
Axeda Ready Demo for Freescale FRDM-KL46Z as accident alert system
Dependencies: FRDM_MMA8451Q KL46Z-USBHost MAG3110 SocketModem TSI mbed FATFileSystem
Fork of AxedaGo-Freescal_FRDM-KL46Z revert by
USBDeviceConnected.h
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #pragma once 00017 00018 #include "USBEndpoint.h" 00019 #include "USBHostConf.h" 00020 00021 class USBEndpoint; 00022 00023 typedef struct { 00024 bool in_use; 00025 uint8_t nb_endpoint; 00026 uint8_t intf_class; 00027 uint8_t intf_subclass; 00028 uint8_t intf_protocol; 00029 USBEndpoint * ep[MAX_ENDPOINT_PER_INTERFACE]; 00030 //FunctionPointer detach; 00031 //char name[10]; 00032 } INTERFACE; 00033 00034 /** 00035 * USBDeviceConnected class 00036 */ 00037 class USBDeviceConnected { 00038 public: 00039 00040 /** 00041 * Constructor 00042 */ 00043 USBDeviceConnected(); 00044 00045 /** 00046 * Attach an USBEndpoint to this device 00047 * 00048 * @param intf_nb interface number 00049 * @param ep pointeur on the USBEndpoint which will be attached 00050 * @returns true if successful, false otherwise 00051 */ 00052 bool addEndpoint(uint8_t intf_nb, USBEndpoint * ep); 00053 00054 /** 00055 * Retrieve an USBEndpoint by its TYPE and DIRECTION 00056 * 00057 * @param intf_nb the interface on which to lookup the USBEndpoint 00058 * @param type type of the USBEndpoint looked for 00059 * @param dir direction of the USBEndpoint looked for 00060 * @param index the index of the USBEndpoint whitin the interface 00061 * @returns pointer on the USBEndpoint if found, NULL otherwise 00062 */ 00063 USBEndpoint * getEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir, uint8_t index = 0); 00064 00065 /** 00066 * Retrieve an USBEndpoint by its index 00067 * 00068 * @param intf_nb interface number 00069 * @param index index of the USBEndpoint 00070 * @returns pointer on the USBEndpoint if found, NULL otherwise 00071 */ 00072 USBEndpoint * getEndpoint(uint8_t intf_nb, uint8_t index); 00073 00074 /** 00075 * Add a new interface to this device 00076 * 00077 * @param intf_nb interface number 00078 * @param intf_class interface class 00079 * @param intf_subclass interface subclass 00080 * @param intf_protocol interface protocol 00081 * @returns true if successful, false otherwise 00082 */ 00083 bool addInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol); 00084 00085 /** 00086 * Get a specific interface 00087 * 00088 * @param index index of the interface to be fetched 00089 * @returns interface 00090 */ 00091 INTERFACE * getInterface(uint8_t index); 00092 00093 /** 00094 * Disconnect the device by calling a callback function registered by a driver 00095 */ 00096 void disconnect(); 00097 00098 void init(uint8_t hub, uint8_t _port, bool _lowSpeed); 00099 void setAddress(uint8_t addr_) { addr = addr_; }; 00100 void setVid(uint16_t vid_) { vid = vid_; }; 00101 void setPid(uint16_t pid_) { pid = pid_; }; 00102 void setClass(uint8_t device_class_) { device_class = device_class_; } 00103 void setSubClass(uint8_t device_subclass_) { device_subclass = device_subclass_; }; 00104 void setProtocol(uint8_t pr) { proto = pr; }; 00105 void setEnumerated() { enumerated = true; }; 00106 void setNbIntf(uint8_t nb_intf) {nb_interf = nb_intf; }; 00107 void setSpeed(bool _lowSpeed) { lowSpeed = _lowSpeed; } 00108 void setName(const char * name_, uint8_t intf_nb) { return; }; 00109 void setEpCtl(USBEndpoint* ep) { ep_ctl = ep; } 00110 00111 static int getNewAddress() { 00112 static int addr = 1; 00113 return addr++; 00114 } 00115 uint8_t getHub() { return hub_nb; }; 00116 uint8_t getAddress() { return addr; }; 00117 uint16_t getVid() { return vid; }; 00118 uint16_t getPid() { return pid; }; 00119 uint8_t getClass() { return device_class; }; 00120 bool getSpeed() { return lowSpeed; } 00121 bool isEnumerated() { return enumerated; }; 00122 USBEndpoint* getEpCtl() { return ep_ctl; } 00123 00124 private: 00125 INTERFACE intf[MAX_INTF]; 00126 uint8_t hub_nb; 00127 uint8_t port; 00128 uint16_t vid; 00129 uint16_t pid; 00130 uint8_t addr; 00131 uint8_t device_class; 00132 uint8_t device_subclass; 00133 uint8_t proto; 00134 bool lowSpeed; 00135 bool enumerated; 00136 uint8_t nb_interf; 00137 USBEndpoint* ep_ctl; 00138 void init(); 00139 };
Generated on Wed Jul 13 2022 02:45:01 by
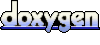