Library I2C pins modification to fit nucleo 32 pins modules
Dependencies: ST_INTERFACES X_NUCLEO_COMMON
Dependents: STM32_MagneticLight
Fork of X_NUCLEO_IKS01A1 by
hts221_class.h
00001 /** 00002 ****************************************************************************** 00003 * @file hts221_class.h 00004 * @author AST / EST 00005 * @version V0.0.1 00006 * @date 14-April-2015 00007 * @brief Header file for component HTS221 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 #ifndef __HTS221_CLASS_H 00039 #define __HTS221_CLASS_H 00040 00041 /* Includes ------------------------------------------------------------------*/ 00042 #include "mbed.h" 00043 #include "DevI2C.h" 00044 #include "hts221.h" 00045 #include "HumiditySensor.h" 00046 #include "TempSensor.h" 00047 00048 /* Classes -------------------------------------------------------------------*/ 00049 /** Class representing a HTS221 sensor component 00050 */ 00051 class HTS221 : public HumiditySensor, public TempSensor { 00052 public: 00053 /** Constructor 00054 * @param[in] i2c device I2C to be used for communication 00055 */ 00056 HTS221(DevI2C &i2c) : HumiditySensor(), TempSensor(), dev_i2c(i2c) { 00057 T0_degC = T1_degC = H0_rh = H1_rh = 0.0; 00058 T0_out = T1_out = H0_T0_out = H1_T0_out = 0; 00059 } 00060 00061 /** Destructor 00062 */ 00063 virtual ~HTS221() {} 00064 00065 /*** Interface Methods ***/ 00066 virtual int Init(void *init_struct) { 00067 return HTS221_Init((HUM_TEMP_InitTypeDef*)init_struct); 00068 } 00069 00070 /** 00071 * @brief Enter sensor shutdown mode 00072 * @return 0 in case of success, an error code otherwise 00073 */ 00074 virtual int PowerOff(void) { 00075 return HTS221_Power_OFF(); 00076 } 00077 00078 virtual int ReadID(uint8_t *ht_id) { 00079 return HTS221_ReadID(ht_id); 00080 } 00081 00082 /** 00083 * @brief Reset sensor 00084 * @return 0 in case of success, an error code otherwise 00085 */ 00086 virtual int Reset(void) { 00087 return HTS221_RebootCmd(); 00088 } 00089 00090 virtual int GetHumidity(float *pfData) { 00091 return HTS221_GetHumidity(pfData); 00092 } 00093 00094 virtual int GetTemperature(float *pfData) { 00095 return HTS221_GetTemperature(pfData); 00096 } 00097 00098 protected: 00099 /*** Methods ***/ 00100 HUM_TEMP_StatusTypeDef HTS221_Init(HUM_TEMP_InitTypeDef *HTS221_Init); 00101 HUM_TEMP_StatusTypeDef HTS221_Power_OFF(void); 00102 HUM_TEMP_StatusTypeDef HTS221_ReadID(uint8_t *ht_id); 00103 HUM_TEMP_StatusTypeDef HTS221_RebootCmd(void); 00104 HUM_TEMP_StatusTypeDef HTS221_GetHumidity(float* pfData); 00105 HUM_TEMP_StatusTypeDef HTS221_GetTemperature(float* pfData); 00106 00107 HUM_TEMP_StatusTypeDef HTS221_Power_On(void); 00108 HUM_TEMP_StatusTypeDef HTS221_Calibration(void); 00109 00110 /** 00111 * @brief Configures HTS221 interrupt lines for NUCLEO boards 00112 */ 00113 void HTS221_IO_ITConfig(void) 00114 { 00115 /* To be implemented */ 00116 } 00117 00118 /** 00119 * @brief Configures HTS221 I2C interface 00120 * @return HUM_TEMP_OK in case of success, an error code otherwise 00121 */ 00122 HUM_TEMP_StatusTypeDef HTS221_IO_Init(void) 00123 { 00124 return HUM_TEMP_OK; /* done in constructor */ 00125 } 00126 00127 /** 00128 * @brief Utility function to read data from HTS221 00129 * @param[out] pBuffer pointer to the byte-array to read data in to 00130 * @param[in] RegisterAddr specifies internal address register to read from. 00131 * @param[in] NumByteToRead number of bytes to be read. 00132 * @retval HUM_TEMP_OK if ok, 00133 * @retval HUM_TEMP_ERROR if an I2C error has occured 00134 */ 00135 HUM_TEMP_StatusTypeDef HTS221_IO_Read(uint8_t* pBuffer, 00136 uint8_t RegisterAddr, uint16_t NumByteToRead) 00137 { 00138 int ret = dev_i2c.i2c_read(pBuffer, 00139 HTS221_ADDRESS, 00140 RegisterAddr, 00141 NumByteToRead); 00142 if(ret != 0) { 00143 return HUM_TEMP_ERROR; 00144 } 00145 return HUM_TEMP_OK; 00146 } 00147 00148 /** 00149 * @brief Utility function to write data to HTS221 00150 * @param[in] pBuffer pointer to the byte-array data to send 00151 * @param[in] RegisterAddr specifies internal address register to read from. 00152 * @param[in] NumByteToWrite number of bytes to write. 00153 * @retval HUM_TEMP_OK if ok, 00154 * @retval HUM_TEMP_ERROR if an I2C error has occured 00155 */ 00156 HUM_TEMP_StatusTypeDef HTS221_IO_Write(uint8_t* pBuffer, 00157 uint8_t RegisterAddr, uint16_t NumByteToWrite) 00158 { 00159 int ret = dev_i2c.i2c_write(pBuffer, 00160 HTS221_ADDRESS, 00161 RegisterAddr, 00162 NumByteToWrite); 00163 if(ret != 0) { 00164 return HUM_TEMP_ERROR; 00165 } 00166 return HUM_TEMP_OK; 00167 } 00168 00169 /*** Instance Variables ***/ 00170 /* IO Device */ 00171 DevI2C &dev_i2c; 00172 00173 /* Temperature in degree for calibration */ 00174 float T0_degC, T1_degC; 00175 00176 /* Output temperature value for calibration */ 00177 int16_t T0_out, T1_out; 00178 00179 /* Humidity for calibration */ 00180 float H0_rh, H1_rh; 00181 00182 /* Output Humidity value for calibration */ 00183 int16_t H0_T0_out, H1_T0_out; 00184 }; 00185 00186 #endif // __HTS221_CLASS_H
Generated on Wed Jul 13 2022 03:04:47 by
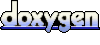