
Test, please delete
ADISENSE Host Library API
Detailed Description
Host library API common to the ADISENSE product family.
Typedef Documentation
typedef void* ADI_SENSE_DEVICE_HANDLE |
A handle used in all API functions to identify the ADISENSE device.
Definition at line 68 of file adi_sense_api.h.
Enumeration Type Documentation
Bit masks (flags) for the different channel alert indicators.
- Enumerator:
Definition at line 125 of file adi_sense_api.h.
Supported connection types for communication with the ADISENSE device.
Definition at line 71 of file adi_sense_api.h.
Bit masks (flags) for the different device status indicators.
- Enumerator:
Definition at line 89 of file adi_sense_api.h.
Bit masks (flags) for the different diagnostics status indicators.
- Enumerator:
Definition at line 109 of file adi_sense_api.h.
Measurement mode options for the ADISENSE device. adi_sense_StartMeasurement
- Enumerator:
Definition at line 190 of file adi_sense_api.h.
enum ADI_SENSE_PRODUCT_ID |
A list of supported product identifiers
Definition at line 62 of file adi_sense_config_types.h.
Identifiers for the user configuration slots in persistent memory.
Definition at line 207 of file adi_sense_api.h.
Function Documentation
ADI_SENSE_RESULT adi_sense_ApplyConfigUpdates | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice ) |
Apply the configuration settings currently stored in device registers.
- Parameters:
-
[in] hDevice ADISENSE device context handle
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Instructs the ADISENSE device to reload and apply configuration from the device configuration registers. Changes to configuration registers are ignored by the device until this function is called.
- Note:
- No other command must be running when this is called.
Definition at line 483 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_Close | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice ) |
Close ADISENSE device context and free resources.
- Parameters:
-
[in] hDevice ADISENSE device context handle
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Definition at line 862 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_EraseExternalFlash | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice ) |
Erases the external flash memory.
- Parameters:
-
[in] hDevice ADISENSE device context handle
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Sends the bulk erase instruction to the external flash device. All stored samples are deleted. It is a blocking operation and takes tens of seconds to complete.
- Note:
- No other command must be running when this is called.
Definition at line 578 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_GetCommandRunningState | ( | ADI_SENSE_DEVICE_HANDLE | hDevice, |
bool_t * | pbCommandRunning | ||
) |
Check if a command is currently running on the device.
- Parameters:
-
[in] hDevice ADISENSE device context handle [out] pbCommandRunning Pointer to return the command running status
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Reads the device status register to check if a command is running.
Definition at line 419 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_GetData | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice, |
ADI_SENSE_MEASUREMENT_MODE const | eMeasurementMode, | ||
ADI_SENSE_DATA_SAMPLE *const | pSamples, | ||
uint8_t const | nBytesPerSample, | ||
uint32_t const | nRequested, | ||
uint32_t *const | pnReturned | ||
) |
Read measurement data samples from the device registers.
- Parameters:
-
[in] hDevice ADISENSE device context handle [in] eMeasurementMode Must be set to the same value used for adi_sense_StartMeasurement(). [out] pSamples Pointer to return a set of requested data samples. [in] nBytesPerSample The size, in bytes, of each sample. [in] nRequested Number of requested data samples. [out] pnReturned Number of valid data samples successfully retrieved.
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Reads the status registers and extracts the relevant information to return to the caller.
- Note:
- This is intended to be called only when the DATAREADY status signal is asserted.
Definition at line 768 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_GetDeviceReadyState | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice, |
bool_t *const | pbReady | ||
) |
Check if the device is ready, following power-up or a reset.
- Parameters:
-
[in] hDevice ADISENSE device context handle [out] pbReady Pointer to return true if the device is ready, or false otherwise
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
This function attempts to read a fixed-value device register via the communication interface.
Definition at line 971 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_GetExternalFlashData | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice, |
ADI_SENSE_DATA_SAMPLE *const | pSamples, | ||
uint32_t const | nIndex, | ||
uint32_t const | nRequested, | ||
uint32_t *const | pnReturned | ||
) |
Read measurement samples stored in the the external flash memory.
- Parameters:
-
[in] hDevice ADISENSE device context handle [out] pSamples Pointer to return a set of requested data samples. [in] nStartIndex Index of first sample to retrieve. [in] nBytesPerSample The size, in bytes, of each sample. [in] nRequested Number of requested data samples. [out] pnReturned Number of valid data samples successfully retrieved.
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Reads the status registers and extracts the relevant information to return to the caller.
Definition at line 615 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_GetExternalFlashSampleCount | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice, |
uint32_t * | nSampleCount | ||
) |
Gets the number of samples stored in the external flash memory.
