AppNearMe µNFC stack for the NXP PN532 chip License: You can use the stack free of charge to prototype with mbed; if you want to use the stack with your commercial product, get in touch!
Dependents: IOT_sensor_nfc AppNearMe_MuNFC_PN532_Test p2p_nfc_test NFCMoodLamp ... more
EventCallback.h
00001 /* 00002 EventCallback.h 00003 Copyright (c) Donatien Garnier 2012 00004 donatien.garnier@appnearme.com 00005 http://www.appnearme.com/ 00006 */ 00007 00008 00009 #ifndef EVENTCALLBACK_H_ 00010 #define EVENTCALLBACK_H_ 00011 00012 #include "NFCEvent.h" 00013 00014 #include "munfc/core/fwk.h" 00015 #include "munfc/event/transaction_event.h" 00016 00017 class EventCallback 00018 { 00019 protected: 00020 EventCallback(); 00021 00022 void init( void (*fn)(transaction_event_callback, void*) ); //Callable by MuNFC 00023 00024 void attach(void (*fn)(NFCEvent, void*), void* arg); 00025 00026 template <class T> 00027 void attach(T* inst, void (T::*member)(NFCEvent)) 00028 { 00029 m_fn = NULL; 00030 m_inst = inst; 00031 memcpy(m_pMember, (char*)&member, sizeof(member)); 00032 m_caller = &EventCallback::memberCaller<T>; 00033 } 00034 00035 00036 private: 00037 void callback(transaction_event event, transaction_type type); 00038 00039 //Function 00040 void(*m_fn)(NFCEvent, void*); 00041 void* m_arg; 00042 00043 00044 //Member of object instance 00045 void* m_inst; 00046 char m_pMember[16]; 00047 void (*m_caller)(EventCallback*, NFCEvent); 00048 00049 template <class T> 00050 static inline void memberCaller(EventCallback* p, NFCEvent event) 00051 { 00052 T* inst = (T*) p->m_inst; 00053 void (T::*member)(NFCEvent); 00054 memcpy(&member, p->m_pMember, sizeof(member)); 00055 (inst->*member)(event); 00056 } 00057 00058 static void staticCallback(transaction_event event, transaction_type type, void* param); 00059 00060 friend class MuNFC; 00061 }; 00062 00063 00064 00065 #endif /* EVENTCALLBACK_H_ */
Generated on Tue Jul 12 2022 17:28:36 by
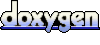