Platform library for RETRO
Embed:
(wiki syntax)
Show/hide line numbers
Retro.h
00001 /* 00002 * (C) Copyright 2015 Valentin Ivanov. All rights reserved. 00003 * 00004 * This file is part of the RetroPlatform Library 00005 * 00006 * The RetroPlatform Library is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU Lesser General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * This program is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU Lesser General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU Lesser General Public License 00017 * along with this program. If not, see <http://www.gnu.org/licenses/> 00018 * 00019 * This library is inspired by Gamebuino Library (http://gamebuino.com) 00020 * from Aurélien Rodot. 00021 */ 00022 #ifndef __RETRO_H__ 00023 #define __RETRO_H__ 00024 00025 #include "mbed.h" 00026 #include "Sound.h" 00027 #include "Display.h" 00028 #include "Color565.h" 00029 00030 #define LCDWIDTH 160 00031 #define LCDHEIGHT 128 00032 00033 #define BTN_LEFT 0 00034 #define BTN_RIGHT 1 00035 #define BTN_DOWN 2 00036 #define BTN_UP 3 00037 #define BTN_ROBOT 4 00038 #define BTN_SHIP 5 00039 00040 #define NUM_BTN 6 00041 00042 class Retro 00043 { 00044 private: 00045 00046 uint32_t frameStartUs, frameEndUs; 00047 00048 private: 00049 static DigitalIn pin[NUM_BTN]; 00050 uint8_t _state[NUM_BTN]; 00051 00052 public: 00053 uint32_t timePerFrame; 00054 uint32_t frameDurationUs; 00055 00056 Sound sound; 00057 Display display; 00058 00059 DigitalOut leftEye; 00060 DigitalOut rightEye; 00061 00062 public: 00063 Retro(); 00064 void initialize(); 00065 bool update(); 00066 00067 void setFrameRate(uint8_t fps); 00068 static bool collideCheck( int x1, int y1, int w1, int h1, int x2, int y2, int w2, int h2); 00069 00070 void playOK(); 00071 void playCancel(); 00072 void playTick(); 00073 00074 void readButtons(); 00075 bool pressed(uint8_t button); 00076 bool released(uint8_t button); 00077 bool held(uint8_t button, uint8_t time); 00078 bool repeat(uint8_t button, uint8_t period); 00079 uint8_t timeHeld(uint8_t button); 00080 00081 }; 00082 00083 00084 uint8_t max(uint8_t val1, uint8_t val2 ); 00085 uint8_t min(uint8_t val1, uint8_t val2 ); 00086 00087 #endif //__RETRO_H__
Generated on Tue Jul 12 2022 23:21:39 by
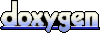