
A quick and dirty FTP server port to mbed, for file i/o ops via LwIP stack.
Embed:
(wiki syntax)
Show/hide line numbers
vfs.h
00001 /** 00002 * @file ap_vfs.h 00003 * @brief Virtual File System interface 00004 * 00005 * @defgroup APACHE_CORE_VFS Virtual File System interface 00006 * @ingroup APACHE_CORE 00007 * @{ 00008 */ 00009 00010 #ifndef APACHE_VFS_H 00011 #define APACHE_VFS_H 00012 00013 #ifdef __cplusplus 00014 extern "C" { 00015 #endif 00016 00017 //..#include "apr.h" 00018 //..#include "apr_file_io.h" 00019 00020 //..#include "httpd.h" 00021 00022 #define AP_USE_VFS 1 00023 #if !AP_USE_VFS 00024 00025 /* VFS Interface disabled */ 00026 00027 #define vfs_file_t apr_file_t 00028 #define vfs_dir_t apr_dir_t 00029 00030 #define ap_vfs_stat(r, finfo, fname, wanted, pool) \ 00031 apr_stat(finfo, fname, wanted, pool) 00032 #define ap_vfs_file_open(r, new, fname, flag, perm, pool) \ 00033 apr_file_open(new, fname, flag, perm, pool) 00034 #define ap_vfs_file_read(thefile, buf, nbytes) \ 00035 apr_file_read(thefile, buf, nbytes) 00036 #define ap_vfs_file_write(thefile, buf, nbytes) \ 00037 apr_file_write(thefile, buf, nbytes) 00038 #define ap_vfs_file_seek(thefile, where, offset) \ 00039 apr_file_seek(thefile, where, offset) 00040 #define ap_vfs_file_eof(thefile) \ 00041 apr_file_eof(thefile) 00042 #define ap_vfs_file_close(thefile) \ 00043 apr_file_close(thefile) 00044 #define ap_vfs_file_remove(r, path, pool) \ 00045 apr_file_remove(path, pool) 00046 #define ap_vfs_file_rename(r, from_path, to_path, p) \ 00047 apr_file_rename(from_path, to_path, p) 00048 #define ap_vfs_file_perms_set(r, fname, perms) \ 00049 apr_file_perms_set(fname, perms) 00050 #define ap_vfs_dir_open(r, new, dirname, pool) \ 00051 apr_dir_open(new, dirname, pool) 00052 #define ap_vfs_dir_read(finfo, wanted, thedir) \ 00053 apr_dir_read(finfo, wanted, thedir) 00054 #define ap_vfs_dir_close(thedir) \ 00055 apr_dir_close(thedir) 00056 #define ap_vfs_dir_make(r, path, perm, pool) \ 00057 apr_dir_make(path, perm, pool) 00058 #define ap_vfs_dir_remove(r, path, pool) \ 00059 apr_dir_remove(path, pool) 00060 00061 #else 00062 00063 /* VFS Public Interface */ 00064 00065 #define AP_DECLARE(x) x 00066 typedef int apr_status_t; // DRB 00067 00068 //..typedef struct vfs_file_t vfs_file_t; 00069 typedef struct vfs_dir_t vfs_dir_t; 00070 typedef struct vfs_mount vfs_mount; 00071 00072 #if 0 // DRB 00073 AP_DECLARE(apr_status_t) ap_vfs_stat(const request_rec *r, 00074 apr_finfo_t *finfo, 00075 const char *fname, 00076 apr_int32_t wanted, 00077 apr_pool_t *pool); 00078 00079 AP_DECLARE(apr_status_t) ap_vfs_file_open(const request_rec *r, 00080 vfs_file_t **new, 00081 const char *fname, 00082 apr_int32_t flag, 00083 apr_fileperms_t perm, 00084 apr_pool_t *pool); 00085 00086 AP_DECLARE(apr_status_t) ap_vfs_file_read(vfs_file_t *thefile, 00087 void *buf, 00088 apr_size_t *nbytes); 00089 00090 AP_DECLARE(apr_status_t) ap_vfs_file_write(vfs_file_t *thefile, 00091 const void *buf, 00092 apr_size_t *nbytes); 00093 00094 AP_DECLARE(apr_status_t) ap_vfs_file_seek(vfs_file_t *thefile, 00095 apr_seek_where_t where, 00096 apr_off_t *offset); 00097 00098 AP_DECLARE(apr_status_t) ap_vfs_file_eof(vfs_file_t *file); 00099 00100 AP_DECLARE(apr_status_t) ap_vfs_file_close(vfs_file_t *file); 00101 00102 AP_DECLARE(apr_status_t) ap_vfs_file_remove(const request_rec *r, 00103 const char *path, 00104 apr_pool_t *pool); 00105 00106 AP_DECLARE(apr_status_t) ap_vfs_file_rename(const request_rec *r, 00107 const char *from_path, 00108 const char *to_path, 00109 apr_pool_t *p); 00110 00111 AP_DECLARE(apr_status_t) ap_vfs_file_perms_set(const request_rec *r, 00112 const char *fname, 00113 apr_fileperms_t perms); 00114 00115 AP_DECLARE(apr_status_t) ap_vfs_dir_open(const request_rec *r, 00116 vfs_dir_t **new, 00117 const char *dirname, 00118 apr_pool_t *pool); 00119 00120 AP_DECLARE(apr_status_t) ap_vfs_dir_read(apr_finfo_t *finfo, 00121 apr_int32_t wanted, 00122 vfs_dir_t *thedir); 00123 00124 AP_DECLARE(apr_status_t) ap_vfs_dir_close(vfs_dir_t *thedir); 00125 00126 AP_DECLARE(apr_status_t) ap_vfs_dir_make(const request_rec *r, 00127 const char *path, 00128 apr_fileperms_t perm, 00129 apr_pool_t *pool); 00130 00131 AP_DECLARE(apr_status_t) ap_vfs_dir_remove(const request_rec *r, 00132 const char *path, 00133 apr_pool_t *pool); 00134 00135 /* VFS Provider interface */ 00136 00137 struct vfs_mount { 00138 vfs_mount *next; 00139 apr_uint32_t flags; 00140 const char *mountpoint; 00141 void *userdata; 00142 00143 /* VFS implementation hooks */ 00144 apr_status_t (*stat) (const vfs_mount *mnt, const request_rec *r, 00145 apr_finfo_t *finfo, const char *fname, 00146 apr_int32_t wanted, apr_pool_t *pool); 00147 apr_status_t (*file_open) (const vfs_mount *mnt, const request_rec *r, 00148 vfs_file_t **new, const char *fname, 00149 apr_int32_t flag, apr_fileperms_t perm, 00150 apr_pool_t *pool); 00151 apr_status_t (*file_read) (const vfs_mount *mnt, vfs_file_t *thefile, 00152 void *buf, apr_size_t *nbytes); 00153 apr_status_t (*file_write) (const vfs_mount *mnt, vfs_file_t *thefile, 00154 const void *buf, apr_size_t *nbytes); 00155 apr_status_t (*file_seek) (const vfs_mount *mnt, vfs_file_t *thefile, 00156 apr_seek_where_t where, apr_off_t *offset); 00157 apr_status_t (*file_eof) (const vfs_mount *mnt, vfs_file_t *file); 00158 apr_status_t (*file_close) (const vfs_mount *mnt, vfs_file_t *file); 00159 apr_status_t (*file_remove) (const vfs_mount *mnt, const request_rec *r, 00160 const char *path, apr_pool_t *pool); 00161 apr_status_t (*file_rename) (const vfs_mount *mnt, const request_rec *r, 00162 const char *from_path, const char *to_path, 00163 apr_pool_t *p); 00164 apr_status_t (*file_perms_set)(const vfs_mount *mnt, const request_rec *r, 00165 const char *fname, apr_fileperms_t perms); 00166 apr_status_t (*dir_open) (const vfs_mount *mnt, const request_rec *r, 00167 