
.
Dependencies: eeprom keypad mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "Keypad.h" 00004 #include "eeprom.h" 00005 #include "stdlib.h" 00006 00007 //EEPROM memory(I2C_SDA,I2C_SCL,0); 00008 Serial PC(D1, D0); 00009 // Define your own keypad values 00010 char Keytable[] = { '1', '2', '3', 'A', // r0 00011 '4', '5', '6', 'B', // r1 00012 '7', '8', '9', 'C', // r2 00013 '*', '0', '#', 'D' // r3 00014 }; 00015 // c0 c1 c2 c3 00016 00017 uint32_t Index; 00018 uint32_t oldIndex; 00019 string arraysong[4][5] ={{"1A", "2A", "3A","4A", "5A"}, 00020 {"1B", "2B", "3B","4B", "5B"}, 00021 {"1C", "2C", "3C","4C", "5C"}, 00022 {"1D", "2D", "3D","4D", "5D"},}; //keep name's songs to random 00023 00024 char like; // if user likes, it send "l" /dislikes send "d" / wants to pass send "o" 00025 00026 uint32_t cbAfterInput(uint32_t index) { 00027 Index = index; 00028 return 0; 00029 } 00030 int randomja(); 00031 int numra; // a number gets from randomja() 00032 00033 int main() { 00034 // r0 r1 r2 r3 c0 c1 c2 c3 00035 Keypad keypad(A5, A4, D2,D3, D4, D5, D6, D7); 00036 keypad.attach(&cbAfterInput); 00037 keypad.start(); // energize the keypad via c0-c3 00038 00039 while (1) { 00040 __wfi(); 00041 //printf("Interrupted\r\n"); 00042 //PC.printf("Index:%d\n",Index); 00043 //PC.printf("oldIndex:%d\n",oldIndex); 00044 //wait_ms(1000); 00045 00046 while (oldIndex != Index){ 00047 PC.printf("Index:%d => Key:%c\r\n", Index, Keytable[Index]); 00048 if (Index == 4){ 00049 numra = rand()%5; 00050 printf("Jibinarak4 %d\n",numra); 00051 printf("song is %s\n",arraysong[Index-4][numra]); 00052 /* memory.write(1,Index,1); 00053 wait_ms(1); 00054 memory.write(2,arraysong,100); 00055 wait_ms(1); 00056 */ 00057 Index =0; numra = 0; 00058 oldIndex = Index; 00059 } 00060 else if (Index == 5){ 00061 numra = rand()%5; 00062 printf("Jibinarak5 %d\n",numra); 00063 printf("song is %s\n",arraysong[Index-4][numra]); 00064 /* memory.write(3,Index,1); 00065 wait_ms(1); 00066 memory.write(4,arraysong,100); 00067 wait_ms(1); 00068 */ 00069 Index =0; numra = 0; 00070 oldIndex = Index; 00071 } 00072 else if (Index == 6){ 00073 numra = rand()%5; 00074 printf("Jibinarak6 %d\n",numra); 00075 PC.printf("song is %s\n",arraysong[Index-4][numra]); 00076 /* memory.write(5,Index,1); 00077 wait_ms(1); 00078 memory.write(6,arraysong,100); 00079 wait_ms(1); 00080 */ 00081 Index =0; numra = 0; 00082 oldIndex = Index; 00083 } 00084 else if (Index == 7){ 00085 numra = rand()%5; 00086 printf("Jibinarak7 %d\n",numra); 00087 PC.printf("song is %s\n",arraysong[Index-4][numra]); 00088 /* memory.write(7,Index,1); 00089 wait_ms(1); 00090 memory.write(8,arraysong,100); 00091 wait_ms(1); 00092 */ 00093 Index =0; numra = 0; 00094 oldIndex = Index; 00095 } 00096 else if (Index == 8){ 00097 like = 'l'; 00098 printf("like : %c\n",like); 00099 00100 /* memory.write(9,Index,1); 00101 wait_ms(1); 00102 memory.write(10,like,1); 00103 wait_ms(1); 00104 */ oldIndex = Index; 00105 } 00106 else if (Index == 9){ 00107 like = 'd'; 00108 printf("like : %c\n",like); 00109 00110 /* memory.write(11,Index,1); 00111 wait_ms(1); 00112 memory.write(12,like,1); 00113 wait_ms(1); 00114 */ oldIndex = Index; 00115 } 00116 else if (Index == 10){ 00117 like = 'o'; 00118 printf("like : %c\n",like); 00119 00120 /* memory.write(13,Index,1); 00121 wait_ms(1); 00122 memory.write(14,like,1); 00123 wait_ms(1); 00124 */ oldIndex = Index; 00125 } 00126 else{oldIndex = Index;} 00127 } 00128 } 00129 00130 }
Generated on Thu Jul 28 2022 16:57:33 by
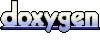