
Axeda Ready Demo for Freescale FRDM-KL46Z as accident alert system
Dependencies: FRDM_MMA8451Q KL46Z-USBHost MAG3110 SocketModem TSI mbed FATFileSystem
Fork of AxedaGo-Freescal_FRDM-KL46Z revert by
USBHostCam.cpp
00001 // USBHostCam.cpp 00002 #include "USBHostCam.h" 00003 00004 #if 0 00005 #define CAM_DBG(x, ...) std::printf("[%s:%d]"x"\n", __PRETTY_FUNCTION__, __LINE__, ##__VA_ARGS__); 00006 #else 00007 #define CAM_DBG(...) while(0); 00008 #endif 00009 #define CAM_INFO(...) do{fprintf(stderr,__VA_ARGS__);}while(0); 00010 #define USB_INFO(...) do{fprintf(stderr,__VA_ARGS__);}while(0); 00011 00012 CamInfo* getCamInfoList(); // CamInfo.cpp 00013 00014 USBHostCam::USBHostCam(uint8_t size, uint8_t option, CamInfo* user_caminfo) 00015 { 00016 CAM_DBG("size: %d, option: %d", size, option); 00017 _caminfo_size = size; 00018 _caminfo_option = option; 00019 if (user_caminfo) { 00020 CamInfoList = user_caminfo; 00021 } else { 00022 CamInfoList = getCamInfoList(); 00023 } 00024 clearOnResult(); 00025 host = USBHost::getHostInst(); 00026 dev = host->getDevice(0); 00027 ep_iso_in = new USBEndpoint; 00028 init(); 00029 } 00030 00031 void USBHostCam::init() 00032 { 00033 CAM_DBG(""); 00034 dev_connected = false; 00035 dev = NULL; 00036 cam_intf = -1; 00037 device_found = false; 00038 caminfo_found = false; 00039 } 00040 00041 bool USBHostCam::connected() 00042 { 00043 return dev_connected; 00044 } 00045 00046 bool USBHostCam::connect() 00047 { 00048 if (dev_connected) { 00049 return true; 00050 } 00051 00052 for (uint8_t i = 0; i < MAX_DEVICE_CONNECTED; i++) { 00053 if ((dev = host->getDevice(i)) != NULL) { 00054 00055 CAM_DBG("Trying to connect Cam device\r\n"); 00056 00057 if(host->enumerate(dev, this)) { 00058 break; 00059 } 00060 if (device_found) { 00061 USB_INFO("New Cam: %s device: VID:%04x PID:%04x [dev: %p - intf: %d]\n", caminfo->name, dev->getVid(), dev->getPid(), dev, cam_intf); 00062 dev->setName(caminfo->name, cam_intf); 00063 //host->registerDriver(dev, cam_intf, this, &USBHostCam::onDisconnect); 00064 int addr = dev->getAddress(); 00065 ep_iso_in->setDevice(dev); 00066 ep_iso_in->setAddress(caminfo->en); 00067 ep_iso_in->setSize(caminfo->mps); 00068 //ep_iso_in->init(addr, caminfo->en, caminfo->mps, caminfo->frameCount, caminfo->queueLimit); 00069 uint8_t buf[26]; 00070 memset(buf, 0, sizeof(buf)); 00071 buf[2] = caminfo->formatIndex; 00072 buf[3] = caminfo->frameIndex; 00073 *reinterpret_cast<uint32_t*>(buf+4) = caminfo->interval; 00074 USB_TYPE res = Control(SET_CUR, VS_COMMIT_CONTROL, 1, buf, sizeof(buf)); 00075 if (res != USB_TYPE_OK) { 00076 CAM_DBG("SET_CUR VS_COMMIT_CONTROL FAILED"); 00077 } 00078 res = setInterfaceAlternate(1, caminfo->if_alt); 00079 if (res != USB_TYPE_OK) { 00080 CAM_DBG("SET_INTERFACE FAILED"); 00081 } 00082 dev_connected = true; 00083 return true; 00084 } 00085 } 00086 } 00087 init(); 00088 return false; 00089 } 00090 00091 #if 0 00092 void USBHostCam::setup() { 00093 caminfo = CamInfoList; 00094 bool found = false; 00095 while(caminfo->vid != 0) { 00096 if (caminfo->vid == host->getDevice(0)->getVid() && 00097 caminfo->pid == host->getDevice(0)->getPid() && 00098 caminfo->size == _caminfo_size && caminfo->option == _caminfo_option) { 00099 found = true; 00100 break; 00101 } 00102 caminfo++; 00103 } 00104 if (!found) { 00105 CAM_INFO("caminfo not found.