Comms Library for communicating via bluetooth
Embed:
(wiki syntax)
Show/hide line numbers
Comms.cpp
00001 #include "Comms.h" 00002 #include "mbed.h" 00003 00004 Comms::Comms(PinName TX, PinName RX) : RawSerial(TX, RX) 00005 { 00006 msg_counter = 0; 00007 newmsg = false; 00008 00009 attach(this, &Comms::commsinterrupt); 00010 } 00011 00012 void Comms::commsinterrupt() 00013 { 00014 char c = getc(); //read the incoming character 00015 00016 if(c == '!') 00017 { 00018 msg_counter = 0; 00019 } 00020 else if(c == '#') 00021 { 00022 msg[msg_counter] = '\0'; 00023 newmsg = true; //enable the new message flag to indicate a COMPLETE message was received 00024 msg_counter = 0; //clear the message string 00025 } 00026 else 00027 { 00028 msg[msg_counter] = c; //add the character to the message string 00029 msg_counter++; //move to the next character in the string 00030 } 00031 00032 if(newmsg == true) 00033 { 00034 sscanf(msg,"%s %f", &cmd, &data); 00035 newmsg = false; 00036 } 00037 } 00038 00039
Generated on Sun Aug 7 2022 14:32:00 by
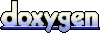