
Test CAN bus on Stm32F746nG
Dependencies: F746_GUI mbed CANMsg
main.cpp
00001 /* 00002 programme test sur carte stm32F746ng-discovery 00003 00004 update 30/07/2019 : display datas on lcd ok 00005 update 29/07/2020 : send can ok ( after received), receive can ok 00006 update 29/07/2020 : remove touch screen 00007 update 28/07/2020 : add serial and CAN 00008 update 21/07/2020 : test buttons 00009 update 20/07/2020 : display buttons 00010 update 17/07/2020 GC : change colors 00011 */ 00012 00013 #include "mbed.h" 00014 #include "stm32746g_discovery_lcd.h" 00015 #include "stm32746g_discovery_ts.h" 00016 #include <string> 00017 #include "CANMsg.h" 00018 #include <stdio.h> 00019 #include <errno.h> 00020 00021 // serial port sur connecteur arduino (D0,D1)-------------------- 00022 Serial serial2(PC_6, PC_7); // TX, RX for udp bridge 00023 Serial pc(USBTX, USBRX); // serial on usb connector 00024 // CAN ------------------------------------------------------------ 00025 CAN can(PB_8, PB_9); // CAN Rx pin name, CAN Tx pin name 00026 CANMsg rxMsg; 00027 CANMsg txMsg; 00028 00029 uint8_t counter = 0; 00030 float voltage; 00031 Timer timer; 00032 volatile bool msgAvailable = false; 00033 AnalogIn analogIn(A0); 00034 CircularBuffer<char, 1024> rxbuf; // PC receiving Buffer 00035 uint8_t text2[30]; 00036 char s2; 00037 char buffer[128]; 00038 #define LED_PIN PC_13 00039 DigitalOut led(LED_PIN); 00040 00041 //#define TARGET_STM32F103C8T6 1 // uncomment this line to use STM32F103C8T6 boards 00042 #define BOARD1 1 // comment out this line when compiling for board #2 00043 #if defined(BOARD1) 00044 const unsigned int RX_ID = 0x100; 00045 const unsigned int TX_ID = 0x101; 00046 #else 00047 const unsigned int RX_ID = 0x101; 00048 const unsigned int TX_ID = 0x100; 00049 #endif 00050 00051 /** 00052 * @brief Prints CAN message to PC's serial terminal 00053 * @param CANMessage to print 00054 */ 00055 void printMsg(CANMessage& msg, int dir) 00056 { if (dir ==0) 00057 { BSP_LCD_DisplayStringAt(0, LINE(6), (uint8_t *)"Message sent ", LEFT_MODE); 00058 } 00059 else 00060 { BSP_LCD_DisplayStringAt(0, LINE(6), (uint8_t *)"Message received", LEFT_MODE); 00061 } 00062 pc.printf(" ID = 0x%.3x\r\n", msg.id); 00063 pc.printf(" Type = %d\r\n", msg.type); 00064 pc.printf(" Format = %d\r\n", msg.format); 00065 pc.printf(" Length = %d\r\n", msg.len); 00066 pc.printf(" Data ="); 00067 for(int i = 0; i < msg.len; i++) 00068 pc.printf(" 0x%.2X", msg.data[i]); 00069 pc.printf("\r\n"); 00070 00071 sprintf((char*)text2, "ID: %d", msg.id); 00072 BSP_LCD_DisplayStringAt(2, LINE(7), (uint8_t *)&text2, LEFT_MODE); 00073 sprintf((char*)text2, "Type: %d", msg.type); 00074 BSP_LCD_DisplayStringAt(2, LINE(8), (uint8_t *)&text2, LEFT_MODE); 00075 sprintf((char*)text2, "Format: %d", msg.format); 00076 BSP_LCD_DisplayStringAt(2, LINE(9), (uint8_t *)&text2, LEFT_MODE); 00077 sprintf((char*)text2, "Length: %d", msg.len); 00078 BSP_LCD_DisplayStringAt(2, LINE(10), (uint8_t *)&text2, LEFT_MODE); 00079 sprintf((char*)text2, "Data: %d", msg.data[0]); 00080 BSP_LCD_DisplayStringAt(2, LINE(11), (uint8_t *)&text2, LEFT_MODE); 00081 } 00082 00083 /** 00084 * @brief Handles received CAN messages 00085 * @note Called on 'CAN message received' interrupt. 