vfs_dir_t **new, const char *dirname, 00168 apr_pool_t *pool); 00169 apr_status_t (*dir_read) (const vfs_mount *mnt, apr_finfo_t *finfo, 00170 apr_int32_t wanted, vfs_dir_t *thedir); 00171 apr_status_t (*dir_close) (const vfs_mount *mnt, vfs_dir_t *thedir); 00172 apr_status_t (*dir_make) (const vfs_mount *mnt, const request_rec *r, 00173 const char *path, apr_fileperms_t perm, 00174 apr_pool_t *pool); 00175 apr_status_t (*dir_remove) (const vfs_mount *mnt, const request_rec *r, 00176 const char *path, apr_pool_t *pool); 00177 }; 00178 00179 /* VFS file type */ 00180 00181 struct vfs_file_t { 00182 vfs_mount *mnt; 00183 void *userdata; 00184 }; 00185 00186 /* VFS directory type */ 00187 00188 struct vfs_dir_t { 00189 vfs_mount *mnt; 00190 void *userdata; 00191 }; 00192 00193 /* linked list of vfs_mount structures */ 00194 00195 extern vfs_mount *ap_vfs_mount_head; 00196 #else 00197 00198 #define VFS_IRWXU 1 00199 #define VFS_IRWXG 2 00200 #define VFS_IRWXO 4 00201 #define ssize_t int 00202 #define loff_t int 00203 #define filldir_t int 00204 #define VFS_ISDIR(x) ( x & 1) // ((x) && (x) type == VFS_DIR) //! Macro to know if a given entry represents a directory 00205 #define VFS_ISREG( x ) ( x & 2) 00206 00207 #define vfs_t int 00208 typedef struct 00209 { 00210 int st_size; 00211 time_t st_mtime; 00212 int st_mode; 00213 } vfs_stat_t; 00214 00215 typedef struct 00216 { 00217 char *name; 00218 } vfs_dirent_t; 00219 00220 00221 /* VFS file type */ 00222 00223 struct vfs_file_t { 00224 vfs_mount *mnt; 00225 void *userdata; 00226 }; 00227 00228 /* VFS directory type */ 00229 00230 struct vfs_dir_t { 00231 vfs_mount *mnt; 00232 void *userdata; 00233 }; 00234 00235 int *vfs_openfs( void ); 00236 vfs_file_t *vfs_open( int *fd, const char *arg, const char *mode ); 00237 ssize_t vfs_read( void *buf,int what, int BufferSize, vfs_file_t *fp ); 00238 int vfs_write( void *buf,int what, int BufferSize, vfs_file_t *fp ); 00239 int vfs_eof( vfs_file_t *fp ); 00240 int vfs_close( vfs_file_t *fp ); 00241 int vfs_stat( int *fd, const char *fname, vfs_stat_t *st ); 00242 char *vfs_getcwd( int *fd, void *x, int y ); 00243 vfs_dir_t *vfs_opendir( int *fd, char *cwd ); 00244 vfs_dirent_t *vfs_readdir( vfs_dir_t *fd ); 00245 int vfs_closedir( vfs_dir_t *fd ); 00246 int vfs_mkdir( int *fd, const char *arg, int mode ); 00247 int vfs_rmdir( int *fd, const char *arg ); 00248 int vfs_rename( int *fd, char *was, const char *arg ); 00249 int vfs_remove( int *fd, const char *arg ); 00250 int vfs_chdir( int *fd, const char *arg ); 00251 00252 00253 //#define vfs_eof( a ) 00254 //#define vfs_close( a ) fclose( a ) 00255 //#define vfs_readdir( a ) readdir( a ) 00256 //#define vfs_stat( a, b, c ) fstat( a, b ) 00257 //#define vfs_closedir( a ) closedir( a ) 00258 00259 #endif // DRB 00260 00261 #endif /* AP_USE_VFS */ 00262 00263 #endif /* APACHE_VFS_H */
Generated on Tue Jul 12 2022 22:52:20 by
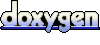