
Runs an RC Brushless DC motor using with an ESC module. Mbed supplies the PWM control signal for the ESC. See https://developer.mbed.org/users/4180_1/notebook/using-a-dc-brushless-motor-with-an-rc-esc/ for more info
main.cpp
00001 // Calibrate and arm ESC and then sweep through motor speed range 00002 // To run: Hold down reset on mbed and power up ESC motor supply 00003 // Wait for three power up beeps from ESC, then release reset (or apply power) on mbed 00004 // See https://github.com/bitdump/BLHeli/blob/master/BLHeli_S%20SiLabs/BLHeli_S%20manual%20SiLabs%20Rev16.x.pdf 00005 // for info on beep codes and calibration 00006 #include "mbed.h" 00007 #include "Servo.h" 00008 PwmOut ledf(LED1); //throttle up test led with PWM dimming 00009 PwmOut ledr(LED2); //throttle down test led with PWM dimming 00010 00011 Servo myservo(p21); 00012 00013 int main() 00014 { 00015 myservo = 0.0; 00016 ledf = ledr = 1; 00017 wait(0.5); //ESC detects signal 00018 //Required ESC Calibration/Arming sequence 00019 //sends longest and shortest PWM pulse to learn and arm at power on 00020 myservo = 1.0; //send longest PWM 00021 ledf = ledr = 0; 00022 wait(8); 00023 myservo = 0.0; //send shortest PWM 00024 wait(8); 00025 //ESC now operational using standard servo PWM signals 00026 while (1) { 00027 for (float p=0.0; p<=1.0; p += 0.025) { //Throttle up slowly to full throttle 00028 myservo = p; 00029 ledf = p; 00030 wait(1.0); 00031 } 00032 myservo = 0.0; //Motor off 00033 ledf = ledr = 0; 00034 wait(4.0); 00035 for (float p=1.0; p>=0.0; p -= 0.025) { //Throttle down slowly from full throttle 00036 myservo = p; 00037 ledr = p; 00038 wait(1.0); 00039 } 00040 myservo = 0.0; //Motor off 00041 ledf = ledr = 0; 00042 wait(4.0); 00043 } 00044 }
Generated on Thu Jul 14 2022 13:40:21 by
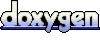