00086 */ 00087 void onCanReceived(void) 00088 { can.read(rxMsg); 00089 pc.printf("-------------------------------------\r\n"); 00090 pc.printf("CAN message received\r\n"); 00091 serial2.printf("CAN message received\r\n"); 00092 BSP_LCD_DisplayStringAt(0, LINE(5), (uint8_t *)"Can msg rec", CENTER_MODE); 00093 //BSP_LCD_DisplayStringAt(0, LINE(3), (uint8_t *)"-----------", CENTER_MODE); 00094 printMsg(rxMsg,1); 00095 //serial2.printf("%d\n",rxMsg); 00096 //serial2.printf("ID: "); 00097 serial2.printf("ID: 0x%.3x ", rxMsg.id); 00098 serial2.printf("D: "); 00099 for(int i = 0; i < rxMsg.len; i++) 00100 serial2.printf(" 0x%.2X", rxMsg.data[i]); 00101 serial2.printf("\r\n"); 00102 if (rxMsg.id == RX_ID) { 00103 // extract data from the received CAN message 00104 // in the same order as it was added on the transmitter side 00105 rxMsg >> counter; 00106 rxMsg >> voltage; 00107 pc.printf(" counter = %d\r\n", counter); 00108 pc.printf(" voltage = %e V\r\n", voltage); 00109 } 00110 timer.start(); // to transmit next message in main 00111 } 00112 00113 // ------------------------------------------------------------------------------------------------------ 00114 int main() 00115 { TS_StateTypeDef TS_State; 00116 uint16_t x, y; 00117 uint8_t text[30]; 00118 uint8_t status; 00119 uint8_t idx; 00120 uint8_t cleared = 0; 00121 uint8_t menu_level = 0; 00122 uint8_t prev_nb_touches = 0; 00123 uint8_t touche_appuyee = 99; 00124 string touche_appuyee_s = "%"; 00125 string text_display1 ("Memorisation Touches "); 00126 char buffer_touche[50]; 00127 // set serial speed 00128 pc.baud(57600); 00129 serial2.baud(57600); 00130 // config can -------------------------------------- 00131 //jpa can = new CAN(PB_8, PB_9); // CAN Rx pin name, CAN Tx pin name 00132 can.frequency(125000); // set CAN bit rate to 125 kbps 00133 //can.filter(RX_ID, 0xFFF, CANStandard, 0); // set filter #0 to accept only standard messages with ID == RX_ID 00134 can.attach(onCanReceived); // attach ISR to handle received messages 00135 //can->attach(&onMsgReceived); //msgAvailable = true; // attach the 'CAN receive-complete' interrupt service routine (ISR) 00136 #if defined(BOARD1) 00137 timer.start(); // start timer 00138 pc.printf("CAN_Hello board #1\r\n"); 00139 #else 00140 pc.printf("CAN_Hello board #2\r\n"); 00141 #endif 00142 pc.printf("\nStart Prog\n"); 00143 serial2.printf("\nStart Prog\n"); 00144 BSP_LCD_Init(); 00145 BSP_LCD_LayerDefaultInit(LTDC_ACTIVE_LAYER, LCD_FB_START_ADDRESS); 00146 BSP_LCD_SelectLayer(LTDC_ACTIVE_LAYER); 00147 //BSP_LCD_DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN DEMO", CENTER_MODE); 00148 //HAL_Delay(1000); 00149 status = BSP_TS_Init(BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00150 if (status != TS_OK) { 00151 BSP_LCD_Clear(LCD_COLOR_RED); 00152 BSP_LCD_SetBackColor(LCD_COLOR_RED); 00153 BSP_LCD_SetTextColor(LCD_COLOR_WHITE); 00154 BSP_LCD_DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN INIT FAIL", CENTER_MODE); 00155 } else { 00156 BSP_LCD_Clear(LCD_COLOR_BLUE); 00157 BSP_LCD_SetBackColor(LCD_COLOR_BLUE); 00158 BSP_LCD_SetTextColor(LCD_COLOR_WHITE); 00159 BSP_LCD_DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN INIT OK", CENTER_MODE); 00160 } 00161 pc.printf("\nInit Display\n"); 00162 HAL_Delay(1000); 00163 