- Parameters:
-
[in] hDevice ADISENSE device context handle [in] pSampleCount Address of the return value.
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
- Note:
- No other command must be running when this is called.
Definition at line 588 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_GetGpioState | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice, |
ADI_SENSE_GPIO_PIN const | ePinId, | ||
bool_t *const | pbAsserted | ||
) |
Get the current state of the specified GPIO input signal.
- Parameters:
-
[in] hDevice ADISENSE device context handle [in] ePinId GPIO pin to query [out] pbAsserted Pointer to return the state of the status signal GPIO pin
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
- ADI_SENSE_INVALID_DEVICE_NUM Invalid GPIO pin specified.
Sets *pbAsserted to true if the status signal is asserted, or false otherwise.
Definition at line 248 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_GetProductID | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice, |
ADI_SENSE_PRODUCT_ID *const | pProductId | ||
) |
Obtain the product ID from the device.
- Parameters:
-
[in] hDevice ADISENSE device context handle [out] pProductId Pointer to return the product ID value
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Reads the product ID registers on the device and returns the value.
Definition at line 1130 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_GetStatus | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice, |
ADI_SENSE_STATUS *const | pStatus | ||
) |
Read the current status from the device registers.
- Parameters:
-
[in] hDevice ADISENSE device context handle [out] pStatus Pointer to return the status summary obtained from the device.
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Reads the status registers and extracts the relevant information to return to the caller.
- Note:
- This may be called at any time, assuming the device is ready.
Read the current status from the device registers.
- Parameters:
-
[in] @param[out] pStatus : Pointer to CORE Status struct.
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
- ADI_SENSE_FAILURE If status register read fails.
Read the general status register for the ADISense module. Indicates Error, Alert conditions, data ready and command running.
Definition at line 316 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_Open | ( | unsigned const | nDeviceIndex, |
ADI_SENSE_CONNECTION *const | pConnectionInfo, | ||
ADI_SENSE_DEVICE_HANDLE *const | phDevice | ||
) |
Open ADISENSE device handle and set up communication interface.
- Parameters:
-
[in] nDeviceIndex Zero-based index number identifying this device instance. Note that this will be used to retrieve a specific device configuration for this device (see adi_sense_SetConfig and ADI_SENSE_CONFIG) [in] pConnectionInfo Host-specific connection details (e.g. SPI, GPIO) [out] phDevice Pointer to return an ADISENSE device handle
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
- ADI_SENSE_NO_MEM Failed to allocate memory resources.
- ADI_SENSE_INVALID_DEVICE_NUM Invalid device index specified
Configure and initialise the Log interface and the SPI/GPIO communication interface to the ADISense module.
Definition at line 215 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_RegisterGpioCallback | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice, |
ADI_SENSE_GPIO_PIN const | ePinId, | ||
ADI_SENSE_GPIO_CALLBACK const | callbackFunction, | ||
void *const | pCallbackParam | ||
) |
Register an application-defined callback function for GPIO interrupts.
- Parameters:
-
[in] hDevice ADISENSE context handle (adi_sense_Open) [in] ePinId GPIO pin on which to enable/disable interrupts [in] callbackFunction Function to be called when an interrupt occurs. Specify NULL here to disable interrupts. [in] pCallbackParam Optional opaque parameter passed to the callback
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
- ADI_SENSE_INVALID_DEVICE_NUM Invalid GPIO pin specified.
Definition at line 261 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_Reset | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice ) |
Reset the ADISENSE device.
- Parameters:
-
[in] hDevice ADISENSE device context handle
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Trigger a hardware-reset of the ADISENSE device.
- Note:
- The device may require several seconds before it is ready for use again. adi_sense_GetDeviceReadyState may be used to check if the device is ready.
Definition at line 280 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_RestoreConfig | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice, |
ADI_SENSE_USER_CONFIG_SLOT const | eSlotId | ||
) |
Restore configuration settings from persistent memory on the device.
- Parameters:
-
[in] hDevice ADISENSE device context handle [in] eSlotId User configuration slot in persistent memory
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Instructs the ADISENSE device to restore the contents of its device configuration registers from non-volatile memory.
- Note:
- No other command must be running when this is called.
Definition at line 554 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_RestoreLutData | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice ) |
Restore LUT data from persistent memory on the device.
- Parameters:
-
[in] hDevice ADISENSE device context handle
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Instructs the ADISENSE device to restore the contents of its LUT data, previously stored with adi_sense_SaveLutData, from non-volatile memory.
- Note:
- No other command must be running when this is called.
