Should be no changes
Fork of mbed-dev-bin by
Embed:
(wiki syntax)
Show/hide line numbers
CAN.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_CAN_H 00017 #define MBED_CAN_H 00018 00019 #include "platform.h" 00020 00021 #if DEVICE_CAN 00022 00023 #include "can_api.h" 00024 #include "can_helper.h" 00025 #include "FunctionPointer.h" 00026 00027 namespace mbed { 00028 00029 /** CANMessage class 00030 */ 00031 class CANMessage : public CAN_Message { 00032 00033 public: 00034 /** Creates empty CAN message. 00035 */ 00036 CANMessage() : CAN_Message() { 00037 len = 8; 00038 type = CANData; 00039 format = CANStandard; 00040 id = 0; 00041 memset(data, 0, 8); 00042 } 00043 00044 /** Creates CAN message with specific content. 00045 */ 00046 CANMessage(int _id, const char *_data, char _len = 8, CANType _type = CANData, CANFormat _format = CANStandard) { 00047 len = _len & 0xF; 00048 type = _type; 00049 format = _format; 00050 id = _id; 00051 memcpy(data, _data, _len); 00052 } 00053 00054 /** Creates CAN remote message. 00055 */ 00056 CANMessage(int _id, CANFormat _format = CANStandard) { 00057 len = 0; 00058 type = CANRemote; 00059 format = _format; 00060 id = _id; 00061 memset(data, 0, 8); 00062 } 00063 }; 00064 00065 /** A can bus client, used for communicating with can devices 00066 */ 00067 class CAN { 00068 00069 public: 00070 /** Creates an CAN interface connected to specific pins. 00071 * 00072 * @param rd read from transmitter 00073 * @param td transmit to transmitter 00074 * 00075 * Example: 00076 * @code 00077 * #include "mbed.h" 00078 * 00079 * Ticker ticker; 00080 * DigitalOut led1(LED1); 00081 * DigitalOut led2(LED2); 00082 * CAN can1(p9, p10); 00083 * CAN can2(p30, p29); 00084 * 00085 * char counter = 0; 00086 * 00087 * void send() { 00088 * if(can1.write(CANMessage(1337, &counter, 1))) { 00089 * printf("Message sent: %d\n", counter); 00090 * counter++; 00091 * } 00092 * led1 = !led1; 00093 * } 00094 * 00095 * int main() { 00096 * ticker.attach(&send, 1); 00097 * CANMessage msg; 00098 * while(1) { 00099 * if(can2.read(msg)) { 00100 * printf("Message received: %d\n\n", msg.data[0]); 00101 * led2 = !led2; 00102 * } 00103 * wait(0.2); 00104 * } 00105 * } 00106 * @endcode 00107 */ 00108 CAN(PinName rd, PinName td); 00109 virtual ~CAN(); 00110 00111 /** Set the frequency of the CAN interface 00112 * 00113 * @param hz The bus frequency in hertz 00114 * 00115 * @returns 00116 * 1 if successful, 00117 * 0 otherwise 00118 */ 00119 int frequency(int hz); 00120 00121 /** Write a CANMessage to the bus. 00122 * 00123 * @param msg The CANMessage to write. 00124 * 00125 * @returns 00126 * 0 if write failed, 00127 * 1 if write was successful 00128 */ 00129 int write(CANMessage msg); 00130 00131 /** Read a CANMessage from the bus. 00132 * 00133 * @param msg A CANMessage to read to. 00134 * @param handle message filter handle (0 for any message) 00135 * 00136 * @returns 00137 * 0 if no message arrived, 00138 * 1 if message arrived 00139 */ 00140 int read(CANMessage &msg, int handle = 0); 00141 00142 /** Reset CAN interface. 00143 * 00144 * To use after error overflow. 00145 */ 00146 void reset(); 00147 00148 /** Puts or removes the CAN interface into silent monitoring mode 00149 * 00150 * @param silent boolean indicating whether to go into silent mode or not 00151 */ 00152 void monitor(bool silent); 00153 00154 enum Mode { 00155 Reset = 0, 00156 Normal, 00157 Silent, 00158 LocalTest, 00159 GlobalTest, 00160 SilentTest 00161 }; 00162 00163 /** Change CAN operation to the specified mode 00164 * 00165 * @param mode The new operation mode (CAN::Normal, CAN::Silent, CAN::LocalTest, CAN::GlobalTest, CAN::SilentTest) 00166 * 00167 * @returns 00168 * 0 if mode change failed or unsupported, 00169 * 1 if mode change was successful 00170 */ 00171 int mode(Mode mode); 00172 00173 /** Filter out incomming messages 00174 * 00175 * @param id the id to filter on 00176 * @param mask the mask applied to the id 00177 * @param format format to filter on (Default CANAny) 00178 * @param handle message filter handle (Optional) 00179 * 00180 * @returns 00181 * 0 if filter change failed or unsupported, 00182 * new filter handle if successful 00183 */ 00184 int filter(unsigned int id, unsigned int mask, CANFormat format = CANAny, int handle = 0); 00185 00186 /** Returns number of read errors to detect read overflow errors. 00187 */ 00188 unsigned char rderror(); 00189 00190 /** Returns number of write errors to detect write overflow errors. 00191 */ 00192 unsigned char tderror(); 00193 00194 enum IrqType { 00195 RxIrq = 0, 00196 TxIrq, 00197 EwIrq, 00198 DoIrq, 00199 WuIrq, 00200 EpIrq, 00201 AlIrq, 00202 BeIrq, 00203 IdIrq 00204 }; 00205 00206 /** Attach a function to call whenever a CAN frame received interrupt is 00207 * generated. 00208 * 00209 * @param fptr A pointer to a void function, or 0 to set as none 00210 * @param event Which CAN interrupt to attach the member function to (CAN::RxIrq for message received, CAN::TxIrq for transmitted or aborted, CAN::EwIrq for error warning, CAN::DoIrq for data overrun, CAN::WuIrq for wake-up, CAN::EpIrq for error passive, CAN::AlIrq for arbitration lost, CAN::BeIrq for bus error) 00211 */ 00212 void attach(void (*fptr)(void), IrqType type=RxIrq); 00213 00214 /** Attach a member function to call whenever a CAN frame received interrupt 00215 * is generated. 00216 * 00217 * @param tptr pointer to the object to call the member function on 00218 * @param mptr pointer to the member function to be called 00219 * @param event Which CAN interrupt to attach the member function to (CAN::RxIrq for message received, TxIrq for transmitted or aborted, EwIrq for error warning, DoIrq for data overrun, WuIrq for wake-up, EpIrq for error passive, AlIrq for arbitration lost, BeIrq for bus error) 00220 */ 00221 template<typename T> 00222 void attach(T* tptr, void (T::*mptr)(void), IrqType type=RxIrq) { 00223 if((mptr != NULL) && (tptr != NULL)) { 00224 _irq[type].attach(tptr, mptr); 00225 can_irq_set(&_can, (CanIrqType)type, 1); 00226 } 00227 else { 00228 can_irq_set(&_can, (CanIrqType)type, 0); 00229 } 00230 } 00231 00232 static void _irq_handler(uint32_t id, CanIrqType type); 00233 00234 protected: 00235 can_t _can; 00236 FunctionPointer _irq[9]; 00237 }; 00238 00239 } // namespace mbed 00240 00241 #endif 00242 00243 #endif // MBED_CAN_H
Generated on Tue Jul 12 2022 19:13:19 by
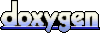