BSP_LCD_Clear(LCD_COLOR_BLUE); 00164 BSP_LCD_SetBackColor(LCD_COLOR_BLUE); 00165 BSP_LCD_SetTextColor(LCD_COLOR_WHITE); 00166 BSP_LCD_DisplayStringAt(0, LINE(2), (uint8_t *)"STM32F746 Disco Appli", CENTER_MODE); 00167 BSP_LCD_SetTextColor(LCD_COLOR_RED); 00168 BSP_LCD_DrawRect(10, 100, 200, 50); 00169 BSP_LCD_DrawRect(10, 180, 200, 50); 00170 BSP_LCD_SetTextColor(LCD_COLOR_YELLOW); 00171 BSP_LCD_DisplayStringAt(25, LINE(5), (uint8_t *)"Setup", LEFT_MODE); 00172 BSP_LCD_DisplayStringAt(25, 200, (uint8_t *)"Run", LEFT_MODE); 00173 BSP_LCD_SetFont(&Font12); 00174 00175 BSP_LCD_Clear(LCD_COLOR_BLUE); 00176 BSP_LCD_SetBackColor(LCD_COLOR_BLUE); 00177 BSP_LCD_SetTextColor(LCD_COLOR_WHITE); 00178 BSP_LCD_SetFont(&Font16); 00179 BSP_LCD_DisplayStringAt(0, LINE(2), (uint8_t *)"Mode Run", CENTER_MODE); 00180 00181 00182 //HAL_Delay(1000); 00183 BSP_LCD_SetFont(&Font12); 00184 BSP_LCD_SetBackColor(LCD_COLOR_BLUE); 00185 BSP_LCD_SetTextColor(LCD_COLOR_WHITE); 00186 00187 while(1) //menu_level == 1) 00188 { // timer send can 00189 if(timer.read_ms() >= 1000) // check for timeout 00190 { timer.stop(); // stop the timer 00191 timer.reset(); // reset the timer 00192 counter++; // increment the counter 00193 voltage = (analogIn * 3.3f)/4096.0f;// read the small drifting voltage from analog input 00194 txMsg.clear(); // clear the Tx message storage 00195 //txMsg.id = TX_ID; // set ID 00196 txMsg.id = 0x18881001;//TX_ID; // set the message ID 00197 txMsg.format = CANExtended ; //extended 00198 // We are about to transmit two data items to the CAN bus. 00199 // counter: uint_8 (unsigned eight bits int) value (one byte). 00200 // voltage: floating point value (four bytes). 00201 // So the total length of payload data is five bytes. 00202 // We'll use the "<<" (append) operator to add data to the CAN message. 00203 // The usage is same as of the similar C++ io-stream operators. 00204 // NOTE: The data length of CAN message is automatically updated when using "<<" operators. 00205 txMsg << counter << voltage; // append data (total data length must be <= 8 bytes!) 00206 00207 if(can.write(txMsg)) // transmit message 00208 { //if(can->write(txMsg)) { // transmit the CAN message 00209 //led = OFF; // turn the LED off 00210 pc.printf("-------------------------------------\r\n"); 00211 pc.printf("CAN message sent\r\n"); 00212 printMsg(txMsg,0); 00213 pc.printf(" counter = %d\r\n", counter); 00214 pc.printf(" voltage = %e V\r\n", voltage); 00215 BSP_LCD_DisplayStringAt(0, LINE(3), (uint8_t *)"TX CAN OK", CENTER_MODE); 00216 BSP_LCD_DisplayStringAt(0, LINE(4), (uint8_t *)"--------", CENTER_MODE); 00217 } 00218 else 00219 { pc.printf("Transmission error\r\n"); 00220 BSP_LCD_DisplayStringAt(0, LINE(4), (uint8_t *)"TX CAN Fail", CENTER_MODE); 00221 BSP_LCD_DisplayStringAt(0, LINE(3), (uint8_t *)"-----------", CENTER_MODE); 00222 } 00223 //timer.start(); // insert transmission lag 00224 } // end if timer 00225 // read can 00226 if (can.read(rxMsg)); 00227 { 00228 pc.printf("-------------------------------------\r\n"); 00229 pc.printf("CAN message received\r\n"); 00230 serial2.printf("CAN message received\r\n"); 00231 00232 printMsg(rxMsg,1); 00233 serial2.printf("%d\n",rxMsg); 00234 serial2.printf("ID: "); 00235 serial2.printf("ID: 0x%.3x ", rxMsg.id); 