Fixed a few minor issues in 4DGL library Moved pc serial port object used for debug output into 4DGL object itself. Previously it was a global object but its constructor wasn't getting run before the global constructor for the 4DGL object itself which led to an assert in the serial code. I also fixed a few potential buffer overruns that I saw in the code as well.
TFT_4DGL_Graphics.cpp
00001 // 00002 // TFT_4DGL is a class to drive 4D Systems TFT touch screens 00003 // 00004 // Copyright (C) <2010> Stephane ROCHON <stephane.rochon at free.fr> 00005 // 00006 // TFT_4DGL is free software: you can redistribute it and/or modify 00007 // it under the terms of the GNU General Public License as published by 00008 // the Free Software Foundation, either version 3 of the License, or 00009 // (at your option) any later version. 00010 // 00011 // TFT_4DGL is distributed in the hope that it will be useful, 00012 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 // GNU General Public License for more details. 00015 // 00016 // You should have received a copy of the GNU General Public License 00017 // along with TFT_4DGL. If not, see <http://www.gnu.org/licenses/>. 00018 00019 #include "mbed.h" 00020 #include "TFT_4DGL.h" 00021 00022 #define ARRAY_SIZE(X) sizeof(X)/sizeof(X[0]) 00023 00024 //**************************************************************************************************** 00025 void TFT_4DGL :: circle(int x, int y , int radius, int color) { // draw a circle in (x,y) 00026 char command[9]= ""; 00027 00028 command[0] = CIRCLE; 00029 00030 command[1] = (x >> 8) & 0xFF; 00031 command[2] = x & 0xFF; 00032 00033 command[3] = (y >> 8) & 0xFF; 00034 command[4] = y & 0xFF; 00035 00036 command[5] = (radius >> 8) & 0xFF; 00037 command[6] = radius & 0xFF; 00038 00039 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00040 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00041 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00042 00043 command[7] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00044 command[8] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00045 00046 writeCOMMAND(command, 9); 00047 } 00048 00049 //**************************************************************************************************** 00050 void TFT_4DGL :: triangle(int x1, int y1 , int x2, int y2, int x3, int y3, int color) { // draw a traingle 00051 char command[15]= ""; 00052 00053 command[0] = TRIANGLE; 00054 00055 command[1] = (x1 >> 8) & 0xFF; 00056 command[2] = x1 & 0xFF; 00057 00058 command[3] = (y1 >> 8) & 0xFF; 00059 command[4] = y1 & 0xFF; 00060 00061 command[5] = (x2 >> 8) & 0xFF; 00062 command[6] = x2 & 0xFF; 00063 00064 command[7] = (y2 >> 8) & 0xFF; 00065 command[8] = y2 & 0xFF; 00066 00067 command[9] = (x3 >> 8) & 0xFF; 00068 command[10] = x3 & 0xFF; 00069 00070 command[11] = (y3 >> 8) & 0xFF; 00071 command[12] = y3 & 0xFF; 00072 00073 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00074 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00075 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00076 00077 command[13] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00078 command[14] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00079 00080 writeCOMMAND(command, 15); 00081 } 00082 00083 //**************************************************************************************************** 00084 void TFT_4DGL :: line(int x1, int y1 , int x2, int y2, int color) { // draw a line 00085 char command[11]= ""; 00086 00087 command[0] = LINE; 00088 00089 command[1] = (x1 >> 8) & 0xFF; 00090 command[2] = x1 & 0xFF; 00091 00092 command[3] = (y1 >> 8) & 0xFF; 00093 command[4] = y1 & 0xFF; 00094 00095 command[5] = (x2 >> 8) & 0xFF; 00096 command[6] = x2 & 0xFF; 00097 00098 command[7] = (y2 >> 8) & 0xFF; 00099 command[8] = y2 & 0xFF; 00100 00101 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00102 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00103 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00104 00105 command[9] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00106 command[10] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00107 00108 writeCOMMAND(command, 11); 00109 } 00110 00111 //**************************************************************************************************** 00112 void TFT_4DGL :: rectangle(int x1, int y1 , int x2, int y2, int color) { // draw a rectangle 00113 char command[11]= ""; 00114 00115 command[0] = RECTANGLE; 00116 00117 command[1] = (x1 >> 8) & 0xFF; 00118 command[2] = x1 & 0xFF; 00119 00120 