
Axeda Ready Demo for Freescale FRDM-KL46Z as accident alert system
Dependencies: FRDM_MMA8451Q KL46Z-USBHost MAG3110 SocketModem TSI mbed FATFileSystem
Fork of AxedaGo-Freescal_FRDM-KL46Z revert by
USBDeviceConnected.cpp
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "USBDeviceConnected.h" 00018 //#include "dbg.h" 00019 #define USB_DBG(...) while(0) 00020 00021 USBDeviceConnected::USBDeviceConnected() { 00022 init(); 00023 } 00024 00025 void USBDeviceConnected::init() { 00026 hub_nb = 0; 00027 port = 0; 00028 vid = 0; 00029 pid = 0; 00030 nb_interf = 0; 00031 enumerated = false; 00032 //activeAddr = false; 00033 //sizeControlEndpoint = 8; 00034 device_class = 0; 00035 device_subclass = 0; 00036 proto = 0; 00037 lowSpeed = false; 00038 for (int i = 0; i < MAX_INTF; i++) { 00039 memset((void *)&intf[i], 0, sizeof(INTERFACE)); 00040 intf[i].in_use = false; 00041 for (int j = 0; j < MAX_ENDPOINT_PER_INTERFACE; j++) { 00042 intf[i].ep[j] = NULL; 00043 //strcpy(intf[i].name, "Unknown"); 00044 } 00045 } 00046 //hub_parent = NULL; 00047 //hub = NULL; 00048 nb_interf = 0; 00049 } 00050 00051 INTERFACE * USBDeviceConnected::getInterface(uint8_t index) { 00052 if (index >= MAX_INTF) 00053 return NULL; 00054 00055 if (intf[index].in_use) 00056 return &intf[index]; 00057 00058 return NULL; 00059 } 00060 00061 bool USBDeviceConnected::addInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol) { 00062 if ((intf_nb >= MAX_INTF) || (intf[intf_nb].in_use)) { 00063 return false; 00064 } 00065 intf[intf_nb].in_use = true; 00066 intf[intf_nb].intf_class = intf_class; 00067 intf[intf_nb].intf_subclass = intf_subclass; 00068 intf[intf_nb].intf_protocol = intf_protocol; 00069 intf[intf_nb].nb_endpoint = 0; 00070 return true; 00071 } 00072 00073 bool USBDeviceConnected::addEndpoint(uint8_t intf_nb, USBEndpoint * ept) { 00074 if ((intf_nb >= MAX_INTF) || (intf[intf_nb].in_use == false) || (intf[intf_nb].nb_endpoint >= MAX_ENDPOINT_PER_INTERFACE)) { 00075 return false; 00076 } 00077 intf[intf_nb].nb_endpoint++; 00078 00079 for (int i = 0; i < MAX_ENDPOINT_PER_INTERFACE; i++) { 00080 if (intf[intf_nb].ep[i] == NULL) { 00081 intf[intf_nb].ep[i] = ept; 00082 return true; 00083 } 00084 } 00085 return false; 00086 } 00087 00088 void USBDeviceConnected::init(uint8_t hub_, uint8_t port_, bool lowSpeed_) { 00089 USB_DBG("init dev: %p", this); 00090 init(); 00091 hub_nb = hub_; 00092 port = port_; 00093 lowSpeed = lowSpeed_; 00094 } 00095 00096 void USBDeviceConnected::disconnect() { 00097 //for(int i = 0; i < MAX_INTF; i++) { 00098 // intf[i].detach.call(); 00099 //} 00100 //init(); 00101 } 00102 00103 00104 USBEndpoint * USBDeviceConnected::getEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir, uint8_t index) { 00105 if (intf_nb >= MAX_INTF) { 00106 return NULL; 00107 } 00108 for (int i = 0; i < MAX_ENDPOINT_PER_INTERFACE; i++) { 00109 if ((intf[intf_nb].ep[i]->getType() == type) && (intf[intf_nb].ep[i]->getDir() == dir)) { 00110 if(index) { 00111 index--; 00112 } else { 00113 return intf[intf_nb].ep[i]; 00114 } 00115 } 00116 } 00117 return NULL; 00118 } 00119 00120 USBEndpoint * USBDeviceConnected::getEndpoint(uint8_t intf_nb, uint8_t index) { 00121 if ((intf_nb >= MAX_INTF) || (index >= MAX_ENDPOINT_PER_INTERFACE)) { 00122 return NULL; 00123 } 00124 return intf[intf_nb].ep[index]; 00125 }
Generated on Wed Jul 13 2022 02:45:01 by
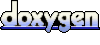