
Using the BLE Loopback UART based program to work with LSM9DS1 sensor
Dependencies: BLE_API LSM9DS1 mbed nRF51822
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "ble/BLE.h" 00019 00020 #include "ble/services/UARTService.h" 00021 #include "LSM9DS1/LSM9DS1.h" 00022 00023 LSM9DS1 imu(p30, p7); 00024 Serial pc(p9, p11); 00025 00026 #define NEED_CONSOLE_OUTPUT 1 /* Set this if you need debug messages on the console; 00027 * it will have an impact on code-size and power consumption. */ 00028 00029 #if NEED_CONSOLE_OUTPUT 00030 #define DEBUG(...) { pc.printf(__VA_ARGS__); } 00031 #else 00032 #define DEBUG(...) /* nothing */ 00033 #endif /* #if NEED_CONSOLE_OUTPUT */ 00034 00035 BLEDevice ble; 00036 DigitalOut led1(LED1); 00037 00038 UARTService *uartServicePtr; 00039 00040 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00041 { 00042 DEBUG("Disconnected!\n\r"); 00043 DEBUG("Restarting the advertising process\n\r"); 00044 ble.startAdvertising(); 00045 } 00046 00047 void onDataWritten(const GattWriteCallbackParams *params) 00048 { 00049 if ((uartServicePtr != NULL) && (params->handle == uartServicePtr->getTXCharacteristicHandle())) { 00050 uint16_t bytesRead = params->len; 00051 uint8_t value = *(params->data); 00052 uint16_t status; 00053 DEBUG("received %u bytes\n\r", bytesRead); 00054 DEBUG("recevied %d data\n\r", value); 00055 00056 switch(value) { 00057 case 49: 00058 DEBUG("Off power\n\r"); 00059 status = imu.begin(imu.G_SCALE_245DPS, imu.A_SCALE_2G, imu.M_SCALE_4GS, 00060 imu.G_POWER_DOWN, imu.A_POWER_DOWN, imu.M_ODR_0625); 00061 break; 00062 case 50: 00063 DEBUG("Low power\n\r"); 00064 status = imu.begin(imu.G_SCALE_245DPS, imu.A_SCALE_2G, imu.M_SCALE_4GS, 00065 imu.G_ODR_15_BW_0, imu.A_POWER_DOWN, imu.M_ODR_0625); 00066 break; 00067 case 51: 00068 DEBUG("High power\n\r"); 00069 status = imu.begin(imu.G_SCALE_2000DPS, imu.A_SCALE_8G, imu.M_SCALE_16GS, 00070 imu.G_ODR_952_BW_100, imu.A_ODR_952, imu.M_ODR_80); 00071 break; 00072 default: 00073 DEBUG("Nothing happened\n\r"); 00074 break; 00075 } 00076 00077 pc.printf("LSM9DS1 WHO_AM_I's returned: 0x%X\r\n", status); 00078 pc.printf("Should be 0x683D\r\n"); 00079 00080 ble.updateCharacteristicValue(uartServicePtr->getRXCharacteristicHandle(), params->data, bytesRead); 00081 } 00082 } 00083 00084 void periodicCallback(void) 00085 { 00086 led1 = !led1; 00087 } 00088 00089 int main(void) 00090 { 00091 led1 = 1; 00092 Ticker ticker; 00093 ticker.attach(periodicCallback, 1); 00094 00095 DEBUG("Initialising the nRF51822\n\r"); 00096 pc.printf("PC: Initialising the nRF51822\n\r"); 00097 ble.init(); 00098 ble.onDisconnection(disconnectionCallback); 00099 ble.onDataWritten(onDataWritten); 00100 00101 /* setup advertising */ 00102 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00103 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00104 ble.accumulateAdvertisingPayload(GapAdvertisingData::SHORTENED_LOCAL_NAME, 00105 (const uint8_t *)"BLE UART", sizeof("BLE UART") - 1); 00106 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_128BIT_SERVICE_IDS, 00107 (const uint8_t *)UARTServiceUUID_reversed, sizeof(UARTServiceUUID_reversed)); 00108 00109 ble.setAdvertisingInterval(1000); /* 1000ms; in multiples of 0.625ms. */ 00110 ble.startAdvertising(); 00111 00112 UARTService uartService(ble); 00113 uartServicePtr = &uartService; 00114 00115 while (true) { 00116 ble.waitForEvent(); 00117 } 00118 }
Generated on Thu Jul 14 2022 10:45:34 by
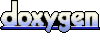