
RF link test program using low cost modules from Sparkfun See http://mbed.org/users/4180_1/notebook/ir-and-rf-remote-controls/
main.cpp
00001 #include "mbed.h" 00002 00003 Serial pc(USBTX, USBRX); // tx, rx 00004 Serial device(p9, p10); // tx, rx 00005 DigitalOut myled1(LED1); 00006 DigitalOut myled2(LED2); 00007 //RF link demo using low-cost Sparkfun RF transmitter and receiver modules 00008 //LEDs indicate link activity 00009 //Characters typed in PC terminal windows will echo back using RF link 00010 int main() { 00011 char temp=0; 00012 device.baud(2400); 00013 while (1) { 00014 00015 //RF Transmit Code 00016 if (pc.readable()==0) { 00017 myled1 = 1; 00018 //Send 10101010 pattern when idle to keep receiver in sync and locked to transmitter 00019 //When receiver loses the sync lock (Around 30MS with no data change seen) it starts sending out noise 00020 device.putc(0xAA); 00021 myled1 = 0; 00022 } else 00023 //Send out the real data whenever a key is typed 00024 device.putc(pc.getc()); 00025 00026 //RF Receive Code 00027 if (device.readable()) { 00028 myled2 = 1; 00029 temp=device.getc(); 00030 //Ignore Sync pattern and do not pass on to PC 00031 if (temp!=0xAA) pc.putc(temp); 00032 myled2 = 0; 00033 } 00034 } 00035 }
Generated on Sun Jul 17 2022 06:10:12 by
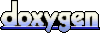