Moves a glow up and down a strip of WS2812 LEDs
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "wsDrive.h" 00003 00004 // time period between each movement 00005 #define updatePeriodMS 15 00006 00007 // number of LEDs in chain 00008 #define chainLen 144 00009 00010 // set the pulldown and then create the driver 00011 DigitalIn dummy(P0_21,PullDown); 00012 wsDrive ledDriver(P0_21,P0_22,P1_15); 00013 00014 // mbuino stnadard definitions 00015 DigitalIn progMode(P0_3,PullDown); // fix the power wasted if we ever sleep. 00016 BusOut LEDs(LED1, LED2, LED3, LED4, LED5, LED6, LED7); // control the LEDs 00017 00018 Timer updateRateTimer; 00019 00020 // pixel storage buffer 00021 pixelInfo16 pixelData[chainLen]; 00022 00023 const uint8_t trailCount = 3; 00024 00025 // info for each trail 00026 struct trailInfo { 00027 float start; // location of the trail from 0 to chainLen-1 00028 int length; // length of the trail 00029 float speed; // speed in moves at in LEDs per update 00030 int backgroundRatio; // background glow level 00031 pixelInfo colour; // colour (and brightness) at the start of the chain 00032 bool dir; // direction of travel - true = increasing location 00033 }; 00034 00035 00036 struct trailInfo lines[trailCount]; 00037 00038 00039 void blankBuffer(pixelInfo *Ptr) 00040 { 00041 memset( (void *)Ptr, 0, chainLen*sizeof(pixelInfo) ); 00042 } 00043 00044 void blankBuffer(pixelInfo16 *Ptr) 00045 { 00046 memset( (void *)Ptr, 0, chainLen*sizeof(pixelInfo16) ); 00047 } 00048 00049 void setPixel (pixelInfo *pixel, pixelInfo *colour, float level) 00050 { 00051 pixel->R = (colour->R * level); 00052 pixel->G = (colour->G * level); 00053 pixel->B = (colour->B * level); 00054 } 00055 00056 void addPixel (pixelInfo16 *pixel, pixelInfo *colour, float level) 00057 { 00058 pixel->R = pixel->R + (int)(colour->R * level); 00059 pixel->G = pixel->G + (int)(colour->G * level); 00060 pixel->B = pixel->B + (int)(colour->B * level); 00061 } 00062 00063 void subtractPixel (pixelInfo16 *pixel, pixelInfo *colour, float level) 00064 { 00065 pixel->R = pixel->R - (int)(colour->R * level); 00066 pixel->G = pixel->G - (int)(colour->G * level); 00067 pixel->B = pixel->B - (int)(colour->B * level); 00068 } 00069 00070 00071 void setTrail(bool add, pixelInfo16* buffer, pixelInfo *colour, int peakPoint, bool increasing, int len) 00072 { 00073 int pixelToUpdate = peakPoint; 00074 for (int pixel = 0; pixel < len; pixel++) { 00075 00076 if (add) 00077 addPixel((buffer+pixelToUpdate), colour, 1.0 - (float)pixel/(float)len); 00078 else 00079 subtractPixel((buffer+pixelToUpdate), colour, 1.0 - (float)pixel/(float)len); 00080 00081 increasing ? pixelToUpdate-- : pixelToUpdate++; 00082 00083 if (pixelToUpdate == chainLen) { 00084 increasing = false; 00085 pixelToUpdate = chainLen-2; 00086 } 00087 if (pixelToUpdate == -1) { 00088 increasing = true; 00089 pixelToUpdate = 1; 00090 } 00091 } 00092 } 00093 00094 void removeTrail (pixelInfo16* buffer, pixelInfo *colour, int peakPoint, bool increasing, int len) 00095 { 00096 setTrail (false, buffer, colour, peakPoint, increasing, len); 00097 } 00098 00099 void addTrail (pixelInfo16* buffer, pixelInfo *colour, int peakPoint, bool increasing, int len) 00100 { 00101 setTrail (true, buffer, colour, peakPoint, increasing, len); 00102 } 00103 00104 00105 int main () 00106 { 00107 LEDs = 0; 00108 00109 00110 // set up the lights. 00111 lines[0].start = 0; 00112 lines[0].speed = 1.1; 00113 lines[0].length = 20; 00114 lines[0].backgroundRatio = 40; 00115 lines[0].colour.R = 120; 00116 lines[0].colour.G = 0; 00117 lines[0].colour.B = 0; 00118 lines[0].dir = true; 00119 00120 lines[1].start = 0; 00121 lines[1].speed = 2.0/3.0; 00122 lines[1].length = 16; 00123 lines[1].backgroundRatio = 40; 00124 lines[1].colour.R = 0; 00125 lines[1].colour.G = 0; 00126 lines[1].colour.B = 120; 00127 lines[1].dir = true; 00128 00129 lines[2].start = 143; 00130 lines[2].speed = 1; 00131 lines[2].length = 20; 00132 lines[2].backgroundRatio = 40; 00133 lines[2].colour.R = 0; 00134 lines[2].colour.G = 120; 00135 lines[2].colour.B = 0; 00136 lines[2].dir = false; 00137 00138 // clear the buffer 00139 blankBuffer(pixelData); 00140 00141 // add the optional background 00142 /* 00143 for (int i = 0; i< chainLen; i++) { 00144 for (int j = 0; j <trailCount; j++) { 00145 addPixel((pixelData+i), &(lines[j].colour), 1.0/lines[j].backgroundRatio); 00146 } 00147 } 00148 */ 00149 00150 // add the initial lines 00151 for (int j = 0; j <trailCount; j++) { 00152 addTrail (pixelData, &(lines[j].colour), lines[j].start, lines[j].dir, lines[j].length); // set the LED data 00153 } 00154 // give the LED driver the buffer to use. 00155 ledDriver.setData(pixelData, chainLen); 00156 00157 LEDs = 1; 00158 00159 updateRateTimer.start(); 00160 while (true) { 00161 ledDriver.sendData(); // send the LED data 00162 00163 LEDs = LEDs+1; 00164 00165 // subtract the current trail locations and then add the new locations. 00166 for (int j = 0; j <trailCount; j++) { 00167 removeTrail (pixelData, &(lines[j].colour), lines[j].start, lines[j].dir, lines[j].length); // set the LED data 00168 00169 lines[j].dir ? lines[j].start+=lines[j].speed : lines[j].start-=lines[j].speed; 00170 if ((int)lines[j].start >= chainLen) { 00171 lines[j].dir = false; 00172 lines[j].start = chainLen-1 - lines[j].speed; 00173 } 00174 if ((int)lines[j].start <= -1) { 00175 lines[j].dir = true; 00176 lines[j].start = lines[j].speed; 00177 } 00178 addTrail (pixelData, &(lines[j].colour), lines[j].start, lines[j].dir, lines[j].length); // set the LED data 00179 } 00180 // wait for the next update time. 00181 while (updateRateTimer.read_ms() < updatePeriodMS) { 00182 } 00183 updateRateTimer.reset(); 00184 } 00185 00186 }
Generated on Tue Jul 19 2022 12:26:12 by
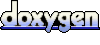