
Pushbutton demo using interrupts with switch contact bounce
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut myled(LED1); 00004 DigitalOut myled2(LED2); 00005 DigitalOut myled3(LED3); 00006 DigitalOut myled4(LED4); 00007 00008 InterruptIn pb(p8); 00009 // SPST Pushbutton count demo using interrupts 00010 // no external PullUp resistor needed 00011 // Pushbutton from P8 to GND. 00012 // A pb hit generates an interrupt and activates the interrupt routine 00013 00014 // Global count variable 00015 int volatile count=0; 00016 00017 // pb Interrupt routine - is interrupt activated by a falling edge of pb input 00018 void pb_hit_interrupt (void) { 00019 count++; 00020 myled4 = count & 0x01; 00021 myled3 = (count & 0x02)>>1; 00022 myled2 = (count & 0x04)>>2; 00023 } 00024 00025 int main() { 00026 // Use internal pullup for pushbutton 00027 pb.mode(PullUp); 00028 // Delay for initial pullup to take effect 00029 wait(.01); 00030 // Attach the address of the interrupt handler routine for pushbutton 00031 pb.fall(&pb_hit_interrupt); 00032 // Blink myled in main routine forever while responding to pb changes 00033 // via interrupts that activate pb_hit_interrupt routine 00034 while (1) { 00035 myled = !myled; 00036 wait(.5); 00037 } 00038 }
Generated on Thu Jul 14 2022 19:12:40 by
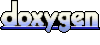