transfer 00092 * @param blocking if true, the write is blocking (wait for completion) 00093 * 00094 * @returns status of the interrupt write 00095 */ 00096 USB_TYPE interruptWrite(USBDeviceConnected * dev, USBEndpoint * ep, uint8_t * buf, uint32_t len, bool blocking = true); 00097 00098 /** 00099 * Enumerate a device. 00100 * 00101 * @param dev device which will be enumerated 00102 * 00103 * @returns status of the enumeration 00104 */ 00105 USB_TYPE enumerate(USBDeviceConnected * dev, IUSBEnumerator* pEnumerator); 00106 00107 /** 00108 * Get a device 00109 * 00110 * @param index index of the device which will be returned 00111 * 00112 * @returns pointer on the "index" device 00113 */ 00114 USBDeviceConnected * getDevice(uint8_t index) { 00115 return (index < DeviceLists_count) ? DeviceLists[index] : NULL; 00116 } 00117 00118 /** 00119 * register a driver into the host associated with a callback function called when the device is disconnected 00120 * 00121 * @param dev device 00122 * @param intf interface number 00123 * @param tptr pointer to the object to call the member function on 00124 * @param mptr pointer to the member function to be called 00125 */ 00126 template<typename T> 00127 void registerDriver(USBDeviceConnected * dev, uint8_t intf, T* tptr, void (T::*mptr)(void)) { 00128 } 00129 00130 int BulkRead(USBEndpoint*ep, uint8_t* data, int size, int timeout_ms = -1); 00131 int IsochronousRead(USBEndpoint*ep, uint8_t* data, int size); 00132 00133 private: 00134 USBHost(); 00135 static USBHost* inst; 00136 virtual bool addDevice(int hub, int port, bool lowSpeed); 00137 void root_enumeration(USBDeviceConnected* dev); 00138 void parseConfDescr(USBDeviceConnected* dev, uint8_t* conf_descr, uint32_t len, IUSBEnumerator* pEnumerator); 00139 USBDeviceConnected* DeviceLists[MAX_DEVICE_CONNECTED]; 00140 int DeviceLists_count; 00141 00142 int ControlRead(USBDeviceConnected* dev, SETUP_PACKET* setup, uint8_t* data, int size); 00143 int ControlWrite(USBDeviceConnected* dev, SETUP_PACKET* setup, uint8_t* data = NULL, int size = 0); 00144 int BulkWrite(USBEndpoint*ep, const uint8_t* data, int size); 00145 int InterruptRead(USBEndpoint*ep, uint8_t* data, int size); 00146 00147 // USB HUB 00148 bool Hub(USBDeviceConnected* dev); 00149 int SetPortPower(USBDeviceConnected* dev, int port); 00150 int ClearPortPower(USBDeviceConnected* dev, int port); 00151 int PortReset(USBDeviceConnected* dev, int port); 00152 int SetPortFeature(USBDeviceConnected* dev, int feature, int index); 00153 int ClearPortFeature(USBDeviceConnected* dev, int feature, int index); 00154 int SetPortReset(USBDeviceConnected* dev, int port); 00155 int GetPortStatus(USBDeviceConnected* dev, int port, uint32_t* status); 00156 }; 00157
Generated on Wed Jul 13 2022 02:45:01 by
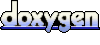