\n"); 00106 exit(1); 00107 } 00108 CAM_INFO("Found: %s\n", caminfo->name); 00109 00110 ep_iso_in.setAddress(caminfo->en); 00111 ep_iso_in.setSize(caminfo->mps); 00112 uint8_t buf[26]; 00113 memset(buf, 0, sizeof(buf)); 00114 buf[2] = caminfo->formatIndex; 00115 buf[3] = caminfo->frameIndex; 00116 *reinterpret_cast<uint32_t*>(buf+4) = caminfo->interval; 00117 USB_TYPE res = Control(SET_CUR, VS_COMMIT_CONTROL, 1, buf, sizeof(buf)); 00118 if (res != USB_TYPE_OK) { 00119 CAM_DBG("SET_CUR VS_COMMIT_CONTROL FAILED"); 00120 } 00121 res = setInterfaceAlternate(1, caminfo->if_alt); 00122 if (res != USB_TYPE_OK) { 00123 CAM_DBG("SET_INTERFACE FAILED"); 00124 } 00125 } 00126 #endif 00127 00128 00129 /*virtual*/ void USBHostCam::setVidPid(uint16_t vid, uint16_t pid) 00130 { 00131 CAM_DBG("vid:%04x,pid:%04x", vid, pid); 00132 caminfo = CamInfoList; 00133 while(caminfo->vid != 0) { 00134 if (caminfo->vid == vid && caminfo->pid == pid && 00135 caminfo->size == _caminfo_size && caminfo->option == _caminfo_option) { 00136 caminfo_found = true; 00137 break; 00138 } 00139 caminfo++; 00140 } 00141 } 00142 00143 /*virtual*/ bool USBHostCam::parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol) //Must return true if the interface should be parsed 00144 { 00145 CAM_DBG("intf_nb=%d,intf_class=%02X,intf_subclass=%d,intf_protocol=%d", intf_nb, intf_class, intf_subclass, intf_protocol); 00146 if ((cam_intf == -1) && caminfo_found) { 00147 cam_intf = intf_nb; 00148 device_found = true; 00149 return true; 00150 } 00151 return false; 00152 } 00153 00154 /*virtual*/ bool USBHostCam::useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir) //Must return true if the endpoint will be used 00155 { 00156 CAM_DBG("intf_nb:%d,type:%d,dir:%d",intf_nb, type, dir); 00157 return false; 00158 } 00159 00160 #define SEQ_READ_IDOL 0 00161 #define SEQ_READ_EXEC 1 00162 #define SEQ_READ_DONE 2 00163 00164 int USBHostCam::readJPEG(uint8_t* buf, int size, int timeout_ms) { 00165 _buf = buf; 00166 _pos = 0; 00167 _size = size; 00168 _seq = SEQ_READ_IDOL; 00169 setOnResult(this, &USBHostCam::callback_motion_jpeg); 00170 Timer timeout_t; 00171 timeout_t.reset(); 00172 timeout_t.start(); 00173 while(timeout_t.read_ms() < timeout_ms && _seq != SEQ_READ_DONE) { 00174 poll(); 00175 } 00176 return _pos; 00177 } 00178 00179 /* virtual */ void USBHostCam::outputJPEG(uint8_t c, int status) { // from decodeMJPEG 00180 if (_seq == SEQ_READ_IDOL) { 00181 if (status == JPEG_START) { 00182 _pos = 0; 00183 _seq = SEQ_READ_EXEC; 00184 } 00185 } 00186 if (_seq == SEQ_READ_EXEC) { 00187 if (_pos < _size) { 00188 _buf[_pos++] = c; 00189 } 00190 if (status == JPEG_END) { 00191 _seq = SEQ_READ_DONE; 00192 } 00193 } 00194 } 00195 00196 void USBHostCam::callback_motion_jpeg(uint16_t frame, uint8_t* buf, int len) { 00197 inputPacket(buf, len); 00198 }
Generated on Wed Jul 13 2022 02:45:01 by
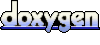