DW1000 UWB driver based on work of Matthias Grob & Manuel Stalder - ETH Zürich - 2015
Embed:
(wiki syntax)
Show/hide line numbers
DW1000Setup.h
00001 #ifndef __DW1000SETUP_H__ 00002 #define __DW1000SETUP_H__ 00003 00004 #include "mbed.h" 00005 /** Class for holding DW1000 config options 00006 * 00007 * Defaults are channel 5, 16MHz PRF, 850kb/s, standard SFD, 128 symbol preamble, preamble code 3. 00008 * All pins are GPIO other than sync and IRQ. 00009 * Smart power is off. 00010 * 00011 */ 00012 class DW1000Setup 00013 { 00014 public: 00015 00016 /** Supported UWB modes 00017 * 00018 * enum for preset mode combinations 00019 */ 00020 typedef enum {fastLocationC5, ///< maximum data speed, short range. Channel 5 00021 fastLocationC4, ///< maximum data speed, short range. Channel 4 00022 tunedDefault, ///< The power up default config with the recomended changes 00023 user110k, ///< 110kb/s settings used by Matthias Grob & Manuel Stalder 00024 rangeRateCompromise ///< 850kb/s settings aimed to give a short packet but with far greater range than the fastLocation options 00025 } UWBMode; 00026 00027 /** Constructor 00028 * @param modeToUse The operating mode to set the initial settings to. 00029 * 00030 */ 00031 DW1000Setup(UWBMode modeToUse = tunedDefault); 00032 00033 00034 /// copy constructor 00035 DW1000Setup(DW1000Setup *orij); 00036 00037 /// Update this config to match the supplied one 00038 void applyConfig(DW1000Setup *orij); 00039 00040 00041 /// enum for PRF options 00042 enum prf_e {prf16MHz, ///< PRF rate of 16MHz. Lower power 00043 prf64MHz ///< PRF rate of 64MHz. Higher power but more accurate timing. 00044 }; 00045 00046 /// enum for data rate options 00047 enum dataRate_e {kbps110, ///< Data rate of 110kb/s (non-standard) 00048 kbps850,///< Data rate of 850kb/s 00049 kbps6800///< Data rate of 6.8Mb/s 00050 }; 00051 00052 /** enum for SFD options 00053 * 00054 * To conform to the standard the standard setting should be used. 00055 * The decawave setting claimes to give better performance. Doesn't work for me at 850kb/s but does at 6.8Mb/s 00056 */ 00057 enum sfd_e {standard, ///< IEEE standard SFD 00058 decaWave, ///< Decawave defined SFD 00059 user ///< user defined SFD 00060 }; 00061 00062 /// enum for preamble length options 00063 enum preamble_e { pre64,///< Preamble is 64 symbols 00064 pre128,///< Preamble is 128 symbols (non-standard) 00065 pre256,///< Preamble is 256 symbols (non-standard) 00066 pre512,///< Preamble is 512 symbols (non-standard) 00067 pre1024,///< Preamble is 1024 symbols 00068 pre1536,///< Preamble is 1536 symbols (non-standard) 00069 pre2048, ///< Preamble is 2048 symbols (non-standard) 00070 pre4096///< Preamble is 4096 symbols 00071 }; 00072 00073 /** Set the Transmit power for smartpower operation 00074 * @param powerdBm The gain setting in dBm (0-33.5) 00075 * @param power500us The gain setting packets under 500us in dBm (0-33.5) 00076 * @param power250us The gain setting packets under 250us in dBm (0-33.5) 00077 * @param power125us The gain setting packets under 125us in dBm (0-33.5) 00078 * @return true if a valid option 00079 */ 00080 bool setSmartTxPower(float powerdBm,float power500us=0,float power250us=0,float power125us=0) ; 00081 00082 /** Set the Transmit power for non-smartpower operation 00083 * @param powerdBm The gain setting in dBm for the main packet(0-33.5) 00084 * @param powerPRF The gain setting in dBm for the preamble (0-33.5) 00085 * @return true if a valid option 00086 */ 00087 bool setTxPower(float powerdBm,float powerPRF=0); 00088 00089 00090 /** Set the PRF 00091 * @return true if a valid option 00092 */ 00093 bool setPRF(enum prf_e newSetting) { 00094 prf = newSetting; 00095 return true; 00096 }; 00097 00098 /** Set the Channel 00099 * @return true if a valid option 00100 * 00101 * channels are 1-5 & 7 00102 */ 00103 bool setChannel(unsigned char newChannel) { 00104 if ((newChannel > 0) && ((newChannel <= 5) || (newChannel == 7))) { 00105 channel = newChannel; 00106 return true; 00107 } 00108 return false; 00109 }; 00110 /** Set the SFD 00111 * @return true if a valid option 00112 */ 00113 bool setSfd(enum sfd_e newSetting) { 00114 sfd = newSetting; 00115 return true; 00116 }; 00117 /** Set the Preamble length 00118 * @return true if a valid option 00119 * 00120 * For 6.8Mb/s it should be 64 to 256 symbols. 00121 * For 850kb/s it should be 256 to 2048 symbols 00122 * For 110kb/s it should be >= 2048 symbols. 