Address is changed to suit Grove Digital Light Sensor.
Fork of TSL2561_I2C by
TSL2561_I2C.h
00001 #ifndef TSL2561_I2C_H 00002 #define TSL2561_I2C_H 00003 #include "mbed.h" 00004 00005 //Defines 00006 #define TSL_SLAVE_ADDRESS 0x29 00007 00008 /* 00009 #define TSL_CONTROL 0x00 00010 #define TSL_TIMING 0x01 00011 #define TSL_THRESHLOWLOW 0x02 00012 #define TSL_THRESHHIGHLOW 0x04 00013 #define TSL_INTERRUPT 0x06 00014 #define TSL_ID 0x0A 00015 #define TSL_DATA0LOW 0x0C 00016 #define TSL_DATA1LOW 0x0E 00017 */ 00018 00019 #define TSL_CONTROL 0x80 00020 #define TSL_TIMING 0x81 00021 #define TSL_THRESHLOWLOW 0x06 00022 #define TSL_THRESHHIGHLOW 0x04 00023 #define TSL_INTERRUPT 0x86 00024 #define TSL_ID 0x0A 00025 #define TSL_DATA0LOW 0x8C 00026 #define TSL_DATA1LOW 0x8E 00027 00028 /** TSL2561_I2C class. 00029 * Abstraction for TAOS TSL2561 Light-To-Digital Converter. 00030 * 00031 * Example: 00032 * @code 00033 * #include "mbed.h" 00034 * #include "TSL2561_I2C.h" 00035 * 00036 * TSL2561_I2C lum_sensor( p9, p10 ); 00037 * 00038 * int main() { 00039 * lum_sensor.enablePower(); 00040 * 00041 * int rgb_readings[4]; 00042 * while(1) { 00043 * printf( "Luminosity: %4.2f\n", lum_sensor.getLux() ); 00044 * wait_ms( 100 ); 00045 * } 00046 * } 00047 * @endcode 00048 */ 00049 class TSL2561_I2C { 00050 public: 00051 /** Create TSL2561_I2C instance 00052 * 00053 * @param sda sda pin for I2C 00054 * @param scl scl pin for I2C 00055 */ 00056 TSL2561_I2C( PinName sda, PinName scl ); 00057 00058 /** Read broadband photodiode (visible plus infrared) 00059 * 00060 * @returns 00061 * Irradiance measured 00062 */ 00063 int getVisibleAndIR(); 00064 00065 /** Read infrared-responding photodiode 00066 * 00067 * @returns 00068 * Irradiance measured 00069 */ 00070 int getIROnly(); 00071 00072 /** Read sensors and calculate Illuminance in lux 00073 * 00074 * @returns 00075 * Illuminance (lux) 00076 */ 00077 float getLux(); 00078 00079 /** Power up the device. 00080 * 00081 * @returns 00082 * 1 if successful 00083 * 0 if otherwise 00084 */ 00085 int enablePower(); 00086 00087 /** Disable power to the device. 00088 * 00089 * @returns 00090 * 1 if successful 00091 * 0 if otherwise 00092 */ 00093 int disablePower(); 00094 00095 /** Check if power to the device is enabled. 00096 * 00097 * @returns 00098 * 1 if power ON 00099 * 0 if power OFF 00100 */ 00101 bool isPowerEnabled(); 00102 00103 /** Return present gain value 00104 * 00105 * @returns 00106 * 1 (low gain mode) 00107 * 16 (high gain mode) 00108 */ 00109 int readGain(); 00110 00111 /** Set gain 00112 * 00113 * @param gain Gain must be either 1 or 16 00114 * 00115 * @returns 00116 * 1 if successful 00117 * 0 if otherwise 00118 */ 00119 int setGain( const int gain ); 00120 00121 /** Read the current integration time. 00122 * 00123 * @returns 00124 * Integration time in milliseconds 00125 */ 00126 float readIntegrationTime(); 00127 00128 /** Set integration time. 00129 * 00130 * @param itime Integration time to set in milliseconds. Should be 13.7, 101 or 402. 00131 * 00132 * @returns 00133 * 1 if successful 00134 * 0 if otherwise 00135 */ 00136 int setIntegrationTime( const float itime ); 00137 00138 /** Read the low trigger point for the comparison function for interrupt generation. 00139 * 00140 * @returns 00141 * Low threshold value 00142 */ 00143 int readLowInterruptThreshold(); 00144 00145 /** Read the high trigger point for the comparison function for interrupt generation. 00146 * 00147 * @returns 00148 * High threshold value 00149 */ 00150 int readHighInterruptThreshold(); 00151 00152 /** Set the low trigger point for the comparison function for interrupt generation. 00153 * 00154 * @param threshold Low threshold value 00155 * 00156 * @returns 00157 * 1 if successful 00158 * 0 if otherwise 00159 */ 00160 int setLowInterruptThreshold( const int threshold ); 00161 00162 /** Set the high trigger point for the comparison function for interrupt generation. 00163 * 00164 * @param threshold High threshold value 00165 * 00166 * @returns 00167 * 1 if successful 00168 * 0 if otherwise 00169 */ 00170 int setHighInterruptThreshold( const int threshold ); 00171 00172 /** Return the number of consecutive values out of range (set by the low and high thresholds) required to trigger an interrupt 00173 * 00174 * @returns 00175 * interrput persistence 00176 */ 00177 int readInterruptPersistence(); 00178 00179 /** Set the number of consecutive values out of range (set by the low and high thresholds) required to trigger an interrupt 00180 * 00181 * @param persistence Value to set. 0: interrupt every ADC cycle, 1-15: corresponding number of cycles until interrupt 00182 * 00183 * @returns 00184 * 1 if successful 00185 * 0 if otherwise 00186 */ 00187 int setInterruptPersistence( const int persistence ); 00188 00189 /** Check the interrupt function's operation mode 00190 * 00191 * @returns 00192 * 0: Interrupt output disabled 00193 * 1: Level Interrupt 00194 * 2: SMBAlert compliant 00195 * 3: Test Mode 00196 */ 00197 int readInterruptControl(); 00198 00199 /** Set the interrupt function's operation mode 00200 * 00201 * @param control Value to set. 0: interrupt every ADC cycle, 1-15: corresponding number of cycles until interrupt 00202 * 00203 * @returns 00204 * 1 if successful 00205 * 0 if otherwise 00206 */ 00207 int setInterruptControl( const int control ); 00208 00209 /** Clear the interrupt, allowing normal operation to resume. 00210 * (writes 0b11000000 to command register to clear interrupt) 00211 * 00212 * @returns 00213 * 1 if successful 00214 * 0 if otherwise 00215 */ 00216 int clearInterrupt(); 00217 00218 /** Get integer indicating part number 00219 * 00220 * @returns 00221 * 0: TSL2560 00222 * 1: TSL2561 00223 */ 00224 int getPartNumber(); 00225 00226 /** Get revision number 00227 * 00228 * @returns 00229 * Revision number 00230 */ 00231 int getRevisionNumber(); 00232 00233 private: 00234 I2C i2c; 00235 00236 int writeSingleRegister( char address, char data ); 00237 int writeMultipleRegisters( char address, char* data, int quantity ); 00238 char readSingleRegister( char address ); 00239 int readMultipleRegisters( char address, char* output, int quantity ); 00240 }; 00241 00242 #endif
Generated on Wed Jul 20 2022 06:42:06 by
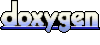