
Triggers Lightning LED and sounds effects
Embed:
(wiki syntax)
Show/hide line numbers
effects.cpp
00001 #include "mbed.h" 00002 00003 // LED on/off times. 00004 // First time is time between toggling sound IO pin and starting lights 00005 // Allows for audio playback start latency and lead in time. 00006 const float thunder1Times[] = {0.1,0.3,0.1,0.3,0.1,0.2,0.2,0.3,0.1,0.3}; 00007 const int thunder1Len = 1 + 1 + 1 + 1 + 1 + 1 + 1 + 1 + 1 + 1 ; 00008 00009 const float thunder2Times[] = {0.1,0.3,0.1,0.3,0.1,0.2, 0.6 ,0.3,0.1,0.3,0.2,0.1, 2 ,0.3,0.15,0.4,0.1,0.2}; 00010 const int thunder2Len = 1 + 1 + 1 + 1 + 1 + 1 + 1 + 1 + 1 + 1 + 1 + 1 + 1 + 1 + 1 + 1 + 1 + 1 ; 00011 00012 // min time between starting a thunder playback and starting the next audio 00013 const float thunder1AudioLen = 20; 00014 const float thunder2AudioLen = 25; 00015 00016 // min time between starting a SFX playback and starting the next audio 00017 const float effectAudioLen = 5; 00018 00019 00020 // actual time between sound starts will be these numbers plus the thunder/effect length above. 00021 const float minTimeBetweenSounds = 10; 00022 const float maxTimeBetweenSounds = 20; 00023 00024 // percentage chance of lightnings 00025 const int pLightning = 40; 00026 const int pLightning2 = 15; 00027 00028 00029 const int randomRange = 10*(maxTimeBetweenSounds - minTimeBetweenSounds); // range of time between sounds in units of 10ths of a second 00030 00031 00032 DigitalOut soundEffectPin(P0_19); 00033 DigitalOut thunder1Pin(P0_4); 00034 DigitalOut thunder2Pin(P0_5); 00035 00036 DigitalOut LEDsPin(P0_18); 00037 00038 DigitalOut LED_1(LED1); 00039 DigitalOut LED_2(LED2); 00040 DigitalOut LED_3(LED3); 00041 BusOut LED_47(LED4,LED5,LED6,LED7); 00042 00043 00044 00045 00046 void LEDon() 00047 { 00048 LEDsPin = 1; 00049 LED_1 = 1; 00050 } 00051 00052 void LEDoff() 00053 { 00054 LEDsPin = 0; 00055 LED_1 = 0; 00056 } 00057 00058 void toggleLED() 00059 { 00060 LEDsPin = !LEDsPin; 00061 LED_1 = LEDsPin; 00062 } 00063 00064 void randomSoundEffect(void) 00065 { 00066 LED_2 = 1; 00067 soundEffectPin = 0; 00068 wait(1); 00069 LED_2 = 0; 00070 soundEffectPin = 1; 00071 wait(effectAudioLen); 00072 } 00073 00074 void thunderEffect1(void) 00075 { 00076 LEDoff(); 00077 thunder1Pin = 0; 00078 int count = 0; 00079 float totalTime = 0; 00080 while (count < thunder1Len) { 00081 wait (thunder1Times[count]); 00082 totalTime += thunder1Times[count]; 00083 toggleLED(); 00084 count++; 00085 } 00086 LEDoff(); 00087 thunder1Pin = 1; 00088 if (thunder1AudioLen > totalTime) 00089 wait (thunder1AudioLen - totalTime); 00090 } 00091 00092 void thunderEffect2(void) 00093 { 00094 LEDoff(); 00095 thunder2Pin = 0; 00096 int count = 0; 00097 float totalTime = 0; 00098 while (count < thunder2Len) { 00099 wait (thunder2Times[count]); 00100 totalTime += thunder2Times[count]; 00101 toggleLED(); 00102 count++; 00103 } 00104 LEDoff(); 00105 thunder2Pin = 1; 00106 if (thunder2AudioLen > totalTime) 00107 wait (thunder2AudioLen - totalTime); 00108 } 00109 00110 00111 void playEffect(void) 00112 { 00113 int randomOrder = rand() % 100; 00114 LED_47 = randomOrder; 00115 LED_3 = 1; 00116 if (randomOrder < pLightning) { 00117 LED_47 = 1; 00118 thunderEffect1(); 00119 } else if (randomOrder < (pLightning+pLightning2)) { 00120 LED_47 = 2; 00121 thunderEffect2(); 00122 } else { 00123 LED_47 = 3; 00124 randomSoundEffect(); 00125 } 00126 LED_3 = 0; 00127 } 00128 00129 void setupRandom(void) 00130 { 00131 AnalogIn RandomIn(P0_14); 00132 // create a 32 bit number out of 32 LSBs from the ADC 00133 uint32_t seedValue = 0; 00134 uint16_t value; 00135 uint8_t counter; 00136 00137 for (counter = 0; counter < 32; counter++) { 00138 seedValue = seedValue<<1; 00139 value = RandomIn.read_u16(); // reads a 10 bit ADC normalised to 16 bits. 00140 if (value & 0x0040) // LSB of ADC output = 1 00141 seedValue++; 00142 } 00143 00144 srand(seedValue); 00145 } 00146 00147 main() 00148 { 00149 LED_47 = 0; 00150 LED_2 = 0; 00151 LED_3 = 0; 00152 LEDoff(); 00153 thunder1Pin = 1; 00154 thunder2Pin = 1; 00155 soundEffectPin = 1; 00156 00157 setupRandom(); 00158 00159 float randomTime; 00160 while (true) { 00161 randomTime = minTimeBetweenSounds + (rand() % randomRange)/10.0; 00162 wait(randomTime); 00163 playEffect(); 00164 } 00165 }
Generated on Wed Jul 13 2022 01:02:29 by
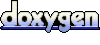