
Code for RFID Robot
Dependencies: DebounceIn HTTPClient ID12RFID SDFileSystem TextLCD WiflyInterface iniparser mbed
RFID.cpp
00001 #include "RFID.h" 00002 00003 //Takes in vector by reference and populates it with unique RFID values 00004 //until pb21 is pressed. 00005 int readTagSequence(std::vector<int>& v) 00006 { 00007 int currTag; 00008 int size = 0; 00009 printLCD("Scan tag", NULL); 00010 while (select) { 00011 if(rfid.readable()) { 00012 currTag = rfid.read(); 00013 size++; 00014 v.resize(size); 00015 v[size-1] = currTag; 00016 char line1[16]; 00017 sprintf(line1, "%d", v[size-1]); 00018 printLCD(line1, "added to list"); 00019 wait(1.5); 00020 printLCD("Scan tag", NULL); 00021 } 00022 else if(!back) return 0; 00023 } 00024 return 1; 00025 } 00026 00027 //Reads a single RFID tag, and returns value 00028 int readTag() 00029 { 00030 printLCD("Scan tag", NULL); 00031 int currTag = 0; 00032 while (!currTag) { 00033 if (rfid.readable()) { 00034 currTag = rfid.read(); 00035 char line1[16]; 00036 sprintf(line1, "%d", currTag); 00037 printLCD(line1, "read"); 00038 wait(1.5); 00039 return currTag; 00040 } 00041 else if (!back) { 00042 return 0; 00043 } 00044 } 00045 return currTag; 00046 }
Generated on Tue Jul 12 2022 18:24:35 by
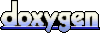