
Clock display on app board LCD is set using an NTP network time server. DHCP service must be enabled for mbed to get an IP address. A network cable is needed.
Dependencies: C12832_lcd EthernetInterface NTPClient mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "NTPClient.h" 00004 #include "C12832_lcd.h" 00005 00006 C12832_LCD lcd; //Graphics LCD 00007 EthernetInterface eth; 00008 NTPClient ntp; 00009 00010 int main() 00011 { 00012 eth.init(); //Use DHCP 00013 wait(2); 00014 lcd.cls(); 00015 lcd.printf("Getting IP Address\r\n"); 00016 if(eth.connect(60000)!=0) { 00017 lcd.printf("DHCP error - No IP"); 00018 wait(10); 00019 } else { 00020 lcd.printf("IP is %s\n", eth.getIPAddress()); 00021 wait(2); 00022 } 00023 lcd.cls(); 00024 lcd.printf("Trying to update time...\r\n"); 00025 if (ntp.setTime("0.pool.ntp.org") == 0) { 00026 lcd.printf("Set time successfully\r\n"); 00027 while(1) { 00028 lcd.cls(); 00029 lcd.locate(0,0); 00030 time_t ctTime; 00031 ctTime = time(NULL); 00032 lcd.printf("%s\r\n", ctime(&ctTime)); 00033 lcd.printf("Current Time (UTC)"); 00034 wait(1); 00035 } 00036 } else { 00037 lcd.printf("NTP Error\r\n"); 00038 } 00039 00040 eth.disconnect(); 00041 00042 while(1) { 00043 } 00044 }
Generated on Sun Jul 17 2022 06:21:48 by
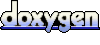