00236 serial2.printf("D: "); 00237 for(int i = 0; i < rxMsg.len; i++) 00238 serial2.printf(" 0x%.2X", rxMsg.data[i]); 00239 serial2.printf("\r\n"); 00240 } 00241 00242 00243 00244 // read serial port 00245 // get serial 2 00246 //serial2.gets(buffer, 4); 00247 //pc.printf("I got '%s'\n", buffer); 00248 char c = pc.getc(); 00249 00250 if((c == 's') ) 00251 { // send trame CAN 00252 if(can.write(txMsg)) // transmit message 00253 { //if(can->write(txMsg)) { // transmit the CAN message 00254 //led = OFF; // turn the LED off 00255 pc.printf("-------------------------------------\r\n"); 00256 pc.printf("CAN message sent\r\n"); 00257 printMsg(txMsg,0); 00258 pc.printf(" counter = %d\r\n", counter); 00259 pc.printf(" voltage = %e V\r\n", voltage); 00260 BSP_LCD_DisplayStringAt(0, LINE(3), (uint8_t *)"TX CAN OK", CENTER_MODE); 00261 BSP_LCD_DisplayStringAt(0, LINE(4), (uint8_t *)"--------", CENTER_MODE); 00262 } 00263 else 00264 { pc.printf("Transmission error\r\n"); 00265 BSP_LCD_DisplayStringAt(0, LINE(4), (uint8_t *)"TX CAN Fail", CENTER_MODE); 00266 BSP_LCD_DisplayStringAt(0, LINE(3), (uint8_t *)"-----------", CENTER_MODE); 00267 } 00268 } 00269 00270 char ser2 = serial2.getc(); 00271 if( (ser2 = 's') ) 00272 { // send trame CAN 00273 if(can.write(txMsg)) // transmit message 00274 { //if(can->write(txMsg)) { // transmit the CAN message 00275 //led = OFF; // turn the LED off 00276 pc.printf("-------------------------------------\r\n"); 00277 pc.printf("CAN message sent\r\n"); 00278 printMsg(txMsg,0); 00279 pc.printf(" counter = %d\r\n", counter); 00280 pc.printf(" voltage = %e V\r\n", voltage); 00281 BSP_LCD_DisplayStringAt(0, LINE(3), (uint8_t *)"TX CAN OK", CENTER_MODE); 00282 BSP_LCD_DisplayStringAt(0, LINE(4), (uint8_t *)"--------", CENTER_MODE); 00283 } 00284 else 00285 { pc.printf("Transmission error\r\n"); 00286 BSP_LCD_DisplayStringAt(0, LINE(4), (uint8_t *)"TX CAN Fail", CENTER_MODE); 00287 BSP_LCD_DisplayStringAt(0, LINE(3), (uint8_t *)"-----------", CENTER_MODE); 00288 } 00289 } 00290 00291 // gestion touches appuyees 00292 BSP_TS_GetState(&TS_State); 00293 if (TS_State.touchDetected) { 00294 // Clear lines corresponding to old touches coordinates 00295 if (TS_State.touchDetected < prev_nb_touches) { 00296 for (idx = (TS_State.touchDetected + 1); idx <= 5; idx++) { 00297 BSP_LCD_ClearStringLine(idx); 00298 } 00299 } 00300 prev_nb_touches = TS_State.touchDetected; 00301 cleared = 0; 00302 sprintf((char*)text, "Touches: %d", TS_State.touchDetected); 00303 BSP_LCD_DisplayStringAt(2, LINE(1), (uint8_t *)&text, LEFT_MODE); 00304 00305 for (idx = 0; idx < TS_State.touchDetected; idx++) { 00306 x = TS_State.touchX[idx]; 00307 y = TS_State.touchY[idx]; 00308 sprintf((char*)text, "Touch %d: x=%d y=%d ", idx+1, x, y); 00309 BSP_LCD_DisplayStringAt(2, LINE(idx+1), (uint8_t *)&text, LEFT_MODE); 00310 } 00311 BSP_LCD_DrawPixel(TS_State.touchX[0], TS_State.touchY[0], LCD_COLOR_ORANGE); 00312 00313 // rajouter test touche appuyée 00314 if ((y>100)& (y<150)) 00315 { // touches 0 a 5 appuyées 00316 if ((x>10)&(x<60)) 00317 { // touche 0 appuyée) 00318 sprintf((char*)text, "Touche 0 appuyée"); 00319 BSP_LCD_DisplayStringAt(2, LINE(idx+1), (uint8_t *)&text, LEFT_MODE); 00320 touche_appuyee = 0;touche_appuyee_s = "0"; 00321 } 00322 if ((x>90)&(x<130)) 00323 { // touche 1 appuyée) 00324 sprintf((char*)text, "Touche 1 appuyée"); 00325 BSP_LCD_DisplayStringAt(2, LINE(idx+1), (uint8_t *)&text, LEFT_MODE); 00326 touche_appuyee = 1;touche_appuyee_s = "1"; 00327 } 00328 if ((x>180)&(x<230)) 00329 { // touche 2 appuyée) 00330 sprintf((char*)text, "Touche 2 appuyée"); 00331 BSP_LCD_DisplayStringAt(2, LINE(idx+1), (uint8_t *)&text, LEFT_MODE); 00332 touche_appuyee = 2;touche_appuyee_s = "2"; 00333 } 00334 if ((x>260)&(x<310)) 00335 { // touche 3 appuyée) 00336 sprintf((char*)text, "Touche 3 appuyée"); 00337 BSP_LCD_DisplayStringAt(2, LINE(idx+1), (uint8_t *)&text, LEFT_MODE); 00338 touche_appuyee = 3;touche_appuyee_s = "3"; 00339 } 00340 if ((x>340)&(x<390)) 00341 { // touche 4 appuyée) 00342 sprintf((char*)text, "Touche 4 appuyée"); 00343 BSP_LCD_DisplayStringAt(2, LINE(idx+1), (uint8_t *)&text, LEFT_MODE); 00344 touche_appuyee = 4;touche_appuyee_s = "4"; 00345 } 00346 if ((x>420)&(x<470)) 00347 { // touche 5 appuyée) 00348 sprintf((char*)text, "Touche 5 appuyée"); 00349 BSP_LCD_DisplayStringAt(2, LINE(idx+1), (uint8_t *)&text, LEFT_MODE); 00350 touche_appuyee = 5;touche_appuyee_s = "5"; 00351 } 00352 text_display1 = text_display1 + touche_appuyee_s; 00353 sprintf(buffer_touche, "text_display1 %d", touche_appuyee); 00354 BSP_LCD_DisplayStringAt(2, 3, (uint8_t *)&buffer_touche, LEFT_MODE); 00355 } 00356 00357 } else { 00358 if (!cleared) 00359 { //BSP_LCD_Clear(LCD_COLOR_BLUE); 00360 // sprintf((char*)text, "Touches: 0"); 00361 // BSP_LCD_DisplayStringAt(2, LINE(2), (uint8_t *)&text, LEFT_MODE); 00362 cleared = 1; 00363 } 00364 } 00365 } 00366 } 00367 00368 //int main() { 00369 // pc.printf("Hello World!\n\r"); 00370 // while(1) { 00371 // pc.putc(pc.getc() + 1); // echo input back to terminal 00372 // }// 00373 //} 00374 /* 00375 int main() { 00376 while(1) { 00377 if(pc.readable()) { 00378 device.putc(pc.getc()); 00379 } 00380 if(device.readable()) { 00381 pc.putc(device.getc()); 00382 } 00383 } 00384 } 00385 */ 00386 /* 00387 #include "mbed.h" 00388 00389 Serial pc(USBTX, USBRX); // tx, rx 00390 PwmOut led(LED1); 00391 00392 float brightness = 0.0; 00393 00394 int main() { 00395 pc.printf("Press 'u' to turn LED1 brightness up, 'd' to turn it down\n"); 00396 00397 while(1) { 00398 char c = pc.getc(); 00399 if((c == 'u') && (brightness < 0.5)) { 00400 brightness += 0.01; 00401 led = brightness; 00402 } 00403 if((c == 'd') && (brightness > 0.0)) { 00404 brightness -= 0.01; 00405 led = brightness; 00406 } 00407 00408 } 00409 } 00410 */ 00411 /* 00412 #include "mbed.h" 00413 00414 Serial pc(USBTX, USBRX); 00415 Serial uart(p28, p27); 00416 00417 DigitalOut pc_activity(LED1); 00418 DigitalOut uart_activity(LED2); 00419 00420 int main() { 00421 while(1) { 00422 if(pc.readable()) { 00423 uart.putc(pc.getc()); 00424 pc_activity = !pc_activity; 00425 } 00426 if(uart.readable()) { 00427 pc.putc(uart.getc()); 00428 uart_activity = !uart_activity; 00429 } 00430 } 00431 } 00432 */ 00433 /* 00434 #include "mbed.h" 00435 00436 DigitalOut myled(LED1); 00437 Serial pc(USBTX, USBRX); 00438 00439 int main() { 00440 char c; 00441 char buffer[128]; 00442 00443 pc.gets(buffer, 4); 00444 00445 pc.printf("I got '%s'\n", buffer); 00446 } 00447 */ 00448 /* 00449 sd card filesystem 00450 /* Example file of using SD/MMC Block device Library for MBED-OS 00451 * Copyright 2017 Roy Krikke 00452 * Licensed under the Apache License, Version 2.0 (the "License"); 00453 * you may not use this file except in compliance with the License. 