
Code for RFID Robot
Dependencies: DebounceIn HTTPClient ID12RFID SDFileSystem TextLCD WiflyInterface iniparser mbed
wifiunit.cpp
00001 #include "mbed.h" 00002 #include "WiflyInterface.h" 00003 #include "HTTPClient.h" 00004 #include <vector> 00005 #include "SD.h" 00006 00007 WiflyInterface wifly(p13, p14, p29, p30, "GTother", "GeorgeP@1927", WPA); 00008 00009 HTTPClient http; 00010 char str[512]; 00011 00012 void setupWiFi() 00013 { 00014 printf("trying to connect"); 00015 wifly.init(); 00016 while (!wifly.connect()) wait (0.1); 00017 printf("IP Address is %s\n\r", wifly.getIPAddress()); 00018 } 00019 00020 vector<robotCommand>* getTagCommands(int tagID) 00021 { 00022 vector<robotCommand>* commandVector = new vector<robotCommand>(); 00023 printLCD("Translating", "Web Server"); 00024 char url[512]; 00025 char str[512]; 00026 sprintf(url, "http://sabacai.com/index.php?tag=%d", tagID); 00027 printf("url is %s", url); 00028 int ret = http.get(url, str, 512); 00029 if (!ret) { 00030 printf("Tag String Received - read %d characters\n", strlen(str)); 00031 str[strlen(str)-1] = '\0'; // remove the last column 00032 printf("Result: %s\n", str); 00033 } 00034 00035 char * pch; 00036 printf ("Splitting string \"%s\" into tokens:\n",str); 00037 pch = strtok (str,","); 00038 00039 int i = 0; 00040 CommandType commandID = None; 00041 00042 while (pch != NULL) 00043 { 00044 printf ("%s\n",pch); 00045 double magnitude = 0.0; 00046 00047 if ((i&0x1) == 0) 00048 { 00049 // this is the command type 00050 if (strcmp(pch, "FORWARD") == 0) 00051 commandID = Forward; 00052 else if (strcmp(pch, "REVERSE") == 0) 00053 commandID = Reverse; 00054 else if (strcmp(pch, "LEFT") == 0) 00055 commandID = Left; 00056 else if (strcmp(pch, "RIGHT") == 0) 00057 commandID = Right; 00058 else if (strcmp(pch, "PLAYSOUND") == 0) 00059 commandID = PlaySound; 00060 00061 printf("command name is %s, id is %d", pch, commandID); 00062 } 00063 else 00064 { 00065 // this is the magnitude 00066 magnitude = atof(pch); 00067 printf("magnitude is %f", magnitude); 00068 } 00069 00070 // do some stuff here 00071 if (i != 0 && ((i&0x1) == 1)) 00072 { 00073 // add the command to the vector 00074 printf(" now adding %d, %f", commandID, magnitude); 00075 commandVector->push_back(robotCommand(commandID, magnitude)); 00076 } 00077 i+=1; 00078 pch = strtok (NULL, ","); 00079 } 00080 00081 return commandVector; 00082 }
Generated on Tue Jul 12 2022 18:24:35 by
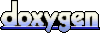