AppNearMe µNFC stack for the NXP PN532 chip License: You can use the stack free of charge to prototype with mbed; if you want to use the stack with your commercial product, get in touch!
Dependents: IOT_sensor_nfc AppNearMe_MuNFC_PN532_Test p2p_nfc_test NFCMoodLamp ... more
TLVList.cpp
00001 /* 00002 TLVList.cpp 00003 Copyright (c) Donatien Garnier 2012 00004 donatien.garnier@appnearme.com 00005 http://www.appnearme.com/ 00006 */ 00007 00008 #include "TLVList.h" 00009 00010 #include "munfc/core/fwk.h" 00011 #include "munfc/ndef/appnearme_ndef_tlv.h" 00012 00013 #include <cstring> //For memcpy, strlen 00014 using std::memcpy; 00015 using std::strlen; 00016 00017 TLVList::TLVList() : m_tlvList(NULL) 00018 { 00019 00020 } 00021 00022 void TLVList::wrap(tlv_list* payload) 00023 { 00024 m_tlvList = payload; 00025 m_space = true; 00026 m_type = NONE; 00027 } 00028 00029 00030 00031 /* Getters */ 00032 00033 /** Iterate to next item 00034 * @return type if next item exists, NONE otherwise 00035 */ 00036 TLVList::TLVType TLVList::getNext() 00037 { 00038 if(!m_tlvList) 00039 { 00040 return NONE; 00041 } 00042 00043 m_type = (TLVList::TLVType) appnearme_ndef_tlv_next_type(m_tlvList); //Explicit cast, this is the same enum 00044 switch(m_type) 00045 { 00046 case UINT8: 00047 m_arrayLen = 0; 00048 m_uint8 = appnearme_ndef_tlv_get_uint8(m_tlvList); 00049 break; 00050 case UINT32: 00051 m_arrayLen = 0; 00052 m_uint32 = appnearme_ndef_tlv_get_uint32(m_tlvList); 00053 break; 00054 case UINT8_ARRAY: 00055 //In this order, otherwise would break state-machine 00056 m_arrayLen = appnearme_ndef_tlv_get_array_length(m_tlvList); 00057 m_array = appnearme_ndef_tlv_get_array(m_tlvList); 00058 break; 00059 case STRING: 00060 //In this order, otherwise would break state-machine 00061 m_strLen = appnearme_ndef_tlv_get_string_length(m_tlvList); 00062 m_str = appnearme_ndef_tlv_get_string(m_tlvList); 00063 break; 00064 } 00065 return m_type; 00066 } 00067 00068 /** Get current item's type. 00069 * @return type if item exists, NONE otherwise 00070 */ 00071 TLVList::TLVType TLVList::getType() 00072 { 00073 return m_type; 00074 } 00075 00076 /** Get uint8_t value 00077 * If the current item's type is uint32_t, the value will be masked with 0xFF 00078 * @return uint8_t value OR 0 if the type is incompatible 00079 */ 00080 uint8_t TLVList::getUInt8() 00081 { 00082 switch(m_type) 00083 { 00084 case UINT8: 00085 return m_uint8; 00086 case UINT32: 00087 return m_uint32 & 0xFF; 00088 default: 00089 return 0; 00090 } 00091 } 00092 00093 /** Get uint32_t value 00094 * If the current item's type is uint8_t, the value will be casted to uint32_t 00095 * @return uint32_t value OR 0 if the type is incompatible 00096 */ 00097 uint32_t TLVList::getUInt32() 00098 { 00099 switch(m_type) 00100 { 00101 case UINT32: 00102 return m_uint32; 00103 case UINT8: 00104 return m_uint8 & 0xFF; 00105 default: 00106 return 0; 00107 } 00108 } 00109 00110 /** Get array length 00111 * @return bytes array length 00112 */ 00113 size_t TLVList::getArrayLength() 00114 { 00115 switch(m_type) 00116 { 00117 case UINT8_ARRAY: 00118 return m_arrayLen; 00119 default: 00120 return 0; 00121 } 00122 } 00123 00124 /** Get array 00125 * @param buf pointer to buffer's start 00126 * @param maxLen maximum number of bytes to copy 00127 * @return number of copied bytes 00128 */ 00129 size_t TLVList::getArray(uint8_t* buf, size_t maxLen) 00130 { 00131 if(m_type != UINT8_ARRAY) 00132 { 00133 return 0; 00134 } 00135 size_t len = MIN(maxLen, m_arrayLen); 00136 memcpy(buf, m_array, len); 00137 return len; 00138 } 00139 00140 /** Get string length 00141 * @return string length 00142 */ 00143 size_t TLVList::getStringLength() 00144 { 00145 switch(m_type) 00146 { 00147 case STRING: 00148 return m_strLen; 00149 default: 00150 return 0; 00151 } 00152 } 00153 00154 00155 /** Get string 00156 * Copy string to buffer (including null-terminating char) 00157 * @param str pointer to string's start 00158 * @param maxLen maximum number of chars to copy (not including null-terminating char) 00159 * @return number of copied chars 00160 */ 00161 size_t TLVList::getString(char* str, size_t maxLen) 00162 { 00163 if(m_type != STRING) 00164 { 00165 return 0; 00166 } 00167 size_t len = MIN(maxLen, m_strLen); 00168 memcpy(str, m_str, len); 00169 str[len] = '\0'; //Add null-terminating char 00170 return len; 00171 } 00172 00173 /* Setters */ 00174 00175 /** Check whether there is space left in list 00176 * @return true if there is space left, false otherwise 00177 */ 00178 bool TLVList::isSpace() 00179 { 00180 return m_space; 00181 } 00182 00183 /** Put uint8_t value 00184 * @param value uint8_t value 00185 * @return true on success, false if there is not enough space in buffer 00186 */ 00187 bool TLVList::putUInt8(uint8_t value) 00188 { 00189 int res = appnearme_ndef_tlv_put_uint8(m_tlvList, value); 00190 if( !res ) 00191 { 00192 m_space = false; 00193 return false; 00194 } 00195 return true; 00196 } 00197 00198 /** Put uint32_t value 00199 * @param value uint32_t value 00200 * @return true on success, false if there is not enough space in buffer 00201 */ 00202 bool TLVList::putUInt32(uint32_t value) 00203 { 00204 int res = appnearme_ndef_tlv_put_uint32(m_tlvList, value); 00205 if( !res ) 00206 { 00207 m_space = false; 00208 return false; 00209 } 00210 return true; 00211 } 00212 00213 /** Put array 00214 * @param buf pointer to buffer's start 00215 * @param len number of bytes to copy 00216 * @return number of copied bytes 00217 */ 00218 size_t TLVList::putArray(uint8_t* buf, size_t len) 00219 { 00220 int res = appnearme_ndef_tlv_put_array(m_tlvList, buf, len); 00221 if( !res ) 00222 { 00223 m_space = false; 00224 return 0; 00225 } 00226 return len; 00227 } 00228 00229 /** Put string 00230 * @param str pointer to null-terminated string's start 00231 * @return number of copied bytes 00232 */ 00233 size_t TLVList::putString(char* str) 00234 { 00235 int res = appnearme_ndef_tlv_put_string(m_tlvList, str); 00236 if( !res ) 00237 { 00238 m_space = false; 00239 return 0; 00240 } 00241 return strlen(str); 00242 } 00243 00244 00245 00246
Generated on Tue Jul 12 2022 17:28:36 by
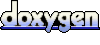