00454 * You may obtain a copy of the License at 00455 * http://www.apache.org/licenses/LICENSE-2.0 00456 * Unless required by applicable law or agreed to in writing, software 00457 * distributed under the License is distributed on an "AS IS" BASIS, 00458 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00459 * See the License for the specific language governing permissions and 00460 * limitations under the License. 00461 00462 #include "mbed.h" 00463 #include "FATFileSystem.h" 00464 #include "SDBlockDeviceDISCOF746NG.h" 00465 #include <stdio.h> 00466 #include <errno.h> 00467 00468 DigitalOut led (LED1); 00469 00470 // Instantiate the Block Device for sd card on DISCO-F746NG 00471 SDBlockDeviceDISCOF746NG bd; 00472 FATFileSystem fs ("fs"); 00473 00474 void 00475 return_error (int ret_val) 00476 { if(ret_val) 00477 printf ("Failure. %d\r\n", ret_val); 00478 else 00479 printf ("done.\r\n"); 00480 } 00481 00482 void 00483 errno_error (void* ret_val) 00484 { if(ret_val == NULL) 00485 printf (" Failure. %d \r\n", errno); 00486 else 00487 printf (" done.\r\n"); 00488 } 00489 00490 int 00491 main () 00492 { 00493 Serial pc (SERIAL_TX, SERIAL_RX); 00494 pc.baud(115200); 00495 printf("Start\n"); 00496 00497 int error = 0; 00498 printf("Welcome to the filesystem example.\r\n" 00499 "Formatting a FAT, RAM-backed filesystem. "); 00500 error = FATFileSystem::format(&bd); 00501 return_error(error); 00502 00503 printf("Mounting the filesystem on \"/fs\". "); 00504 error = fs.mount(&bd); 00505 return_error(error); 00506 00507 printf("Opening a new file, numbers.txt."); 00508 FILE* fd = fopen("/fs/numbers.txt", "w"); 00509 errno_error(fd); 00510 00511 for (int i = 0; i < 20; i++) { 00512 printf("Writing decimal numbers to a file (%d/20)\r", i); 00513 fprintf(fd, "%d\r\n", i); 00514 } 00515 printf("Writing decimal numbers to a file (20/20) done.\r\n"); 00516 00517 printf("Closing file."); 00518 fclose(fd); 00519 printf(" done.\r\n"); 00520 00521 printf("Re-opening file read-only."); 00522 fd = fopen("/fs/numbers.txt", "r"); 00523 errno_error(fd); 00524 00525 printf("Dumping file to screen.\r\n"); 00526 char buff[16] = { 0 }; 00527 while(!feof (fd)) { 00528 int size = fread(&buff[0], 1, 15, fd); 00529 fwrite(&buff[0], 1, size, stdout); 00530 } 00531 printf("EOF.\r\n"); 00532 00533 printf("Closing file."); 00534 fclose(fd); 00535 printf(" done.\r\n"); 00536 00537 printf("Opening root directory."); 00538 DIR* dir = opendir("/fs/"); 00539 errno_error(fd); 00540 00541 struct dirent* de; 00542 printf("Printing all filenames:\r\n"); 00543 while((de = readdir (dir)) != NULL) { 00544 printf(" %s\r\n", &(de->d_name)[0]); 00545 } 00546 00547 printf("Closeing root directory. "); 00548 error = closedir(dir); 00549 return_error(error); 00550 printf("Filesystem Demo complete.\r\n"); 00551 00552 // Blink led with 2 Hz 00553 while(true) { 00554 led = !led; 00555 wait (0.5); 00556 } 00557 } 00558 */
Generated on Tue Jul 12 2022 18:19:35 by
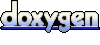