A library to interface with the LSM9DS1 IMU using SPI
Embed:
(wiki syntax)
Show/hide line numbers
LSM9DS1_SPI.h
00001 // Written by: Erik van de Coevering 00002 00003 #ifndef __LSM9DS1_SPI_H__ 00004 #define __LSM9DS1_SPI_H__ 00005 00006 #include "mbed.h" 00007 00008 class lsm9ds1_spi 00009 { 00010 SPI& spi; 00011 DigitalOut csAG; 00012 DigitalOut csM; 00013 00014 public: 00015 lsm9ds1_spi(SPI& _spi, PinName _csAG, PinName _csM); 00016 unsigned int WriteRegAG(uint8_t WriteAddr, uint8_t WriteData); 00017 unsigned int WriteRegM(uint8_t WriteAddr, uint8_t WriteData); 00018 unsigned int ReadRegAG(uint8_t WriteAddr, uint8_t WriteData); 00019 unsigned int ReadRegM(uint8_t WriteAddr, uint8_t WriteData); 00020 void ReadRegsAG(uint8_t ReadAddr, uint8_t *ReadBuf, unsigned int Bytes ); 00021 void ReadRegsM(uint8_t ReadAddr, uint8_t *ReadBuf, unsigned int Bytes ); 00022 00023 void selectAG(); 00024 void selectM(); 00025 void deselectAG(); 00026 void deselectM(); 00027 00028 void init(); 00029 unsigned int whoami(); 00030 unsigned int whoamiM(); 00031 float read_temp(); // reads temperature in degrees C 00032 void read_acc(); // reads accelerometer in G 00033 void read_gyr(); // reads gyroscope in DPS 00034 void read_mag(); // reads magnetometer in gauss 00035 void read_all(); // reads acc / gyro / magneto 00036 00037 float acc_multiplier; 00038 float gyro_multiplier; 00039 float mag_multiplier; 00040 float accelerometer_data[3]; 00041 float gyroscope_data[3]; 00042 float magnetometer_data[3]; 00043 00044 }; 00045 00046 #define FS_G_245DPS 0x00 00047 #define FS_G_500DPS 0x08 00048 #define FS_G_2000DPS 0x18 00049 #define ODR_G_15HZ 0x20 00050 #define ODR_G_60HZ 0x40 00051 #define ODR_G_119HZ 0x60 00052 #define ODR_G_238HZ 0x80 00053 #define ODR_G_476HZ 0xA0 00054 #define ODR_G_952HZ 0xC0 00055 #define BW_G_33HZ 0x00 // Bandwidth dependant on ODR, these freq's are correct for ODR=476Hz or 952Hz. Check datasheet for other ODR's 00056 #define BW_G_40HZ 0x01 00057 #define BW_G_58HZ 0x02 00058 #define BW_G_100HZ 0x03 00059 #define FS_A_2G 0x00 00060 #define FS_A_4G 0x10 00061 #define FS_A_8G 0x18 00062 #define FS_A_16G 0x08 00063 #define FS_M_4GAUSS 0x00 00064 #define FS_M_8GAUSS 0x20 00065 #define FS_M_12GAUSS 0x40 00066 #define FS_M_16GAUSS 0x60 00067 00068 #define ACC_SENS_2G 0.000061f 00069 #define ACC_SENS_4G 0.000122f 00070 #define ACC_SENS_8G 0.000244f 00071 #define ACC_SENS_16G 0.000732f 00072 #define GYR_SENS_245DPS 0.00875f 00073 #define GYR_SENS_500DPS 0.0175f 00074 #define GYR_SENS_2000DPS 0.07f 00075 #define MAG_SENS_4GAUSS 0.00014f 00076 #define MAG_SENS_8GAUSS 0.00029f 00077 #define MAG_SENS_12GAUSS 0.00043f 00078 #define MAG_SENS_16GAUSS 0.000732f 00079 00080 #define READ_FLAG 0x80 00081 00082 #endif 00083 00084
Generated on Sun Jul 24 2022 17:12:27 by
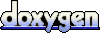