00123 */ 00124 bool setPreambleLength(enum preamble_e newSetting) { 00125 preamble = newSetting; 00126 return true; 00127 }; 00128 /** Set the Data rate 00129 * @return true if a valid option 00130 */ 00131 bool setDataRate(enum dataRate_e newSetting) { 00132 dataRate = newSetting; 00133 return true; 00134 }; 00135 /** Set the Preamble code 00136 * @return true if a valid option 00137 * 00138 * @note Not all codes are valid for all channels, see the table below 00139 00140 <table> 00141 <tr><th>channel</th><th>16MHzPrf</th><th>64MHzPrf</th></tr> 00142 <tr><td>1</td><td>1, 2</td><td>9, 10, 11, 12</td></tr> 00143 <tr><td>2</td><td>3, 4</td><td>9, 10, 11, 12</td></tr> 00144 <tr><td>3</td><td>5, 6</td><td>9, 10, 11, 12</td></tr> 00145 <tr><td>4</td><td>7, 8</td><td>17, 18, 19, 20</td></tr> 00146 <tr><td>5</td><td>3, 4</td><td>9, 10, 11, 12</td></tr> 00147 <tr><td>7</td><td>7, 8</td><td>17, 18, 19, 20</td></tr> 00148 </table> 00149 00150 */ 00151 bool setPreambleCode(unsigned char newCode) { 00152 if ((newCode > 0) && (newCode <= 24)) { 00153 preambleCode = newCode; 00154 return true; 00155 } 00156 return false; 00157 }; 00158 /** Set the smartpower state 00159 * @return true if a valid option 00160 * 00161 * only takes effect at 6.8Mb/s 00162 */ 00163 bool setSmartPower(bool enable) { 00164 enableSmartPower = enable; 00165 return true; 00166 }; 00167 00168 00169 /** Get the current transmit gains 00170 * @return An array containing the power levels 00171 * 00172 * An array of 4 floats is returned. 00173 * For smart power these are the 4 power levels (normal, <500us, <250us, <125us). 00174 * For non-smart power the first and last values are ignored, the second is the power for the phy header, the 3rd is the power for the reaminder of the frame. 00175 */ 00176 const float *getTxPowers() { 00177 return powers; 00178 } 00179 00180 /** Get the current channel 00181 * @return the channel number 00182 */ 00183 unsigned char getChannel() { 00184 return channel; 00185 }; 00186 /** Get the current PRF 00187 * @return the PRF 00188 */ 00189 enum prf_e getPRF() { 00190 return prf; 00191 }; 00192 /** Get the current data rate 00193 * @return the data rate 00194 */ 00195 enum dataRate_e getDataRate() { 00196 return dataRate; 00197 }; 00198 00199 /** Get the current SFD mode 00200 * @return the SFD 00201 */ 00202 enum sfd_e getSfd() { 00203 return sfd; 00204 }; 00205 /** Get the current preamble length 00206 * @return the preamble length 00207 */ 00208 enum preamble_e getPreambleLength() { 00209 return preamble; 00210 }; 00211 /** Get the current preamble code 00212 * @return the preamble code 00213 */ 00214 unsigned char getPreambleCode() { 00215 return preambleCode; 00216 }; 00217 /** Get the current smart power mode 00218 * @return true if smartpower is on 00219 */ 00220 bool getSmartPower() { 00221 return enableSmartPower; 00222 }; 00223 00224 /** Set which pins are GPIO 00225 * 00226 * @param pins A bitmask of which pins are not to be used as GPIO 00227 * 00228 * e.g. 0x01 sets GPIO0 as it's alternative function (RXOKLED) and all other pins to be GPIO. 00229 * 00230 * The default is 0x0180 - GPIO 8 = irq, GPIO 7 = sync. All others are GPIO functionality 00231 */ 00232 void setGPIO(uint16_t pins) { 00233 GPIO_Pins = pins; 00234 }; 00235 00236 /** Get which pins are GPIO 00237 * 00238 * @return A bitmask of which pins are not to be used as GPIO 00239 * 00240 * e.g. 0x01 Indicates that GPIO0 as it's alternative function (RXOKLED) and all other pins to be GPIO. 00241 * 00242 * The default is 0x0180 - GPIO 8 = irq, GPIO 7 = sync. All others are GPIO functionality 00243 */ 00244 uint16_t getGPIO() { 00245 return GPIO_Pins; 00246 }; 00247 00248 /** Check that the settings are self consistent 00249 * 00250 * @return true if the settings are valid 00251 * 00252 * Will check the following: 00253 * - Preamble size is sensible for the data rate 00254 * - Preamble code is valid for the channel 00255 * - That smart power is only enabled at 6.8Mb/s 00256 */ 00257 bool check(); 00258 00259 /** Get setup description 00260 * 00261 * @param buffer Data buffer to place description in 00262 * @param len Length of data buffer 00263 * 00264 * Places a text string describing the current setup into the suppled buffer. 00265 */ 00266 void getSetupDescription(char *buffer, int len); 00267 00268 private: 00269 float powers[4]; 00270 uint16_t GPIO_Pins; 00271 unsigned char channel; // 1-5 , 7 00272 enum prf_e prf; 00273 enum dataRate_e dataRate; 00274 enum sfd_e sfd; 00275 enum preamble_e preamble; 00276 unsigned char preambleCode; // 1-24. See section 10.5 of user manual for details. 00277 bool enableSmartPower; 00278 }; 00279 00280 #endif
Generated on Mon Jul 18 2022 07:51:56 by
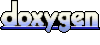