A basic library for RS485 communication
RS485 Class Reference
#include <RS485.h>
Public Member Functions | |
RS485 (PinName tx, PinName rx, PinName dere) | |
Create a BufferedSerial port, connected to the specified transmit and receive pins. | |
void | sendComplemented (const byte what) |
sendComplemented byte send a byte complemented, repeated only values sent would be (in hex): 0F, 1E, 2D, 3C, 4B, 5A, 69, 78, 87, 96, A5, B4, C3, D2, E1, F0 the byte to complement | |
void | sendMsg (const byte *data, const byte length) |
send message cyclic redundancy check | |
byte | recvMsg (byte *data, const byte length, unsigned long timeout) |
receive message reads serial port and populates data | |
Static Public Member Functions | |
static byte | crc8 (const byte *addr, byte len) |
calculate 8-bit CRC cyclic redundancy check |
Detailed Description
RS485 Library.
Can send from 1 to 255 bytes from one node to another with:
Packet start indicator (STX) Each data byte is doubled and inverted to check validity Packet end indicator (ETX) Packet CRC (checksum)
Using MAX485 modules or the MAX485 CSA+
Example:
#include "mbed.h" #include <RS485.h> Serial pc(USBTX, USBRX); RS485 RS485(PC_10,PC_11,PB_3); // Tx, Rx , !RE and DE MAX485 pin DigitalOut ho(PB_3); // this pin should be connected to !RE and DE typedef uint8_t byte; byte regvalue[9]; byte data[9] = {0x01,0x04,0x00,0x48,0x00,0x02,0xf1,0xdd};//your data int main() { pc.printf("main\n"); while(1) { pc.printf("Starting\n"); ho = 1; // Enable sending on MAX485 RS485.sendMsg(data,sizeof(data)); wait_ms(600); // Must wait for all the data to be sent ho = 0; // Enable receiving on MAX485 pc.printf("Getting data\n"); if(RS485.readable() >0){ memset(regvalue,0,sizeof(regvalue)); wait_ms(200); RS485.recvMsg(regvalue,sizeof(data),500); wait_ms(200); for (int count = 0; count < 9; count++) { pc.printf("%X - ", regvalue[count]); } }else printf("No Data\n"); printf("Done\n"); wait_ms(1000); } }
Definition at line 82 of file RS485.h.
Constructor & Destructor Documentation
RS485 | ( | PinName | tx, |
PinName | rx, | ||
PinName | dere | ||
) |
Member Function Documentation
byte crc8 | ( | const byte * | addr, |
byte | len | ||
) | [static] |
byte recvMsg | ( | byte * | data, |
const byte | length, | ||
unsigned long | timeout | ||
) |
void sendComplemented | ( | const byte | what ) |
Generated on Tue Jul 12 2022 20:26:33 by
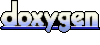