AppNearMe µNFC stack for the NXP PN532 chip License: You can use the stack free of charge to prototype with mbed; if you want to use the stack with your commercial product, get in touch!
Dependents: IOT_sensor_nfc AppNearMe_MuNFC_PN532_Test p2p_nfc_test NFCMoodLamp ... more
TLVList.h
00001 /* 00002 TLVList.h 00003 Copyright (c) Donatien Garnier 2012 00004 donatien.garnier@appnearme.com 00005 http://www.appnearme.com/ 00006 */ 00007 00008 /** \file TLVList.h 00009 * List of Type/Length/Values items 00010 */ 00011 00012 #ifndef TLVLIST_H_ 00013 #define TLVLIST_H_ 00014 00015 #include <cstdint> //For uint_*t 00016 #include <cstring> //For size_t 00017 00018 using std::uint8_t; 00019 using std::uint16_t; 00020 using std::uint32_t; 00021 using std::size_t; 00022 00023 typedef struct __tlv_list tlv_list; 00024 00025 /** A simple container 00026 * List of Type/Length/Values items 00027 */ 00028 class TLVList 00029 { 00030 public: 00031 00032 //getNext... 00033 //get() 00034 //put() 00035 00036 00037 //typedef tlv_type TLVType; 00038 /** Type of a TLV item 00039 * 00040 */ 00041 enum TLVType 00042 { 00043 UINT8, //!< Unsigned char (byte) 00044 UINT32, //!< Unsigned int 00045 UINT8_ARRAY, ///< Byte array 00046 STRING, //!<String 00047 NONE, //!<End of packet 00048 UNKNOWN //!< Unknown type 00049 }; 00050 00051 /* Getters */ 00052 00053 /** Iterate to next item. 00054 * @return type if next item exists, NONE otherwise 00055 */ 00056 TLVType getNext(); 00057 00058 /** Get current item's type. 00059 * @return type if item exists, NONE otherwise 00060 */ 00061 TLVType getType(); 00062 00063 /** Get uint8_t value. 00064 * If the current item's type is uint32_t, the value will be masked with 0xFF. 00065 * @return uint8_t value OR 0 if the type is incompatible 00066 */ 00067 uint8_t getUInt8(); 00068 00069 /** Get uint32_t value. 00070 * If the current item's type is uint8_t, the value will be casted to uint32_t. 00071 * @return uint32_t value OR 0 if the type is incompatible 00072 */ 00073 uint32_t getUInt32(); 00074 00075 /** Get array length. 00076 * @return bytes array length 00077 */ 00078 size_t getArrayLength(); 00079 00080 /** Get array. 00081 * @param buf pointer to buffer's start 00082 * @param maxLen maximum number of bytes to copy 00083 * @return number of copied bytes 00084 */ 00085 size_t getArray(uint8_t* buf, size_t maxLen); 00086 00087 /** Get string length. 00088 * @return string length 00089 */ 00090 size_t getStringLength(); 00091 00092 /** Get string. 00093 * Copy string to buffer (including null-terminating char). 00094 * @param str pointer to string's start 00095 * @param maxLen maximum number of chars to copy (not including null-terminating char) 00096 * @return number of copied chars 00097 */ 00098 size_t getString(char* str, size_t maxLen); 00099 00100 /* Setters */ 00101 00102 /** Check whether there is space left in list. 00103 * @return true if there is space left, false otherwise 00104 */ 00105 bool isSpace(); 00106 00107 /** Put uint8_t value. 00108 * @param value uint8_t value 00109 * @return true on success, false if there is not enough space in buffer 00110 */ 00111 bool putUInt8(uint8_t value); 00112 00113 /** Put uint32_t value. 00114 * @param value uint32_t value 00115 * @return true on success, false if there is not enough space in buffer 00116 */ 00117 bool putUInt32(uint32_t value); 00118 00119 /** Put array. 00120 * @param buf pointer to buffer's start 00121 * @param len number of bytes to copy 00122 * @return number of copied bytes 00123 */ 00124 size_t putArray(uint8_t* buf, size_t len); 00125 00126 /** Put string. 00127 * @param str pointer to null-terminated string's start 00128 * @return number of copied bytes 00129 */ 00130 size_t putString(char* str); 00131 00132 protected: 00133 TLVList(); 00134 00135 void wrap(tlv_list* payload); 00136 00137 private: 00138 tlv_list* m_tlvList; 00139 00140 TLVType m_type; 00141 00142 union 00143 { 00144 uint8_t m_uint8; 00145 uint32_t m_uint32; 00146 uint8_t* m_array; 00147 char* m_str; 00148 }; 00149 00150 union 00151 { 00152 size_t m_arrayLen; 00153 size_t m_strLen; 00154 }; 00155 00156 bool m_space; 00157 00158 friend class NdefCallback; 00159 }; 00160 00161 00162 #endif /* TLVLIST_H_ */
Generated on Tue Jul 12 2022 17:28:36 by
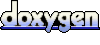