Definition at line 715 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_RunCalibration | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice ) |
Run built-in calibration on the device.
- Parameters:
-
[in] hDevice ADISENSE device context handle
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Instructs the ADISENSE device to execute its self-calibration routines, on any enabled measurement channels, according to the current applied configuration settings. Device status registers will be updated to indicate if any errors were detected.
- Note:
- No other command must be running when this is called.
Definition at line 747 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_RunDiagnostics | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice ) |
Run built-in diagnostic checks on the device.
- Parameters:
-
[in] hDevice ADISENSE device context handle
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Instructs the ADISENSE device to execute its built-in diagnostic tests, on any enabled measurement channels, according to the current applied configuration settings. Device status registers will be updated to indicate if any errors were detected by the diagnostics.
- Note:
- No other command must be running when this is called.
Definition at line 736 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_RunDigitalCalibration | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice ) |
Run built-in digital calibration on the device.
- Parameters:
-
[in] hDevice ADISENSE device context handle
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Instructs the ADISENSE device to execute its calibration routines, on any enabled digital channels, according to the current applied configuration settings. Device status registers will be updated to indicate if any errors were detected.
- Note:
- No other command must be running when this is called.
Definition at line 758 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_SaveConfig | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice, |
ADI_SENSE_USER_CONFIG_SLOT const | eSlotId | ||
) |
Store the configuration settings to persistent memory on the device.
- Parameters:
-
[in] hDevice ADISENSE device context handle [in] eSlotId User configuration slot in persistent memory
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Instructs the ADISENSE device to save the current contents of its device configuration registers to non-volatile memory.
- Note:
- No other command must be running when this is called.
- Do not power down the device while this command is running.
Definition at line 530 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_SaveLutData | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice ) |
Store the LUT data to persistent memory on the device.
- Parameters:
-
[in] hDevice ADISENSE device context handle
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Instructs the ADISENSE device to save the current contents of its LUT data buffer, set using adi_sense_SetLutData, to non-volatile memory.
- Note:
- No other command must be running when this is called.
- Do not power down the device while this command is running.
Definition at line 705 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_SetConfig | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice, |
ADI_SENSE_CONFIG *const | pConfig | ||
) |
Write full configuration settings to the device registers.
- Parameters:
-
[in] hDevice ADISENSE device context handle [out] pConfig Pointer to the configuration data structure
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Translates configuration details provided into device-specific register settings and updates device configuration registers.
- Note:
- Settings are not applied until adi_sense_ApplyConfigUpdates() is called
Definition at line 2824 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_Shutdown | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice ) |
Trigger a shut down of the device.
- Parameters:
-
[in] hDevice ADISENSE device context handle
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Instructs the ADISENSE device to initiate a shut down, typically used to conserve power when the device is not in use. The device may be restarted by calling adi_sense_Reset(). Note that active configuration settings are not preserved during shutdown and must be reloaded after the device has become ready again.
- Note:
- No other command must be running when this is called.
Definition at line 476 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_StartMeasurement | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice, |
ADI_SENSE_MEASUREMENT_MODE const | eMeasurementMode | ||
) |
Start the measurement cycles on the device.
- Parameters:
-
[in] hDevice ADISENSE device context handle [in] eMeasurementMode Allows a choice of special modes for the measurement. See ADI_SENSE_MEASUREMENT_MODE for further information.
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Instructs the ADISENSE device to start executing measurement cycles according to the current applied configuration settings. The DATAREADY status signal will be asserted whenever new measurement data is published, according to selected settings. Measurement cycles may be stopped by calling adi_sense_StopMeasurement.
- Note:
- No other command must be running when this is called.
Start the measurement cycles on the device.
- Parameters:
-
[out]
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
- ADI_SENSE_FAILURE
Sends the latch config command. Configuration for channels in conversion cycle should be completed before this function. Channel enabled bit should be set before this function. Starts a conversion and configures the format of the sample.
Definition at line 504 of file adi_sense_1000.c.
ADI_SENSE_RESULT adi_sense_StopMeasurement | ( | ADI_SENSE_DEVICE_HANDLE const | hDevice ) |
Stop the measurement cycles on the device.
- Parameters:
-
[in] hDevice ADISENSE device context handle
- Returns:
- Status
- ADI_SENSE_SUCCESS Call completed successfully.
Instructs the ADISENSE device to stop executing measurement cycles. The command may be delayed until the current conversion, if any, has been completed and published.
- Note:
- To be used only if a measurement command is currently running.
Definition at line 725 of file adi_sense_1000.c.
Generated on Tue Jul 12 2022 18:59:24 by
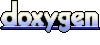