
Axeda Ready Demo for Freescale FRDM-KL46Z as accident alert system
Dependencies: FRDM_MMA8451Q KL46Z-USBHost MAG3110 SocketModem TSI mbed FATFileSystem
Fork of AxedaGo-Freescal_FRDM-KL46Z revert by
USBHostGPS.h
00001 // Simple USBHost GPS Dongle for FRDM-KL46Z 00002 #include "USBHost.h" 00003 00004 #define PL2303_SET_LINE_CODING 0x20 00005 00006 class USBHostGPS : public IUSBEnumerator { 00007 public: 00008 00009 /** 00010 * Constructor 00011 */ 00012 USBHostGPS(int baud = 38400); 00013 00014 /** 00015 * Try to connect a USB GPS device 00016 * 00017 * @return true if connection was successful 00018 */ 00019 bool connect(); 00020 00021 /** 00022 * Check if a USB GPS is connected 00023 * 00024 * @returns true if a mouse is connected 00025 */ 00026 bool connected(); 00027 00028 int readNMEA(char* data, int size, int timeout_ms) { 00029 int result = host->BulkRead(bulk_in, (uint8_t*)data, size, timeout_ms); 00030 return (result >= 0) ? bulk_in->getLengthTransferred() : 0; 00031 } 00032 void attachEvent(void (*ptr)(uint8_t* data, int size)) { 00033 if (ptr != NULL) { 00034 onUpdate = ptr; 00035 } 00036 } 00037 void poll() { 00038 int result = host->BulkRead(bulk_in, buf, sizeof(buf), 0); 00039 if (result >= 0) { 00040 if (onUpdate) { 00041 (*onUpdate)(buf, bulk_in->getLengthTransferred()); 00042 } 00043 } 00044 } 00045 00046 protected: 00047 //From IUSBEnumerator 00048 virtual void setVidPid(uint16_t vid, uint16_t pid); 00049 virtual bool parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol); //Must return true if the interface should be parsed 00050 virtual bool useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir); //Must return true if the endpoint will be used 00051 00052 private: 00053 USBHost * host; 00054 USBDeviceConnected* dev; 00055 USBEndpoint* bulk_in; 00056 bool dev_connected; 00057 bool gps_device_found; 00058 int gps_intf; 00059 void (*onUpdate)(uint8_t* data, int size); 00060 uint8_t buf[64]; 00061 int baud; 00062 void init(); 00063 };
Generated on Wed Jul 13 2022 02:45:01 by
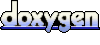