
Snake vs Block Game to be run upon K64F.
Snake Class Reference
#include <Snake.h>
Public Member Functions | |
Snake () | |
Constructor. | |
~Snake () | |
Destructor. | |
void | init (N5110 *lcd) |
Initialises the snakes position struct and reset variable and gets pointers of lcd from int main() to be used privately in the entire class. | |
void | _setLength (int length) |
This mutator sets the length of the snake directly when called. | |
void | _setSpeed (int speed) |
This mutator sets the speed of the snake directly when called. | |
void | draw () |
This function draws the Snake sprite onto the screen at the specified coordinates. | |
void | set_pos (Vector2D p) |
This function sets a position for where the snake should spawn. | |
void | update (Direction d, int *immobile_bead_n) |
This function updates the Snake sprite position on screen. | |
void | chainSnakeTogether (int *immobile_bead_n) |
This function makes all of the snake beeds chained together by making the lower ones drag towards where the top one was in the previous loop. | |
void | moveSnake (int *immobile_bead_n) |
This function makes the controls of W/E directions only exclusive to the top beed in the snake. | |
void | _setSnakeLimits () |
This function makes sure that the snake stays where it is when it ate food and does not travel off the screen. | |
Vector2D | get_pos (int snakeIndex) |
This function returns the coordinate of the top-left pixel in the Snake sprite of each bead which is reffered to by snakeIndex. |
Detailed Description
Snake Class.
This class draws and updates snake after every collision, and creates perfect animations of the snake moving.
- Date:
- 9th May 2019
Definition at line 13 of file Snake.h.
Constructor & Destructor Documentation
Member Function Documentation
void _setLength | ( | int | length ) |
void _setSnakeLimits | ( | ) |
void _setSpeed | ( | int | speed ) |
void chainSnakeTogether | ( | int * | immobile_bead_n ) |
This function makes all of the snake beeds chained together by making the lower ones drag towards where the top one was in the previous loop.
- Parameters:
-
immobile_bead_n[10] this array is indexed by the beed number of the colliding snake, if immobile_bead_n[2] = 1 the particular bead can move freely.
void draw | ( | ) |
Vector2D get_pos | ( | int | snakeIndex ) |
This function returns the coordinate of the top-left pixel in the Snake sprite of each bead which is reffered to by snakeIndex.
- Parameters:
-
int snakeIndex
This is the index of the snake bead of which the position needs to be returned.
- Returns:
- Vector2D snakepos
This is a struct that returns the x and y position of the snake bead that is being referred to by the snakeIndex.
void init | ( | N5110 * | lcd ) |
Initialises the snakes position struct and reset variable and gets pointers of lcd from int main() to be used privately in the entire class.
- Parameters:
-
N5110 *lcd
pointer to the N5110 object in main, address of this pointer is saved to make availability to the entire class, without passing address to each function.
void moveSnake | ( | int * | immobile_bead_n ) |
void set_pos | ( | Vector2D | p ) |
void update | ( | Direction | d, |
int * | immobile_bead_n | ||
) |
This function updates the Snake sprite position on screen.
- Parameters:
-
Direction d
This struct saves the direction of snake movement.
- Parameters:
-
immobile_bead_n[10] this array is indexed by the beed number of the colliding snake, if immobile_bead_n[2] = 1 the particular bead can move freely.
Generated on Wed Jul 13 2022 04:14:35 by
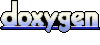