Comms Library for communicating via bluetooth
Embed:
(wiki syntax)
Show/hide line numbers
Comms.h
00001 /* Comms Library for communicating with the bluetooth car. v1.0 00002 * Copyright (c) 2017 Armand Coetzer 00003 * s213293048@nmmu.ac.za 00004 * 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in 00014 * all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00022 * THE SOFTWARE. 00023 */ 00024 00025 #ifndef Comms_H 00026 #define Comms_H 00027 00028 #include "mbed.h" 00029 00030 /** Class library to create a serial connection from the MCU to the bluetooth device. 00031 * 00032 * Example: 00033 * @code 00034 * #include "mbed.h" 00035 *#include "Comms.h" 00036 * 00037 *Comms BT(PC_6, PC_7); 00038 * 00039 *int main() 00040 *{ 00041 * while(1) 00042 * { 00043 * if(BT.newmsg == true) 00044 * { 00045 * BT.printf("%s\n",BT.cmd); 00046 * BT. printf("%.2f\n", BT.data); 00047 * BT.clrmsg(); 00048 * } 00049 * 00050 * } 00051 *} 00052 * @endcode 00053 */ 00054 00055 class Comms : public RawSerial{ 00056 public: 00057 /** Create a serial connection from the MCU to the bluetooth device. 00058 * @param TX Pin for transmitting data. 00059 * @param RX Pin for Receiving data. 00060 */ 00061 Comms(PinName TX, PinName RX); 00062 00063 // /** changing the boolen variable to false. 00064 // * @param 00065 // * None 00066 // */ 00067 // void clrmsg(); 00068 00069 /** float variable for storing the speed value. 00070 * @param 00071 * None 00072 */ 00073 float data; 00074 00075 /** Character string for storing the direction. 00076 * @param 00077 * None 00078 */ 00079 char cmd[50]; 00080 00081 /** boolean variable to state if there is a new message or not. 00082 * @param 00083 * None 00084 */ 00085 bool newmsg; 00086 00087 00088 private: 00089 00090 void commsinterrupt(); 00091 00092 char msg[50]; //incoming message string 00093 int msg_counter; //character position counter in the message string 00094 00095 }; 00096 00097 #endif
Generated on Sun Aug 7 2022 14:32:00 by
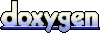