command[3] = (y1 >> 8) & 0xFF; 00121 command[4] = y1 & 0xFF; 00122 00123 command[5] = (x2 >> 8) & 0xFF; 00124 command[6] = x2 & 0xFF; 00125 00126 command[7] = (y2 >> 8) & 0xFF; 00127 command[8] = y2 & 0xFF; 00128 00129 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00130 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00131 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00132 00133 command[9] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00134 command[10] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00135 00136 writeCOMMAND(command, 11); 00137 } 00138 00139 //**************************************************************************************************** 00140 void TFT_4DGL :: ellipse(int x, int y , int radius_x, int radius_y, int color) { // draw an ellipse 00141 char command[11]= ""; 00142 00143 command[0] = ELLIPSE; 00144 00145 command[1] = (x >> 8) & 0xFF; 00146 command[2] = x & 0xFF; 00147 00148 command[3] = (y >> 8) & 0xFF; 00149 command[4] = y & 0xFF; 00150 00151 command[5] = (radius_x >> 8) & 0xFF; 00152 command[6] = radius_x & 0xFF; 00153 00154 command[7] = (radius_y >> 8) & 0xFF; 00155 command[8] = radius_y & 0xFF; 00156 00157 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00158 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00159 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00160 00161 command[9] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00162 command[10] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00163 00164 writeCOMMAND(command, 11); 00165 } 00166 00167 //**************************************************************************************************** 00168 void TFT_4DGL :: pixel(int x, int y, int color) { // draw a pixel 00169 char command[7]= ""; 00170 00171 command[0] = PIXEL; 00172 00173 command[1] = (x >> 8) & 0xFF; 00174 command[2] = x & 0xFF; 00175 00176 command[3] = (y >> 8) & 0xFF; 00177 command[4] = y & 0xFF; 00178 00179 int red5 = (color >> (16 + 3)) & 0x1F; // get red on 5 bits 00180 int green6 = (color >> (8 + 2)) & 0x3F; // get green on 6 bits 00181 int blue5 = (color >> (0 + 3)) & 0x1F; // get blue on 5 bits 00182 00183 command[5] = ((red5 << 3) + (green6 >> 3)) & 0xFF; // first part of 16 bits color 00184 command[6] = ((green6 << 5) + (blue5 >> 0)) & 0xFF; // second part of 16 bits color 00185 00186 writeCOMMAND(command, 7); 00187 } 00188 00189 //****************************************************************************************************** 00190 int TFT_4DGL :: read_pixel(int x, int y) { // read screen info and populate data 00191 00192 char command[5]= ""; 00193 00194 command[0] = READPIXEL; 00195 00196 command[1] = (x >> 8) & 0xFF; 00197 command[2] = x & 0xFF; 00198 00199 command[3] = (y >> 8) & 0xFF; 00200 command[4] = y & 0xFF; 00201 00202 int i, temp = 0, color = 0, resp = 0; 00203 char response[2] = ""; 00204 00205 freeBUFFER(); 00206 00207 for (i = 0; i < 5; i++) { // send all chars to serial port 00208 writeBYTE(command[i]); 00209 } 00210 00211 while (!_cmd.readable()) wait_ms(TEMPO); // wait for screen answer 00212 00213 while (_cmd.readable() && resp < ARRAY_SIZE(response)) { 00214 temp = _cmd.getc(); 00215 response[resp++] = (char)temp; 00216 } 00217 00218 color = ((response[0] << 8) + response[1]); 00219 00220 return color; // WARNING : this is 16bits color, not 24bits... need to be fixed 00221 } 00222 00223 //****************************************************************************************************** 00224 void TFT_4DGL :: screen_copy(int xs, int ys , int xd, int yd , int width, int height) { 00225 00226 char command[13]= ""; 00227 00228 command[0] = SCREENCOPY; 00229 00230 command[1] = (xs >> 8) & 0xFF; 00231 command[2] = xs & 0xFF; 00232 00233 command[3] = (ys >> 8) & 0xFF; 00234 command[4] = ys & 0xFF; 00235 00236 command[5] = (xd >> 8) & 0xFF; 00237 command[6] = xd & 0xFF; 00238 00239 command[7] = (yd >> 8) & 0xFF; 00240 command[8] = yd & 0xFF; 00241 00242 command[9] = (width >> 8) & 0xFF; 00243 command[10] = width & 0xFF; 00244 00245 command[11] = (height >> 8) & 0xFF; 00246 command[12] = height & 0xFF; 00247 00248 writeCOMMAND(command, 13); 00249 } 00250 00251 //**************************************************************************************************** 00252 void TFT_4DGL :: pen_size(char mode) { // set pen to SOLID or WIREFRAME 00253 char command[2]= ""; 00254 00255 command[0] = PENSIZE; 00256 command[1] = mode; 00257 00258 writeCOMMAND(command, 2); 00259 }
Generated on Thu Jul 14 2022 21:04:09 by
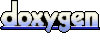