a
Embed:
(wiki syntax)
Show/hide line numbers
HCSR04.h
00001 #ifndef HCSR04_H 00002 #define HCSR04_H 00003 00004 #include "mbed.h" 00005 00006 00007 00008 /** 00009 * Sonar HC-SR04 example. 00010 * @code 00011 * #include "mbed.h" 00012 * #include "HCSR04.h" 00013 * 00014 * DigitalOut myled(LED1); 00015 * Serial pc(USBTX,USBRX); 00016 * 00017 * HCSR04 sonar(PTD5, PTA13); 00018 * 00019 * int main() { 00020 * while(1) { 00021 * printf("Distancia detectada pelo sensor Frente %.2f cm \n", sonar.getCm()); 00022 * wait_ms(1000); 00023 * } 00024 * } 00025 * 00026 * @endcode 00027 */ 00028 00029 class HCSR04 { 00030 public: 00031 /** Constructor, create HCSR04 instance 00032 * 00033 * @param trigger TRIG pin 00034 * @param echo ECHO pin 00035 */ 00036 HCSR04(PinName trigger, PinName echo); 00037 00038 /** It make a reading of the sonar Faz uma leitura do sonar 00039 * 00040 * @returns Tempo do pulso echo em microsegundos*/ 00041 float readEcho(void); 00042 00043 /** It messures the distance in centimeter "cm" 00044 * 00045 *@returns Distance in centimeter 00046 */ 00047 float getCm(void); 00048 00049 /** Mede a distência em polegadas "in" 00050 * 00051 *@returns Distência em in*/ 00052 float getIn(void); 00053 00054 private: 00055 float tdist; //Leitura do tempo transcorrido 00056 float distcm; //Guarda o valor da distanciância em centímetros 00057 float distin; //Guarda o valor da distência em polegadas 00058 00059 DigitalOut _t; //Configuração do pino de Trigger 00060 DigitalIn _e; //Configuração do pino de Echo 00061 Timer _tempo; //Cria um objeto timer 00062 00063 }; 00064 00065 #endif
Generated on Sun Aug 7 2022 14:02:38 by
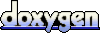