
Axeda Ready Demo for Freescale FRDM-KL46Z as accident alert system
Dependencies: FRDM_MMA8451Q KL46Z-USBHost MAG3110 SocketModem TSI mbed FATFileSystem
Fork of AxedaGo-Freescal_FRDM-KL46Z revert by
USBHostMSD.h
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef USBHOSTMSD_H 00018 #define USBHOSTMSD_H 00019 00020 #if _USB_DBG 00021 #define USB_DBG(...) do{fprintf(stderr,"[%s@%d] ",__PRETTY_FUNCTION__,__LINE__);fprintf(stderr,__VA_ARGS__);fprintf(stderr,"\r\n");} while(0); 00022 #else 00023 #define USB_DBG(...) while(0); 00024 #endif 00025 00026 #if 0 00027 #define USB_DBG2(...) do{fprintf(stderr,"[%s@%d] ",__PRETTY_FUNCTION__,__LINE__);fprintf(stderr,__VA_ARGS__);fprintf(stderr,"\r\n");} while(0); 00028 #else 00029 #define USB_DBG2(...) while(0); 00030 #endif 00031 00032 #if 1 00033 #define USB_INFO(...) do{fprintf(stderr,__VA_ARGS__);fprintf(stderr,"\r\n");}while(0); 00034 #else 00035 #define USB_INFO(...) while(0); 00036 #endif 00037 00038 #include "USBHostConf.h" 00039 #include "USBHost.h" 00040 #include "FATFileSystem.h" 00041 00042 /** 00043 * A class to communicate a USB flash disk 00044 */ 00045 class USBHostMSD : public IUSBEnumerator, public FATFileSystem { 00046 public: 00047 /** 00048 * Constructor 00049 * 00050 * @param rootdir mount name 00051 */ 00052 USBHostMSD(const char * rootdir); 00053 00054 /** 00055 * Check if a MSD device is connected 00056 * 00057 * @return true if a MSD device is connected 00058 */ 00059 bool connected(); 00060 00061 /** 00062 * Try to connect to a MSD device 00063 * 00064 * @return true if connection was successful 00065 */ 00066 bool connect(); 00067 00068 protected: 00069 //From IUSBEnumerator 00070 virtual void setVidPid(uint16_t vid, uint16_t pid); 00071 virtual bool parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol); //Must return true if the interface should be parsed 00072 virtual bool useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir); //Must return true if the endpoint will be used 00073 00074 // From FATFileSystem 00075 virtual int disk_initialize(); 00076 virtual int disk_status() {return 0;}; 00077 virtual int disk_read(uint8_t * buffer, uint64_t sector); 00078 virtual int disk_write(const uint8_t * buffer, uint64_t sector); 00079 virtual int disk_sync() {return 0;}; 00080 virtual uint64_t disk_sectors(); 00081 00082 private: 00083 USBHost * host; 00084 USBDeviceConnected * dev; 00085 bool dev_connected; 00086 USBEndpoint * bulk_in; 00087 USBEndpoint * bulk_out; 00088 uint8_t nb_ep; 00089 00090 // Bulk-only CBW 00091 typedef __packed struct { 00092 uint32_t Signature; 00093 uint32_t Tag; 00094 uint32_t DataLength; 00095 uint8_t Flags; 00096 uint8_t LUN; 00097 uint8_t CBLength; 00098 uint8_t CB[16]; 00099 } CBW; 00100 00101 // Bulk-only CSW 00102 typedef __packed struct { 00103 uint32_t Signature; 00104 uint32_t Tag; 00105 uint32_t DataResidue; 00106 uint8_t Status; 00107 } CSW; 00108 00109 CBW cbw; 00110 CSW csw; 00111 00112 int SCSITransfer(uint8_t * cmd, uint8_t cmd_len, int flags, uint8_t * data, uint32_t transfer_len); 00113 int testUnitReady(); 00114 int readCapacity(); 00115 int inquiry(uint8_t lun, uint8_t page_code); 00116 int SCSIRequestSense(); 00117 int dataTransfer(uint8_t * buf, uint32_t block, uint8_t nbBlock, int direction); 00118 int checkResult(uint8_t res, USBEndpoint * ep); 00119 int getMaxLun(); 00120 00121 int blockSize; 00122 uint64_t blockCount; 00123 00124 int msd_intf; 00125 bool msd_device_found; 00126 bool disk_init; 00127 00128 void init(); 00129 }; 00130 00131 #endif
Generated on Wed Jul 13 2022 02:45:01 by
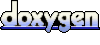