
Use a Sparkfun Blackberry Trackball Breakout Board as a mouse.
Dependencies: mbed BBTrackball
acceleration.h
00001 /* Copyright 2011 Adam Green (http://mbed.org/users/AdamGreen/) 00002 00003 Licensed under the Apache License, Version 2.0 (the "License"); 00004 you may not use this file except in compliance with the License. 00005 You may obtain a copy of the License at 00006 00007 http://www.apache.org/licenses/LICENSE-2.0 00008 00009 Unless required by applicable law or agreed to in writing, software 00010 distributed under the License is distributed on an "AS IS" BASIS, 00011 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 See the License for the specific language governing permissions and 00013 limitations under the License. 00014 */ 00015 /* Classes to apply acceleration to input from Sparkfun's Blackberry Trackball 00016 Breakout board. 00017 */ 00018 #ifndef _ACCELERATION_H_ 00019 #define _ACCELERATION_H_ 00020 00021 #include <mbed.h> 00022 #include <limits.h> 00023 #include "BBTrackball.h" 00024 00025 00026 00027 /* Class which can apply acceleration to each of the roller inputs from the 00028 Sparkfun Blackberry Trackball device. 00029 */ 00030 template<size_t SampleCount, 00031 int DecelerateThreshold, 00032 int AccelerateThreshold, 00033 int MaxAcceleration> 00034 class CAcceleratedRoller 00035 { 00036 public: 00037 CAcceleratedRoller(void) 00038 { 00039 m_Acceleration = 1; 00040 m_VelocitySum = 0; 00041 m_SampleIndex = 0; 00042 memset(m_Samples, 0, sizeof(m_Samples)); 00043 } 00044 00045 void AccumulateSample(short CurrVelocity); 00046 int Acceleration(void) const 00047 { 00048 return m_Acceleration; 00049 } 00050 00051 protected: 00052 unsigned char ClampToUChar(short Value); 00053 int m_Acceleration; 00054 int m_VelocitySum; 00055 size_t m_SampleIndex; 00056 unsigned char m_Samples[SampleCount]; 00057 }; 00058 00059 00060 class CAcceleratedTrackball : public CBBTrackball 00061 { 00062 public: 00063 CAcceleratedTrackball(PinName BluePin, 00064 PinName RedPin, 00065 PinName GreenPin, 00066 PinName WhitePin, 00067 PinName UpPin, 00068 PinName DownPin, 00069 PinName LeftPin, 00070 PinName RightPin, 00071 PinName ButtonPin) : CBBTrackball(BluePin, 00072 RedPin, 00073 GreenPin, 00074 WhitePin, 00075 UpPin, 00076 DownPin, 00077 LeftPin, 00078 RightPin, 00079 ButtonPin), 00080 m_IterationsSinceLastMotion(INT_MAX) 00081 { 00082 } 00083 void GetState(int& DeltaX, int& DeltaY, int& ButtonPressed); 00084 00085 protected: 00086 void UpdateLEDColourBasedOnMotion(int VelocityX, 00087 int VelocityY, 00088 int ButtonPressed); 00089 00090 int m_IterationsSinceLastMotion; 00091 CAcceleratedRoller<250, 4, 4, 40> m_UpAcceleration; 00092 CAcceleratedRoller<250, 4, 4, 40> m_DownAcceleration; 00093 CAcceleratedRoller<250, 4, 4, 30> m_LeftAcceleration; 00094 CAcceleratedRoller<250, 4, 4, 30> m_RightAcceleration; 00095 }; 00096 00097 #endif // _ACCELERATION_H_
Generated on Fri Jul 15 2022 14:34:39 by
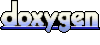