
Axeda Ready Demo for Freescale FRDM-KL46Z as accident alert system
Dependencies: FRDM_MMA8451Q KL46Z-USBHost MAG3110 SocketModem TSI mbed FATFileSystem
Fork of AxedaGo-Freescal_FRDM-KL46Z revert by
USBHALHost.h
00001 // Simple USBHost for FRDM-KL46Z 00002 #pragma once 00003 #include "mbed.h" 00004 #include "USBHostTypes.h" 00005 #include "USBEndpoint.h" 00006 00007 struct SETUP_PACKET { 00008 uint8_t bmRequestType; 00009 uint8_t bRequest; 00010 uint16_t wValue; 00011 uint16_t wIndex; 00012 uint16_t wLength; 00013 }; 00014 00015 #define GET_CONFIGURATION 8 00016 00017 // TOK_PID[5:2] 00018 #define Bus_Timeout 0x00 00019 #define Data_Error 0x0f 00020 00021 enum ODD_EVEN { 00022 ODD = 0, 00023 EVEN = 1, 00024 }; 00025 00026 class Report { 00027 public: 00028 void clear(); 00029 void print_errstat(); 00030 // error count 00031 uint32_t errstat_piderr; // USBx_ERRSTAT[PIDERR] 00032 uint32_t errstat_crc5eof;// USBx_ERRSTAT[CRC5EOF] 00033 uint32_t errstat_crc16; // USBx_ERRSTAT[CRC16] 00034 uint32_t errstat_dfn8; // USBx_ERRSTAT[DFN8] 00035 uint32_t errstat_btoerr; // USBx_ERRSTAT[BTOERR] 00036 uint32_t errstat_dmaerr; // USBx_ERRSTAT[DMAERR] 00037 uint32_t errstat_bsterr; // USBx_ERRSTAT[BTSERR] 00038 // 00039 uint32_t nak; 00040 uint32_t stall; 00041 }; 00042 00043 class USBHALHost { 00044 public: 00045 uint8_t LastStatus; 00046 uint8_t prev_LastStatus; 00047 Report report; 00048 00049 protected: 00050 USBHALHost(); 00051 void init(); 00052 virtual bool addDevice(int port, int hub, bool lowSpeed) = 0; 00053 void setAddr(int addr, bool lowSpeed = false); 00054 void setEndpoint(); 00055 void token_transfer_init(); 00056 int token_setup(USBEndpoint* ep, SETUP_PACKET* setup, uint16_t wLength = 0); 00057 int token_in(USBEndpoint* ep, uint8_t* data = NULL, int size = 0, int retryLimit = 10); 00058 int token_out(USBEndpoint* ep, const uint8_t* data = NULL, int size = 0, int retryLimit = 10); 00059 int token_iso_in(USBEndpoint* ep, uint8_t* data, int size); 00060 void token_ready(); 00061 private: 00062 static void _usbisr(void); 00063 void UsbIrqhandler(); 00064 __IO bool attach_done; 00065 __IO bool token_done; 00066 bool wait_attach(); 00067 bool root_lowSpeed; 00068 ODD_EVEN tx_ptr; 00069 ODD_EVEN rx_ptr; 00070 static USBHALHost * instHost; 00071 };
Generated on Wed Jul 13 2022 02:45:01 by
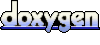