A program designed to run on the microbit. Used for driving a buggy.
Embed:
(wiki syntax)
Show/hide line numbers
Microbit_function.cpp
00001 00002 #include "Microbit_function.h" 00003 #include "mbed.h" 00004 00005 MicroBit uBit; 00006 00007 // These MiroBit images are used by the Display function to indicate what direction the buggy will travel in. 00008 MicroBitImage arrow_Up("0,0,1,0,0\n0,1,1,1,0\n1,0,1,0,1\n0,0,1,0,0\n0,0,1,0,0\n"); 00009 MicroBitImage arrow_Down("0,0,1,0,0\n0,0,1,0,0\n1,0,1,0,1\n0,1,1,1,0\n0,0,1,0,0\n"); 00010 MicroBitImage arrow_Left("0,0,1,0,0\n0,1,0,0,0\n1,1,1,1,1\n0,1,0,0,0\n0,0,1,0,0\n"); 00011 MicroBitImage arrow_Right("0,0,1,0,0\n0,0,0,1,0\n1,1,1,1,1\n0,0,0,1,0\n0,0,1,0,0\n"); 00012 MicroBitImage arrow_TopLeft("1,1,1,0,0\n1,1,0,0,0\n1,0,1,0,0\n0,0,0,1,0\n0,0,0,0,1\n"); 00013 MicroBitImage arrow_TopRight("0,0,1,1,1\n0,0,0,1,1\n0,0,1,0,1\n0,1,0,0,0\n1,0,0,0,0\n"); 00014 MicroBitImage arrow_BottomLeft("0,0,0,0,1\n0,0,0,1,0\n1,0,1,0,0\n1,1,0,0,0\n1,1,1,0,0\n"); 00015 MicroBitImage arrow_BottomRight("1,0,0,0,0\n0,1,0,0,0\n0,0,1,0,1\n0,0,0,1,1\n0,0,1,1,1\n"); 00016 MicroBitImage cross("1,0,0,0,1\n0,1,0,1,0\n0,0,1,0,0\n0,1,0,1,0\n1,0,0,0,1\n"); 00017 MicroBitImage On("1,1,1,1,1\n1,1,1,1,1\n1,1,1,1,1\n1,1,1,1,1\n1,1,1,1,1\n"); 00018 00019 // 00020 MicroBitPin P0(MICROBIT_ID_IO_P0, MICROBIT_PIN_P0, PIN_CAPABILITY_ALL); 00021 MicroBitPin P1(MICROBIT_ID_IO_P1, MICROBIT_PIN_P1, PIN_CAPABILITY_ALL); 00022 00023 extern void microBit_Setup() 00024 { 00025 uBit.init(); 00026 } 00027 00028 extern void Display(Direction Current_Direction) // This function is used to simply display the direction the motors are turning 00029 { 00030 switch(Current_Direction) // Displays the related arrow accoring to which direction the buggy is moving in. 00031 { 00032 case Up: uBit.display.printAsync(arrow_Up); break; 00033 case Down: uBit.display.printAsync(arrow_Down); break; 00034 case Right: uBit.display.printAsync(arrow_Right); break; 00035 case Left: uBit.display.printAsync(arrow_Left); break; 00036 case TopLeft: uBit.display.printAsync(arrow_TopLeft); break; 00037 case BottomLeft: uBit.display.printAsync(arrow_BottomLeft); break; 00038 case TopRight: uBit.display.printAsync(arrow_TopRight); break; 00039 case BottomRight: uBit.display.printAsync(arrow_BottomRight); break; 00040 case Stop: uBit.display.printAsync(cross); break; 00041 case Clear: uBit.display.clear(); break; 00042 } 00043 } 00044 extern void pointNorth() //This function Will direct the buggy to face North 00045 { 00046 int direction = uBit.compass.heading(); //Declare a variable to store the direction in 00047 while((direction > 2) && (direction < 358)) // While it is not facing north 00048 { 00049 if (direction <= 180) // if it is less then 180 then turn left 00050 { 00051 left(0.1); 00052 } 00053 if (direction > 180) // if it is more then 180 then turn right 00054 { 00055 right(0.1); 00056 } 00057 direction = uBit.compass.heading(); // check the direction again 00058 } 00059 } 00060 00061 extern void pointSouth() // this function is similar to hedNorth but will point to South instead 00062 { 00063 int direction = uBit.compass.heading(); //Declares a variable which will be used to store the direction 00064 while((direction < 178) || (direction > 182)) //While it is not pointing South 00065 { 00066 if ((direction > 180) && (direction <= 360)) // if it is between 180 and 360 turn left. 00067 { 00068 left(0.1); 00069 } 00070 if ((direction > 0) && (direction <=180)) // if it is less than 180 turn right. 00071 { 00072 right(0.1); 00073 } 00074 direction = uBit.compass.heading(); // check the direction again 00075 } 00076 } 00077 00078 extern Compass_Dirs Compass() // this function is designed to display the direction the microbit is facing, and returns that value. 00079 { 00080 int direction = uBit.compass.heading(); // declare a variable to be used for storing the direction. 00081 if((direction < 45) || (direction >= 325)) // if the direciton is pointing generally north then output North. 00082 { 00083 uBit.display.print("N"); 00084 return North; 00085 } 00086 else if((direction < 135) && (direction >= 45)) // if the direction is generally east then output east. 00087 { 00088 uBit.display.print("E"); 00089 return East; 00090 } 00091 else if((direction < 225) && (direction >= 135)) // if the direction is generally South then output south 00092 { 00093 uBit.display.print("S"); 00094 return South; 00095 } 00096 else if((direction < 325) && (direction >= 225)) // if the direction is generally West then output West. 00097 { 00098 uBit.display.print("W"); 00099 return West; 00100 } 00101 return Default; 00102 } 00103 00104 extern int left_Sensor()// This function returns the analogue voltage on the left sensor. 00105 { 00106 return P0.getAnalogValue(); //This is the function that actually get the voltage. 00107 } 00108 00109 extern int right_Sensor() //This function returns the analogue voltage of the right sensor. 00110 { 00111 return P1.getAnalogValue(); //gets the voltage on P1, should be connected to the right sensor. 00112 }
Generated on Fri Jul 15 2022 13:57:07 by
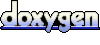