
Axeda Ready Demo for Freescale FRDM-KL46Z as accident alert system
Dependencies: FRDM_MMA8451Q KL46Z-USBHost MAG3110 SocketModem TSI mbed FATFileSystem
Fork of AxedaGo-Freescal_FRDM-KL46Z revert by
USBHostMouse.h
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "USBHost.h" 00018 00019 class USBHostMouse : public IUSBEnumerator { 00020 public: 00021 00022 /** 00023 * Constructor 00024 */ 00025 USBHostMouse(); 00026 00027 /** 00028 * Try to connect a mouse device 00029 * 00030 * @return true if connection was successful 00031 */ 00032 bool connect(); 00033 00034 /** 00035 * Check if a mouse is connected 00036 * 00037 * @returns true if a mouse is connected 00038 */ 00039 bool connected(); 00040 00041 int readReport(uint8_t* data) { 00042 int rc = host->interruptRead(dev, int_in, data, 4); 00043 return rc == USB_TYPE_OK ? 4 : 0; 00044 } 00045 void attachEvent(void (*ptr)(uint8_t* data, int size)) { 00046 if (ptr != NULL) { 00047 onUpdate = ptr; 00048 } 00049 } 00050 void poll() { 00051 USB_TYPE rc = host->interruptRead(dev, int_in, report, sizeof(report)); 00052 if (rc == USB_TYPE_OK) { 00053 if (onUpdate) { 00054 (*onUpdate)(report, int_in->getLengthTransferred()); 00055 } 00056 } 00057 } 00058 00059 protected: 00060 //From IUSBEnumerator 00061 virtual void setVidPid(uint16_t vid, uint16_t pid); 00062 virtual bool parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol); //Must return true if the interface should be parsed 00063 virtual bool useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir); //Must return true if the endpoint will be used 00064 00065 private: 00066 USBHost * host; 00067 USBDeviceConnected * dev; 00068 USBEndpoint * int_in; 00069 uint8_t report[4]; 00070 00071 bool dev_connected; 00072 bool mouse_device_found; 00073 int mouse_intf; 00074 00075 uint8_t buttons; 00076 int8_t x; 00077 int8_t y; 00078 int8_t z; 00079 00080 void (*onUpdate)(uint8_t* data, int size); 00081 void (*onButtonUpdate)(uint8_t buttons); 00082 void (*onXUpdate)(int8_t x); 00083 void (*onYUpdate)(int8_t y); 00084 void (*onZUpdate)(int8_t z); 00085 int report_id; 00086 void init(); 00087 }; 00088
Generated on Wed Jul 13 2022 02:45:01 by
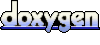